Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial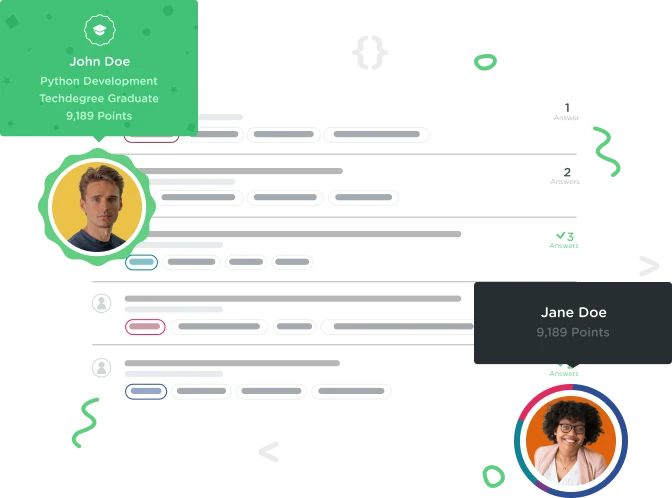
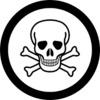
John Weber
6,273 PointsC# Basics: Bonus exercises (Calculator)
I am getting a specific error over and over again on a C# Bonus Exercise (Programming a Calculator). I am brand new to the language and would appreciate any help I could get.
The program is intended to take two numbers from a user, an operator, and then combine the numbers with the operator.
using System;
namespace Treehouse.bonus
{
class Program
{
static void Main()
{
while(true) {
Console.WriteLine("Enter a number: ");
var number1 = Console.ReadLine();
Console.WriteLine("Enter another number: ");
var number2 = Console.ReadLine();
Console.WriteLine("Enter an operator (+, -, *, /, or %): ");
var sign = Console.ReadLine();
try
{
Double number3 = Double.Parse(number1);
Double number4 = Double.Parse(number2);
if(sign == "+")
{
Console.WriteLine(number1 + " + " + number2 + " = " (number3 + number4));
}
else if(sign == "-")
{
Console.WriteLine(number1 + " - " + number2 + " = " (number3 - number4));
}
else if(sign == "*")
{
Console.WriteLine(number1 + " * " + number2 + " = " (number3 * number4));
}
else if(sign == "/")
{
Console.WriteLine(number1 + " / " + number2 + " = " (number3 / number4));
}
else
{
Console.WriteLine("The operator you selected isn't valid... Please try again.");
continue;
}
}
catch(FormatException)
{
Console.WriteLine("One or more of numbers you inputed is invalid. Please try again.");
continue;
}
}
}
}
}
The error message I am getting is this:
quiz.cs(31,59): error CS0119: Expression denotes a value', where a
method group' was expected
quiz.cs(36,59): error CS0119: Expression denotes a value', where a
method group' was expected
quiz.cs(41,59): error CS0119: Expression denotes a value', where a
method group' was expected
quiz.cs(46,59): error CS0119: Expression denotes a value', where a
method group' was expected
... in regards to the else/if operator lines of code. If I simply use an '=' as opposed to '==', I get a different set of errors... What am I missing?
Thank you in advance for your help!!!
2 Answers

srikarvedantam
8,369 PointsWell, you seem to be missing concatenation operator before printing the result. For instance,
Console.WriteLine(number1 + " + " + number2 + " = " (number3 + number4));
in the above statement, after " = ", you can drop in a '+' so that string concatenation can happen. So, it should be as below:
Console.WriteLine(number1 + " + " + number2 + " = " + (number3 + number4));
You may do this for the lines where errors are reported (i.e. 31, 36, 41, 46).

srikarvedantam
8,369 PointsYou are welcome, John:
John Weber
6,273 PointsJohn Weber
6,273 PointsThank you so much for answering both my questions, Srikar. I am just learning to program and sometimes it is really hard to catch simple mistakes without another set of eyes. You just made my night!!!