Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial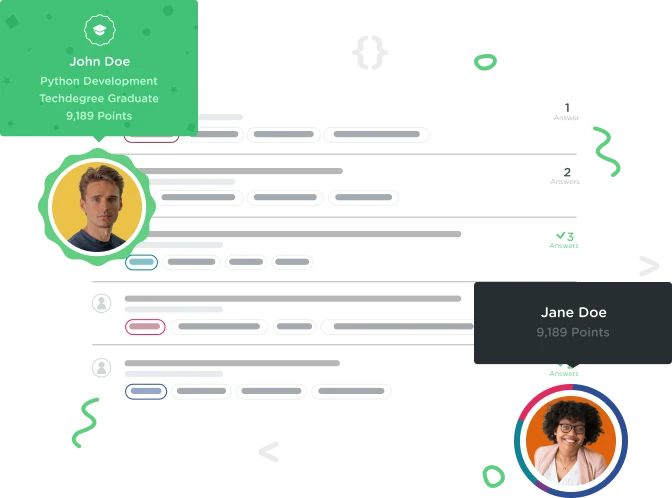

Alex Lee
408 PointsC+ Basic final task STUCK
Hi I could solve this task at all, if someone could help me and points out what I did wrong it would really be appreciated, if possible please provide a solution. Thanks so much.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
try {
string input = Console.ReadLine();
int total = int.Parse(input);
int count =0;
if (total<0)
{
Console.WriteLine("You must enter a positive number.");
return;
}
while (total>count)
Console.WriteLine("\"Yay!\"");
counter++;
}
catch (FormatException) {
Console.Write("You must enter a whole number.");
}
}
}
}
4 Answers
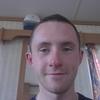
Ronan Parkinson
4,804 PointsIt may be may be the counter++ in your while loop. Should it not be count++ ?
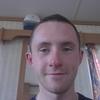
Ronan Parkinson
4,804 Pointsusing System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
try {
string input = Console.ReadLine();
int total = int.Parse(input);
int count =0;
Console.WriteLine("Enter the number of times to print \"Yay!\": ");
if (total<0)
{
Console.WriteLine("You must enter a positive number.");
return;
}
while (total>count){
Console.WriteLine("\"Yay!\"");
count++;
}
}
catch (FormatException) {
Console.Write("You must enter a whole number.");
}
}
}
}
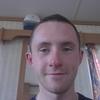
Ronan Parkinson
4,804 PointsThis works. The catch should not be inside to try. The while loop was missing a curly brace after the parentheses. When asking for a number, you had console.write but you must have console.writeline. (sorry about the solution post it didn't come out right).

Mikkel Madsen
4,229 PointsSorry to say but there is no need for the total. As the count already did what we needed. and as this just need to be compared so we don't get a negative number we just need to make sure the the number the user inputs is higher than 0. So it easier just to compare the count against the 0. and if the count is less than 0 you should write out the Console.WriteLine();
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
try
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string input = Console.ReadLine();
int count = int.Parse(input);
if(count>0)
{
int i = 0;
while(i < count)
{
i += 1;
Console.WriteLine("Yay!");
}
}
else
{
Console.WriteLine("You must enter a positive number.");
}
}
catch(FormatException)
{
Console.WriteLine("You must enter a whole number.");
}
}
}
}