Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial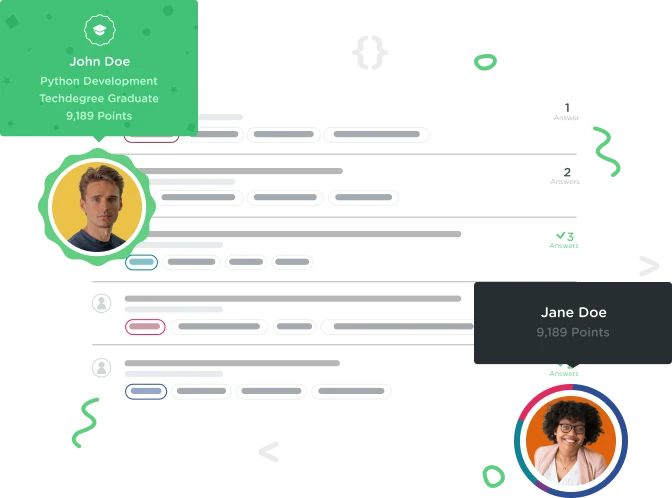
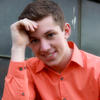
Nathan Stahlman
3,768 PointsC# beginner Final Challenge
I have tried a hundred ways to make this work, but I have no Ideas. I cannot implement the try/catch correctly. Any ideas?
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
bool going = true;
int number = 0;
while(going)
{
try
{
Console.Write("Enter the number of times to print \"Yay!\": ");
var typedIn = Console.ReadLine();
if(typedIn == null)
{
continue;
}
var times = Int32.Parse(typedIn);
number = times;
}
catch (FormatException)
{
Console.Write("You must enter a whole number.");
continue;
}
going = false;
}
for (int i = 0; i < number; i++)
{
Console.Write("Yay!");
if (i == number)
{
going = false;
}
}
}
}
}
3 Answers
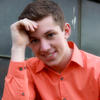
Nathan Stahlman
3,768 PointsHey guys, It was being picky. I didn't have my while loop in the try section. Here it is. using System;
namespace Treehouse.CodeChallenges { class Program { static void Main() { Console.Write("Enter the number of times to print \"Yay!\": ");
int times = 0;
int counter = 0;
string typedIn;
bool going = true;
typedIn = Console.ReadLine();
try
{
times = int.Parse(typedIn);
while(counter < times)
{
Console.Write("Yay!");
counter += 1;
}
}
catch(FormatException)
{
Console.Write("You must enter a whole number.");
}
}
}
}
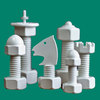
Steven Parker
231,269 PointsYou have an extra loop that prevents the program from ending if bad values are entered..
A program that follows the challenge instructions will only ask for input one time, and either print "Yay!"s or an error message. But either way it should end.

Chris Roberts-Watts
5,434 PointsYeah i understand what your saying, i have been doing C# for a couple of years so have a reasonable understanding of it to know what he is written is okay but if they are after something specific then there we go.
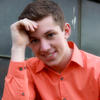
Nathan Stahlman
3,768 PointsI got rid of the extra loop, but it still doesn't work. Where should I go from here?
using System;
namespace Treehouse.CodeChallenges { class Program { static void Main() { Console.Write("Enter the number of times to print \"Yay!\": ");
int times = 0;
int counter = 0;
string typedIn;
bool going = true;
typedIn = Console.ReadLine();
while(going)
{
try
{
times = int.Parse(typedIn);
}
catch(FormatException)
{
Console.Write("You must enter a whole number.");
break;
}
counter += 1;
if (counter == times)
{
going = false;
}
Console.Write("Yay!");
}
}
}
}
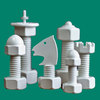
Steven Parker
231,269 PointsTry putting the loop inside the try block instead of vice-versa. Also, you might want to check the loop counter in a way that would allow the program to end before you print anything. What if the number of repeats asked for were 0?

Chris Roberts-Watts
5,434 PointsThe code you have works fine, it does catch correctly. I do however suggest using this Console.ReadLine(); at the end of your program so you can see the Yay!
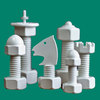
Steven Parker
231,269 Points Using ReadLine a second time would also prevent the program from ending and prevent passing the challenge.

Chris Roberts-Watts
5,434 Pointsdoes it say you cant use the readline in the challenge then as there are no mentions of it in this post?
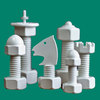
Steven Parker
231,269 PointsThe challenges are looking for specific performance based on the instructions. As I said, "A program that follows the challenge instructions will only ask for input only one time, and either print "Yay!"s or an error message. But either way it should end." Modifying what the program does will prevent passing, even if it might be considered an "improvement".

Chris Roberts-Watts
5,434 PointsFair enough i have not specifically done the code challenge so i wouldnt no exactly what they are asking for but there is nothing fundamentally wrong with what he has written, perhaps if i do the challenge it will be more obvious.
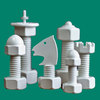
Steven Parker
231,269 PointsI often caution students to be wary when testing challenge code externally. The challenge isn't to just write code that works, but code that performs in a specific way as described in the instructions.