Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial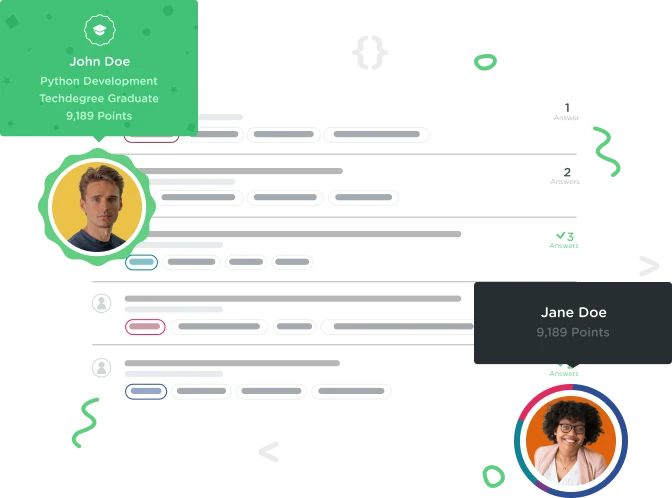
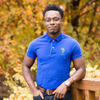
David Murumbi
1,442 PointsC# ending while loop after x number of times
So I am building a project just to test what I've learned so far in c# after completing the basics course. I am trying to ask the user to solve a riddle but they only have 3 tries to solve it then that riddle skips to the next one. I haven't figured out a way to end a while loop after an x amount of times even after doing some research on stack overflow and Microsoft. Please help. Also if you have suggestions to make my code look cleaner you can add that in as well thx!!!
using System;
class MainClass
{
public static void Main (string[] args)
{
//Prompt user to play or quit(done) the game
Console.Write("Welcome to our Quiz!\n");
Console.Write("Start now by typing 'Play' or 'Done' to quit: ");
string start;
start = Console.ReadLine();
if(start.ToLower() == "play")
{
Console.WriteLine("\nAwesome let's start");
}
else
{
Console.Write("Than You! Goodbye");
System.Environment.Exit(0);
}
//Ask first riddle
Console.WriteLine("\nQ1: What has a head, a tail, is brown, and has no legs? ");
while (true)
{
string answerOne;
answerOne = Console.ReadLine();
if (answerOne == "a coin")
{
Console.WriteLine("A1: {0}", answerOne);
Console.WriteLine("YES! You got it!!!");
}
else if (answerOne != "a coin")
{
Console.WriteLine("Nop! Nice try... give it another shot: ");
}
}
//It must keep track of how many they get wrong,
}
}
2 Answers
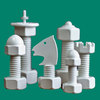
Steven Parker
230,274 PointsYou can have a counter in your loop, and an extra condition for a wrong answer to determine whether to end the loop or give another try. You also don't need to test for the opposite of a previously tested condition, the "else" handles that for you:
int tries = 0; // keep a count of tries
while (true)
{
string answerOne = Console.ReadLine();
if (answerOne == "a coin")
{
Console.WriteLine("A1: {0}", answerOne);
Console.WriteLine("YES! You got it!!!");
break; // end the loop on win
}
else if (++tries < 3) // increment tries and check against limit
{
Console.Write("Nope! Nice try... give it another shot: ");
}
else // too many tries
{
Console.WriteLine("Sorry, you didn't get that one.");
break; // end the loop
}
}
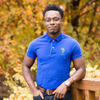
David Murumbi
1,442 PointsThank you so much for your help!
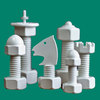
Steven Parker
230,274 PointsDavid Murumbi — Glad to help. You can mark a question solved by choosing a "best answer".
And happy coding!
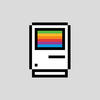
Hakim Rachidi
38,490 PointsA more complete example
If I got you right you want to let the user solve riddles, which are asked randomly, and if the user answers a specific number of question wrong the game ends. The user wins the game when all questions a answered right.
To build such a game you need some kind of game loop (most of the times a while loop is best suited) to
- ask a random question
- get a random number between 0 and the length of questions available
- use the random number to get a random item from a questions store
- get the users input
- compare expected result to the user input
- if they equal delete the question from the store so it won't get asked again and use the
continue
keyword to jump to the next loop cycle - if they don't equal increment a failure counter which gets checked in the while condition
- if they equal delete the question from the store so it won't get asked again and use the
- check that the user hasn't exceeded the failure threshold (lost) and that the user hasn't answered all question (win) via the while condition
var failureCounter = 0; // initialize counter, threshold, and QAs
var failureThreshold = 3;
Dictionary<string, string> qaStore = new Dictionary<string, string>() { // All QAs are stored in a Dictionary
new KeyValuePair()
{
Key = "What has a head, a tail, is brown, and has no legs?", // where the key is the question
Value="a worm" // and the Value is the answer
}
};
var rnd = new Random();
while (failureCounter <= failureThreshold && qaStore.length > 0) // checks for every cycle that the failure count has not exceed the threshold and that there are questions in the Dictionary
{
var randomIndex = rnd.Next(0, qaStore.length); // get a random index for the qaStore (bewteen zero and the qaStores length)
var qa = qaStore.ToList()[randomIndex];
Console.WriteLine(qa.Key.ToString());
var userAnswer = Console.ReadLine();
if(userAnswer.ToLower() == qa.Value) {
Console.WriteLine("Correct!!!!");
qaStore.Remove(qa); // remove the question from the qaStore dictionary so it won't get asked again
continue; // skips the rest in THIS loop cycle but starts a new cycle in this while loop
}
else
{
Console.WriteLine("That was wrong!!");
failureCounter++; // increases the failure count by 1
}
}
if(failureCounter > failureThreshold) {
// if the user has exceed the failureThreshold and the while loop ends
Console.WriteLine("You lost!");
}
else if(qaStore.length < 0) {
// if the user has answered all questions and the while loop ends
Console.WriteLine("You won!");
}
Hope that this is not too complex;
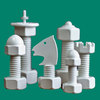
Steven Parker
230,274 PointsIt doesn't look like the question is ever "asked" (shown to the user). And the loop ends when you lose, but what if you win? Wouldn't it just repeat over and over?
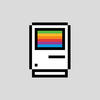
Hakim Rachidi
38,490 PointsIf you add more questions to the question Dictionary and add a random index than it won't.
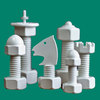
Steven Parker
230,274 PointsI just thought you might want to show a more complete example .
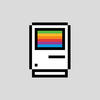
Hakim Rachidi
38,490 PointsThanks. I added the abillity to win the game and upated the answer.
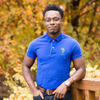
David Murumbi
1,442 PointsHey thank a lot what you wrote is a little advanced for me but will definitely review for the future
David Murumbi
1,442 PointsDavid Murumbi
1,442 PointsI know how to end while loops but it's really just ending after "x" number of times I can't figure out what to do.