Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial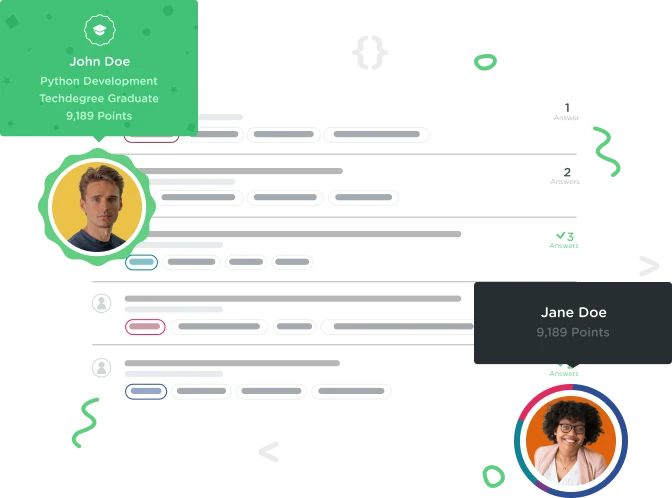
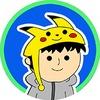
smatt
2,115 PointsC# Basics Interactive quiz task best practice?
Interactive Quiz Write an interactive quiz. It should ask the user three multiple-choice or true/false questions about something. It must keep track of how many they get wrong, and print out a "score" at the end.
So, I have done this task, my code runs and operates (other than validation which I haven't added in yet).
I was wondering if someone would mind seeing what could be done better in this?
// Apologies, copying and pasting from workspaces to questions is adding extra whitespace in the Markdown.
using System;
namespace Quiz
{
class Program
{
static void Main()
{
var correctAnswer1 = "B";
var userAnswer1 = "D";
var correctAnswer2 = "A";
var userAnswer2 = "D";
var correctAnswer3 = "C";
var userAnswer3 = "D";
var correctTotal = 0;
var entry = "unassigned";
while (true)
{
Console.WriteLine("Welcome to the quiz! You will now be asked 3 multiple choice questions!");
Console.WriteLine("Are you ready to start?");
entry = Console.ReadLine();
if (entry.ToLower() == "no")
{
break;
}
else if (entry.ToLower() == "yes")
{
Console.WriteLine("Lets Begin!");
break;
}
}
while (entry.ToLower() == "yes")
{
Console.WriteLine("Please input all answers in letter format. i.e \"A\", \"B\" and \"C\"");
// Question 1
Console.WriteLine("Question 1: 2 + 2 = ?");
Console.WriteLine("A: 6 | B: 4 | C: 8");
userAnswer1 = Console.ReadLine();
if (userAnswer1.ToLower() == "quit")
{
break;
}
else if (userAnswer1.ToUpper() == correctAnswer1)
{
correctTotal++;
Console.WriteLine("Correct!");
}
else if (userAnswer1.ToUpper() != correctAnswer1)
{
Console.WriteLine("Incorrect. Next Question.");
}
// Question 2
Console.WriteLine("Question 2: Grass is which colour?");
Console.WriteLine("A: Green | B: Blue | C: Red");
userAnswer2 = Console.ReadLine();
if (userAnswer2.ToLower() == "quit")
{
break;
}
else if (userAnswer2.ToUpper() == correctAnswer2)
{
correctTotal++;
Console.WriteLine("Correct!");
}
else if (userAnswer2.ToUpper() != correctAnswer2)
{
Console.WriteLine("Incorrect. Next Question.");
}
// Question 3
Console.WriteLine("Question 3: The sky is which colour");
Console.WriteLine("A: Cyan | B: Purple | C: Blue");
userAnswer3 = Console.ReadLine();
if (userAnswer3.ToLower() == "quit")
{
break;
}
else if (userAnswer3.ToUpper() == correctAnswer3)
{
correctTotal++;
Console.WriteLine("Correct! You have reached the end of the quiz!");
}
else if (userAnswer3.ToUpper() != correctAnswer3)
{
Console.WriteLine("Incorrect. You have finished the quiz!");
}
Console.WriteLine("Congratulations on finishing the quiz. Youre total score is " + correctTotal + "/3.");
break;
}
Console.WriteLine("Thanks For Playing, Goodbye!");
}
}
}
1 Answer
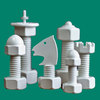
Steven Parker
231,269 PointsYou seem to already have a very solid program, good job! From here, the improvements would be in making the code more compact (or adding features). Ways to condense the code:
- eliminate repeated code with a generic "ask question / get answer" function
- store questions and answers in arrays (or one array of complex objects)
- use a loop to iterate through the questions
Some of these techniques may not have been introduced yet, and might be topics in future lessons.