Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial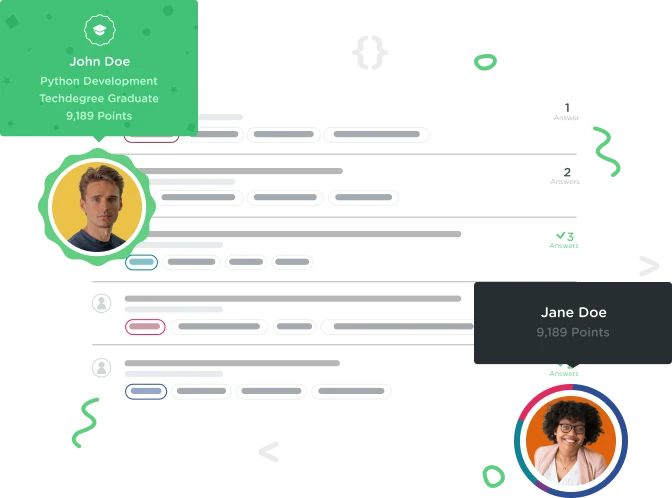

Sean Brannon
596 PointsC# Final Challenge, lost as to what i am doing wrong.
not sure what i am doing wrong on this one, i keep getting the same error and i know where it is but i just dont know how to fix it.
System.ArgumentNullException: Value cannot be null. Parameter name: String
at System.Number.StringToNumber (System.String str, System.Globalization.NumberStyles options, System.Number+NumberBuffer& number, System.Globalization.NumberFormatInfo info, System.Boolean parseDecimal) [0x00054] in /builddir/build/BUILD/mono-4.8.1/mcs/class/referencesource/mscorlib/system/number.cs:1074
at System.Number.ParseInt32 (System.String s, System.Globalization.NumberStyles style, System.Globalization.NumberFormatInfo info) [0x00014] in /builddir/build/BUILD/mono-4.8.1/mcs/class/referencesource/mscorlib/system/number.cs:745
at System.Int32.Parse (System.String s) [0x00000] in /builddir/build/BUILD/mono-4.8.1/mcs/class/referencesource/mscorlib/system/int32.cs:120
at Treehouse.CodeChallenges.Program.Main () [0x00012] in <86cdb0f446c94141914cf2255066b6ab>:0
at MonoTester.Run () [0x00095] in MonoTester.cs:86
at MonoTester.Main (System.String[] args) [0x00013] in MonoTester.cs:28
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
var celebrations = 0;
while (true)
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string entry = Console.ReadLine();
int yays = int.Parse(entry);
celebrations = celebrations + yays;
if (celebrations <=0)
{
try
{
while (celebrations < 5)
{
Console.WriteLine("Yay!");
}
}
catch(FormatException)
{
continue;
}
}
}
}
}
}
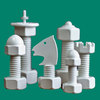
Steven Parker
230,274 PointsI was showing an example of a different way you might construct a loop. The "do something" comment is where the WriteLine would go.
2 Answers
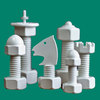
Steven Parker
230,274 PointsIf the input is not valid, you should print out an error message and then end, but the code shown has no error message. And if it is valid, you need to keep a count of the times you go through the loop so you can end. If you don't modify your counter inside the loop, the test will always be true and the loop will never stop.
You also have an extra outer loop that prevents the program from ending. A program conforming to the challenge instructions will prompt and accept input only one time, and will output a number of "Yay!"s or an error message depending on the validity of the input, and then it will end.

Sean Brannon
596 PointsThank you so much, i have fixed my code to a working level but now im lost on how to get the Console.WriteLine to write Yays! times the number the user as entered.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{ var celebrations = 0;
bool repeat = true;
while(repeat)
{
Console.Write("Enter the number of times to print \"Yay!\": ");
string entry = Console.ReadLine();
int yays = int.Parse(entry);
celebrations = celebrations + yays;
if(celebrations >= 5)
{
repeat = false;
}
}
Console.WriteLine("Yay!");
}
}
}
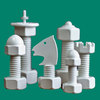
Steven Parker
230,274 PointsSince the prompt and input should only be done once, it should not be in the loop. And while it's possible to manage your own loop control variable, it might be easier to test your repeat condition directly in the loop, something like this:
while (yays > 0) {
// do something...
yays -= 1;
}

Sean Brannon
596 PointsTook me a bit to finally grasp what you wrote, but i got it. as soon as i finally realized i should move input and counts outside of the loop it clicked.
Sean Brannon
596 PointsSean Brannon
596 PointsOk i am still lost on what code i should be using to track the number the user enters and change that into a certain amount of Yay!
this is the code i currently have which only writes 1 Yay!
as for the code Steven Parker wrote, i have no idea how im supposed to implement it. and i am also not sure which function it is (subtraction assignment or Unsubscribe) either of which i dont know how to use.