Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial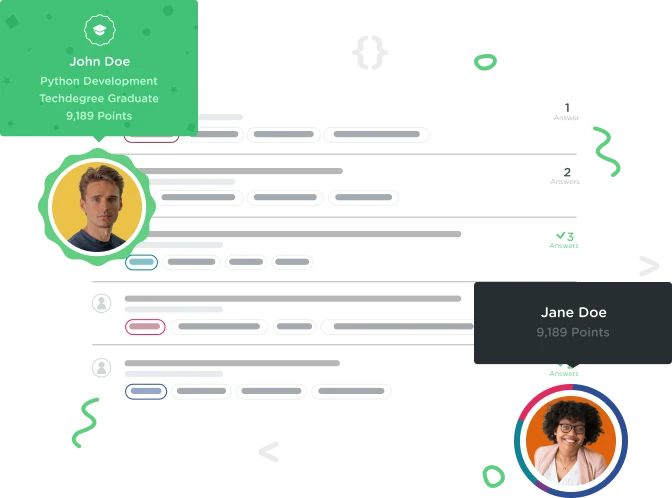

Tristan Johnson
Courses Plus Student 3,022 PointsC# Basics if/else if/else Compile Error
Getting a compile error when trying to compile on this lesson:
(1,12): error CS1525: Unexpected symbol .', expecting
,', ;', or
='
Here is the code:
using System;
namespace Treehouse.FitnessFrog
{
class Program
{
static void Main()
{
int runningTotal = 0;
bool keepGoing = true;
while (keepGoing)
{
// Prompt the user for minutes exercised
Console.Write("Enter how many minutes you exercised or type \"quit\" to exit: ");
string entry = Console.ReadLine();
if (entry == "quit")
{
keepGoing = false;
}
else
{
int minutes = int.Parse(entry);
if (minutes <= 10)
{
Console.WriteLine("Better than nothing, am I right?");
}
else if (minutes <= 30)
{
Console.WriteLine("Way to go hot stuff!");
}
else if (minutes <= 60)
{
Console.WriteLine("You must be a ninja warrior in training!");
}
else
{
Console.WriteLine("Okay, now you're showing off!");
}
}
runningTotal = runningTotal + minutes;
// Add minutes exercised to total
// Display total minutes exercised to the screen
Console.WriteLine("You've entered " + runningTotal + " minutes");
// Repeat until user quits
}
Console.WriteLine ("Goodbye");
}
} }
For the life of me, I don't see the error. Any help is much appreciated. Thanks!
1 Answer
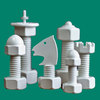
Steven Parker
231,269 PointsAfter reconstructing the program parts that were outside of the blockquote, it appears that the only problem was the closing brace of the else was one line out of place. See the NOTE comment added near the bottom:
using System;
namespace Treehouse.FitnessFrog {
class Program {
static void Main() {
int runningTotal = 0; bool keepGoing = true;
while (keepGoing)
{
// Prompt the user for minutes exercised
Console.Write("Enter how many minutes you exercised or type \"quit\" to exit: ");
string entry = Console.ReadLine();
if (entry == "quit")
{
keepGoing = false;
}
else
{
int minutes = int.Parse(entry);
if (minutes <= 10)
{
Console.WriteLine("Better than nothing, am I right?");
}
else if (minutes <= 30)
{
Console.WriteLine("Way to go hot stuff!");
}
else if (minutes <= 60)
{
Console.WriteLine("You must be a ninja warrior in training!");
}
else
{
Console.WriteLine("Okay, now you're showing off!");
}
// Add minutes exercised to total
runningTotal = runningTotal + minutes;
} // <-- NOTE: this line was above the running total
// Display total minutes exercised to the screen
Console.WriteLine("You've entered " + runningTotal + " minutes");
// Repeat until user quits
}
Console.WriteLine ("Goodbye");
}
}
}