Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial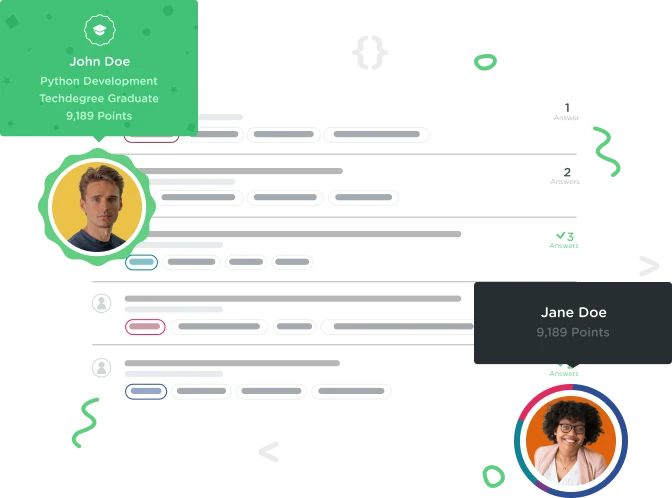

Jenny P
829 PointsC# challenge Frog / EatFly
So I get this error:
Frog.cs(19,21): error CS0111: A member `Treehouse.CodeChallenges.Frog.EatFly(int, int)' is already defined. Rename this member or use different parameter types Frog.cs(14,21): (Location of the symbol related to previous error) Compilation failed: 1 error(s), 0 warnings
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public readonly int ReactionTime;
public Frog(int tongueLength, int reactionTime)
{
TongueLength = tongueLength;
ReactionTime = reactionTime;
}
public bool EatFly(int distanceToFly, int flyReactionTime)
{
return TongueLength >= distanceToFly;
}
public bool EatFly(int distanceToFly, int flyReactionTime){
return TongueLength >= distanceToFly && flyReactionTime <= TongueLength;
}
}
}
4 Answers

Jenny P
829 PointsAccording to the challenge am I supposed to name a second method with the name EatFly. I think they want me to overload methods. I think that is what I am doing?
The challenge says:
"Add another method named EatFly that takes two integer parameters and returns a boolean value. Name the parameters distanceToFly and flyReactionTime. Return true if the frog’s tongue is longer (i.e. greater) or equal to than the distance to the fly and its reaction time is faster (i.e. less) or equal to than the fly’s reaction time."
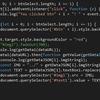
Damien Bactawar
8,549 Pointspublic bool EatFly(int distanceToFly)
{
return TongueLength >= distanceToFly;
}
public bool EatFly(int distanceToFly, int flyReactionTime){
return TongueLength >= distanceToFly && flyReactionTime <= TongueLength;
}
Okay these are the two methods. The first method was already done for you in the challenge but you changed it. Restore this EatFly method which only takes one parameter. Then add the EatFly method which you wrote (and which I pasted here).
This is method overloading because depending on the number and type of arguments that you pass into the method determines which method is called. The first EatFly method takes 1 parameter and the second EatFly method takes 2 parameters.
Also there is a problem with one of the "conditions" that you wrote. I'm sure you can fix it, just read the question carefully and remember you have access to the parameters that were passed in (distanceToFly and flyReactionTime) and the fields (TongueLength and ReactionTime).
Let me know how it goes!

Jenny P
829 PointsYes! Finally :D. Thank you.
2 questions: So overloading wont work if both methods has 2 parameters?
Are TongueLength and ReactionTime reachable in the methods because they are in a constructor?
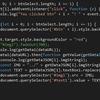
Damien Bactawar
8,549 PointsYou are welcome!
"So overloading wont work if both methods has 2 parameters?" -
If there are two methods with the same name and they both take in two parameters of the SAME type then it wont work. If the types are different then it will.
"Are TongueLength and ReactionTime reachable in the methods because they are in a constructor?" -
TongueLength and ReactionTime are fields which were declared right at the top of the class. They are public so other classes can access them as well as the methods inside this class (Frog.cs). Actually even if the fields were private, the methods would still be able to access the fields because they are in the same class. The constructor is just for when an instance of a Frog object is created.
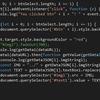
Damien Bactawar
8,549 Points"A member `Treehouse.CodeChallenges.Frog.EatFly(int, int)' is already defined..."
You have created two methods that have the same name and take the same parameters. Either change the name or the parameters of one of the methods. Also you may want to read about "polymorphism".
Jenny P
829 PointsJenny P
829 PointsIf I change the name on the second EatFly method the preview is empty but I get an error from the challenge saying
"Bummer: Did you alter the original EatFly method instead of creating a new one?"