Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial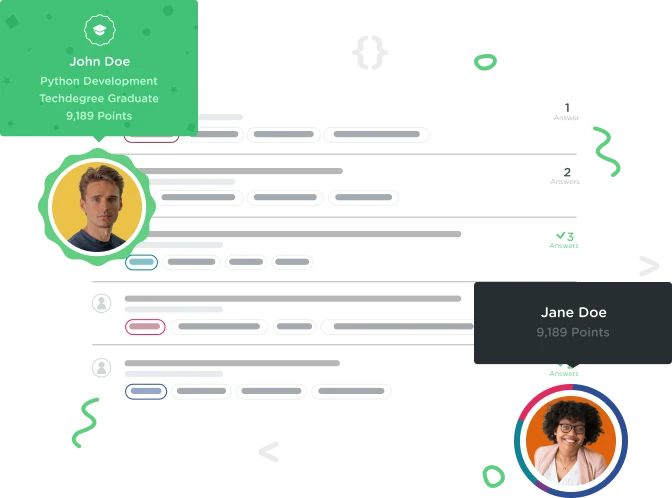

shirshah sahel
10,035 PointsC ++ Branching/ Switch
Need some help with this assignment before the deadline .
Topic
Branching
switch Statement
Description
Enhance the last assignment to include input validation. The enhanced assignment should check the values of day, month, and year entered by the user to ensure that they are OK. If they are not OK, the program should display an error message and return. The program should check the input values before computing the day number.
The program should validate the input values to ensure that:
· Year value is from 2000 to 2099 inclusive.
· Month value is from 1 to 12 inclusive.
· Day value is valid for the given month and the year.
Testing
Input Test Run 1
Enter Month: 4
Enter Day: 31
Enter Year: 2003
Output Test Run 1
Mismatching Month/Day Values Entered.
Month Value Entered: 4
Day Value Entered: 31
Input Test Run 2
Enter Month: 3
Enter Day: 31
Enter Year: 201
Output Test Run 2
Unacceptable Year Entered 201.
Acceptable range For Year is 2000 to 2099
Input Test Run 3
Enter Month: 2
Enter Day: 29
Enter Year: 2003
Output Test Run 3
Mismatching Month/Day Values Entered.
Month Value Entered: 2
Day Value Entered: 29
Input Test Run 4
Enter Month: 2
Enter Day: 29
Enter Year: 2004
Output Test Run 4
Date: 2/29/2004
Day Number: 60
Input Test Run 5
Enter Month: 3
Enter Day: 1
Enter Year: 2003
Output Test Run 5
Date: 3/1/2003
Day Number: 60
Input Test Run 6
Enter Month: 3
Enter Day: 1
Enter Year: 2004
Output Test Run 6
Date: 3/1/2004
Day Number: 61
Sample Code
/*
Java Code
*/
int day, month, year;
//input day, month, and year values here
//Validating Year Value
if ( year < 2000 || year > 2099 )
{
JOptionPane.showMessageDialog
(null, “Unacceptable Year Entered: “ + year );
JOptionPane.showMessageDialog
(null, “Acceptable range For Year is 2000 to 2099 “ );
System.return (0);
}
//Determining Leap year
boolean leapyear = false;
if ((year % 4) == 0)
{
leapyear = true;
}
//Validating Month Value
if ( month < 1 || month > 12 )
{
JOptionPane.showMessageDialog
(null, “Invalid Month Entered: “ + month );
System.return (0);
}
//Validating YearValue
if ( year < 2000 || year > 2099 )
{
JOptionPane.showMessageDialog
(null, “Unacceptable Year Entered: “ + year );
JOptionPane.showMessageDialog
(null, “Valid Range For Year 2000 to 2099 );
System.return (0);
}
//Validating Day Value
if ( ( day < 1 ) || ( day > 31 ) )
{
JOptionPane.showMessageDialog
(null, “Invalid Day Entered: “ + day );
System.return (0);
}
//Validating Other Day Values
if ( ( month == 4 || month == 6 || month == 9 || month == 11) && ( day > 30 ) )
{
JOptionPane.showMessageDialog
(null, “Mismatching Month/Day Values Entered. “ );
JOptionPane.showMessageDialog
(null, “Month Value Entered: “ + month );
JOptionPane.showMessageDialog
(null, “Day Value Entered: “ + day );
System.return (0);
}
if ( (month == 2) && ( day > 29 ) && ( leapyear ) )
{
JOptionPane.showMessageDialog
(null, “Mismatching Month/Day Values Entered: “ );
JOptionPane.showMessageDialog
(null, “Month Value Entered: “ + month );
JOptionPane.showMessageDialog
(null, “Day Value Entered: “ + day );
System.return (0);
}
if ( (month == 2) && ( day > 28 ) && ( ! leapyear ) )
{
JOptionPane.showMessageDialog
(null, “Mismatching Month/Day Values Entered: “ );
JOptionPane.showMessageDialog
(null, “Month Value Entered: “ + month );
JOptionPane.showMessageDialog
(null, “Day Value Entered: “ + day );
System.return (0);
}
/*
C++ Code
*/
/*
The code below asks the user to input month, day and year one after the other. It then validates the values entered. If any of the values is invalid, it displays an error message and returns.
