Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial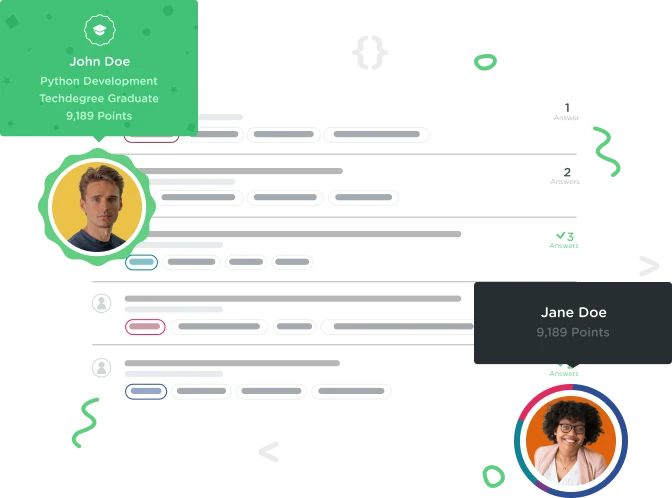
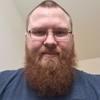
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsC# How to avoid using the same loop-code over and over?
Hi,
I'm making an address book in c# (console app) and so far it's working fine (still a lot to do) and I finally figured how to make it print the information to a .txt file.
I chose to store all the informations typed in in an array and then looping through the array when printing the message (to the console and the .txt file).
My problem is my loop look very identical 2 places and the third place the only difference is the word "SW" is changed to "Console" - I'd love if someone had a great idea how to maybe make a method to use instead of copy/paste the loop :-)
using System;
using System.IO;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace AddressBook
{
class Helpers
{
private static string[] info = new string[5];
private static readonly string _path = @"AddressBook.txt";
public static void Instructions()
{
Console.WriteLine("Type 'new' to add a new person to your 'Address Book'.");
Console.WriteLine("Type 'quit' to exit the program.");
Console.WriteLine("Type 'help' to get the instructions again.");
}
public static void AddNewPerson()
{
Console.Write("First Name: ");
info[0] = "\nFirst name: " + Console.ReadLine();
Console.Write("Last Name: ");
info[1] = "Last name: " + Console.ReadLine();
Console.Write("Address: ");
info[2] = "Address: " + Console.ReadLine();
while (true)
{
Console.Write("Phone Number: ");
try
{
var number = int.Parse(Console.ReadLine());
info[3] = "Phone number: " + number;
break;
}
catch(FormatException)
{
Console.WriteLine("A phone number can only contain numbers");
continue;
}
catch(OverflowException)
{
Console.WriteLine("The number is too long.");
continue;
}
}
Console.Write("Email: ");
info[4] = "Email: " + Console.ReadLine();
for (int i = 0; i < info.Length; i++)
{
Console.WriteLine(info[i]);
}
}
public static void SaveMessage()
{
if (!File.Exists(_path))
{
using (StreamWriter SW = new StreamWriter(_path))
{
for (int i = 0; i < info.Length; i++)
{
SW.WriteLine(info[i]);
}
SW.WriteLine(); // Added to make a blank line before the new person is added to the file
SW.Close();
}
}
else
{
using (StreamWriter SW = File.AppendText(_path))
{
for (int i = 0; i < info.Length; i++)
{
SW.WriteLine(info[i]);
}
SW.WriteLine(); // Added to make a blank line before the new person is added to the file
SW.Close();
}
}
}
}
}
2 Answers
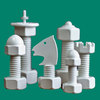
Steven Parker
231,007 Points
This might be a good use of a function or method.
If you find your code doing the same or very similar things over and over, it might be a good opportunity to put the repeated portion of the code into a separate function or method, and then call it from the places where it was previously replicated.
Ideally, any differences between the places the code would be used can be accommodated by arguments passed to the function when it is called.
If you're working with Visual Studio, you can highlight one sample of the repeated code, then right-click and select Refactor -> Extract Method
. It will then ask you to name the new method and it will create it for you (though some adjustments may be needed).
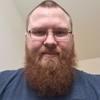
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsAh yes, I forgot about arguments >.<
So far I dont think my code look much like example in "C# objects" course cause for now it's mainly methods, methods and more methods (function) :-/ I might have to watch the course again to learn more about OOP :-P
Thank you very much Steven Parker :-D
Kyle Jensen
Courses Plus Student 6,293 PointsKyle Jensen
Courses Plus Student 6,293 PointsHow is your project going?? Just a thought on formatting. You can format your phone numbers with the following:
myString = String.Format("Some phone number: {0: (###) ### - ####}", 1234567890); //=> (123) 456 - 7890