Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial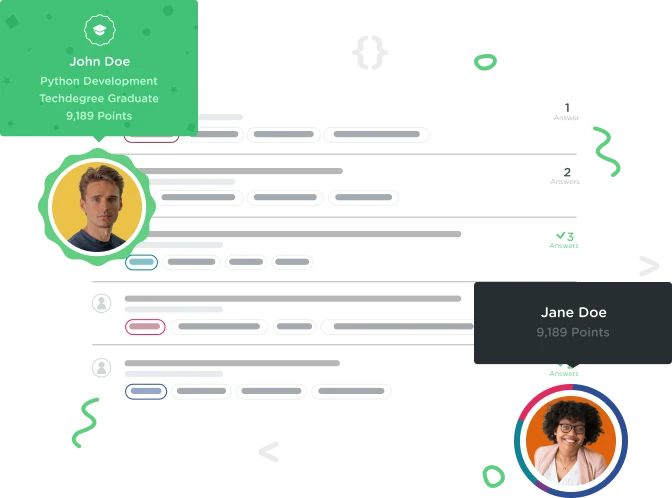

Kevin Maldjian
1,162 PointsC++ Calculating averages
include <string>
#include <iostream>
using namespace std;
float CalcAvgClassGrade( int s1, int s2, int s3, int s4, int s5)
{
float avg,sum;
// Sum grades, and get avg
sum = s1 + s2 + s3 + s4 + s5;
avg = sum / 2;
return avg;
}
string getMax (int s1, int s2, int s3, int s4, int s5, string n1, string n2, string n3, string n4, string n5)
{
float max;
string maxName;
// Compares each grade to find the max
max = s1;
maxName = n1;
if(max < s2)
{max = s2;
maxName = n2;
}
if(max < s3)
{max = s3;
maxName = n3;
}
if (max < s4)
{max = s4;
maxName = n4;
}
if (max < s5)
{max = s5;
maxName = n5;
}
return maxName;
}
float getMin (int s1, int s2, int s3, int s4, int s5, string n1, string n2, string n3, string n4, string n5)
{
float max;
string minName;
// Compares each grade to find the min
min = s1;
minName = n1;
if(min < s2)
{ min = s2;
minName = n2 ;}
if(min < s3)
{ min = s3;
minName = n3}
if (min < s4);
{ min = s4;
minName = n4}
if (min < s5)
{ min = s5;
minName = n5
}
return minName;
}
int main()
{
string student1, student2, student3, student4, student5;
int student1Grade, student2Grade, student3Grade, student4Grade, student5Grade;
float class_avg;
// Enter Student Names;
cout << "Enter Student Name: " ;
cin >> student1;
cout << "Enter Student Grade: " ;
cin >> student1Grade;
cout << "Enter Student Name: " ;
cin >> student2;
cout << "Enter Student Grade: " ;
cin >> student2Grade;
cout << "Enter Student Name: " ;
cin >> student3;
cout << "Enter Student Grade: " ;
cin >> student3Grade;
cout << "Enter Student Name: " ;
cin >> student4;
cout << "Enter Student Grade: " ;
cin >> student4Grade;
cout << "Enter Student Name: " ;
cin >> student5;
cout << "Enter Student Grade: " ;
cin >> student5Grade;
6
// Class AVG
classAvg = f_CalcAvgClassGrade(student1Grade, student2Grade, student3Grade, student4Grade, student5Grade,);
cout << "Class average = "<< classAvg
// MAX GRADE
classMax = s_getMax(student1Grade, student2Grade, student3Grade, student4Grade, student5Grade, student1, student2, student3, student4, student5)
cout << classMax << " has the highest grade in the class"
// Min Grade
classMin = s_Min(student1Grade, student2Grade, student3Grade, student4Grade, student5Grade, )
cout << classMin <<" has the lowest grade in the class"
}
I am attempting to build a program that announces the class average, top student and bottom student. I am getting alot of errors into the compiler. This is my first time ever coding in c++ so I have been having some trouble picking up the syntax. Can anyone find any errors in this?