*/
int day, month, year;
//input day, month, and year values here.
//Validate input values
//Determining Leap year
Bool leapyear = false;
if ((year % 4) == 0)
{
leapyear = true;
}
//Validating YearValue
if ( year < 2000 || year > 2099 )
{
cout << “Unacceptable Year Entered: “ << year << endl;
cout << “Valid Range For Year 2000 to 2099 << endl;
return (0);
}
//Validating Month Value
if ( month < 1 || month > 12 )
{
cout << “Invalid Month Entered: “ << month << endl;
return (0);
}
//Validating Day Value
if ( ( day < 1 ) || ( day > 31 ) )
{
cout << “Invalid Day Entered: “ << day << endl;
return (0);
}
//Validating Other Day Values
if ( ( month == 4 || month == 6 || month == 9 || month == 11) && ( day > 30 ) )
{
cout << “Mismatching Month/Day Values Entered. “ << endl;
cout << “Month Value Entered: “ << month << endl;
cout << “Day Value Entered: “ << day << endl;
return (0);
}
if ( (month == 2) && ( day > 29 ) && ( leapyear ) )
{
cout << “Mismatching Month/Day Values Entered: “ << endl;
cout << “Month Value Entered: “ << month << endl;
cout << “Day Value Entered: “ << day << endl;
return (0);
}
if ( (month == 2) && ( day > 28 ) && ( ! leapyear ) )
{
cout << “Mismatching Month/Day Values Entered: “ << endl;
cout << “Month Value Entered: “ << month << endl;
cout << “Day Value Entered: “ << day << endl;
return (0);
}
My code :
#include <iostream>
using namespace std;
int main()
{
int day, month, year;
cout<<"Enter month"<<endl;
cin>> month;
cout<<"Enter day" << endl;
cin>>day;
cout <<"Enter year"<<endl;
cin>> year;
bool leapyear = false;
if ((year % 4) == 0)
{
leapyear = true;
}
if (year<2000 || year > 2099)
{
cout <<"year is valid " <<endl;
}
else
{
cout <<"Unacceptable Year Entered "<< year << endl;
cout <<"Valid Range For Year 2000 to 2099"<< endl;
}
if (month<1 || month> 12)
{
cout <<"month is valid "<<endl;
}
else
{
cout << "Invalid Month Entered "<<month<<endl;
}
// Validating Day Value
if ((day<1) || day>31)
{
cout <<"day is valid "<<endl;
} else
{
cout <<" Invalid Day Entered "<<day<<endl;
}
// Validating Other Day Values
if ((month == 4 || month == 6 || month == 9 || month == 11) && (day >30))
{
cout << "month/Day is valid "<<endl;
}
else
cout << "Mismatching Month/Day Values Entered:"<<endl;
cout <<" Month Value Entered:" << month << endl;
cout <<"Day Value Entered:"<< day << endl;
}
if ( (month == 2) && ( day > 29 ) && ( leapyear ) )
{
cout <<"Month /day values are valid "<<endl;
}else
cout << “Mismatching month/day Values Entered: “ << endl;
cout << “Month Value Entered: “ << month << endl;
cout << “Day Value Entered: “ << endl;
return (0);
}
if ( (month == 2) && ( day > 28 ) && ( ! leapyear ) )
{
cout << "month/day values are valid" <<endl;
}
else
cout << “Mismatching Month/Day Values Entered: “ << endl;
cout << “Month Value Entered: “ << month << endl;
cout << “Day Value Entered: “ << day << endl;
return 0;
}
[MOD: formatted code blocks - srh]
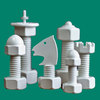
Steven Parker
231,269 PointsI'm also not sure what is being asked here, or why there seems to be a mix of Java and C++ code.
But use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area. Or watch this video on code formatting.
2 Answers

shirshah sahel
10,035 PointsQuestion is on how to use switch statement in c++ language ignore the java code . for example: I have written this code for the program but still don't ask for the input and output that teachers want.
#include<iostream>
using namespace std;
/*
The code below asks the user to input month, day and year one after the other.
It then validates the values entered. If any of the values is invalid,
it displays an error message and returns.
*/
//main function
int main()
{
int day, month, year;
//input day, month, and year values here.
cout<<"Enter Month:";
cin>>month;
cout<<"Enter Day:";
cin>>day;
cout<<"Enter Year:";
cin>>year;
//Declaring leapyear variable
bool leapyear = false;
if ((year % 4) == 0)
{
leapyear = true;
}
//Validating YearValue
if (year < 2000 || year > 2099)
{
cout <<"Unacceptable Year Entered: "<< year << endl;
cout <<"Valid Range For Year 2000 to 2099 "<< endl;
return 0;
}
//Validating Month Value
if (month < 1 || month >12)
{
cout << "Invalid Month Entered: " << month << endl;
return 0;
}
//Validating Day Value
if ((day < 1)||(day >31 ) )
{
cout << "Invalid Day Entered: " << day << endl;
return 0;
}
//Validating Other Day Values
if (( month == 4 || month == 6 || month == 9 || month == 11) && ( day > 30 ) )
{
cout << "Mismatching Month/Day Values Entered. " << endl;
cout << "Month Value Entered: " << month << endl;
cout << "Day Value Entered: " << day << endl;
return 0;
}
if ((month == 2) && ( day > 29 ) && ( leapyear ) )
{
cout << "Mismatching Month/Day Values Entered. " << endl;
cout << "Month Value Entered: " << month << endl;
cout << "Day Value Entered: " << day << endl;
return 0;
}
if ( (month == 2) && ( day > 28 ) && (!leapyear ))
{
cout << "Mismatching Month/Day Values Entered. " << endl;
cout << "Month Value Entered: " << month << endl;
cout << "Day Value Entered: " << day << endl;
return 0;
}
// Determining day number and your day calculation start here
int dayNum;
//Finally If your execution reaches here
switch (month) {
case 1: //Checking for Jan
dayNum = day;
break;
case 2: //For Feb-> Adding Current days and Jan
dayNum = day + 31;
break;
case 3: //March -> adding for current month and for Jan and Feb
dayNum = day + 31 + 28;
break;
case 4: //April
dayNum = day + 31 + 28 + 31;
break;
case 5: //May
dayNum = day + 31 + 28 + 31 + 30;
break;
case 6: //June
dayNum = day + 31 + 28 + 31 + 30 + 31;
break;
case 7: //July
dayNum = day + 31 + 28 + 31 + 30 + 31 + 30;
break;
case 8: //August
dayNum = day + 31 + 28 + 31 + 30 + 31 + 30 + 31;
break;
case 9: //September
dayNum = day + 31 + 28 + 31 + 30 + 31 + 30 + 31 + 31;
break;
case 10: //October
dayNum = day + 31 + 28 + 31 + 30 + 31 + 30 + 31 + 31 + 30;
break;
case 11: //November
dayNum = day + 31 + 28 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + 31;
break;
case 12: //December
dayNum = day + 31 + 28 + 31 + 30 + 31 + 30 + 31 + 31 + 30 + 31 +30;
break;
}
//add 1 for a leap year
if (leapyear==true && month > 2)
{
dayNum ++;
}
//Printing output
cout<<"Data: "<<month<<"/"<<day<<"/"<<year<<endl;
cout<<"Day Number: "<<dayNum;
return 0;
}
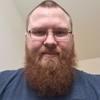
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsA quick search on google gave this example from MSDN :-)
// switch_statement1.cpp
#include <stdio.h>
int main() {
char *buffer = "Any character stream";
int capa, lettera, nota;
char c;
capa = lettera = nota = 0;
while ( c = *buffer++ ) // Walks buffer until NULL
{
switch ( c )
{
case 'A':
capa++;
break;
case 'a':
lettera++;
break;
default:
nota++;
}
}
printf_s( "\nUppercase a: %d\nLowercase a: %d\nTotal: %d\n",
capa, lettera, (capa + lettera + nota) );
}
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsHenrik Christensen
Python Web Development Techdegree Student 38,322 PointsNot sure what the question here is