Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial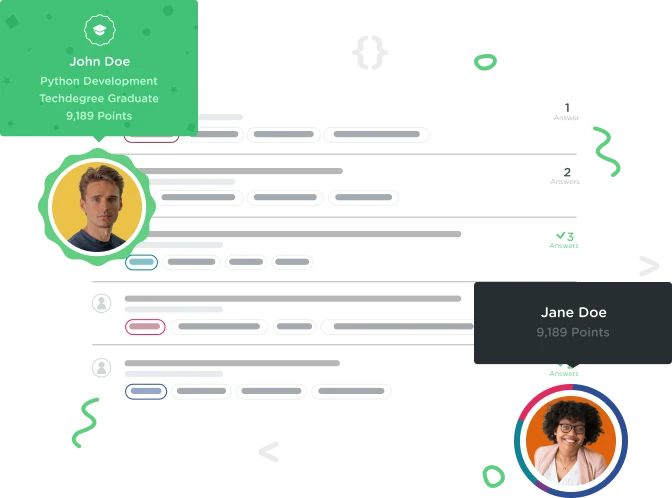

Bruno DaSilva
316 PointsC#: Initializing in base constructor
How do I initialize something in the base constructor?
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength
public Square(int sideLength) : base()
{
SideLength = sideLength;
}
}
}
3 Answers
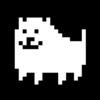
Receipt Box
1,866 PointsHey Bruno, your code that you initially posted will work, you just need to do two things:
1) Like Seth said, you need to write "base(4)", so that NumSides is set to 4 in the Polygon base constructor.
2) You need a semicolon on line 15, or you'll get a compiler error. (You can click "Preview" to see what the error is)
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
public Square(int sideLength) : base(4)
{
SideLength = sideLength;
}
}
}

Bruno DaSilva
316 Pointsoh my god... To think I spent like 30 minutes trying to figure out that little tiny mistake before just giving up... Thats sad haha, thanks..

Seth Kroger
56,413 PointsYou do it by passing arguments through base(), and they need to be the same arguments the base class's constructor takes. Because Polygon's constructor takes the number of sides as an argument, you need to pass that through base().
public Square(int sideLength) : base(4) // A square has 4 sides.
{
SideLength = sideLength;
}

Bruno DaSilva
316 PointsHello I already tried this but for some reason it still doesnt work? If you copy my exact code from above and paste it (with fixing that problem you just pointed out) it still wont work?

Mark Phillips
1,749 PointsI also spent about 30 minutes trying to figure out what I was doing wrong.
turns out despite it being valid syntax I was setting the parameter and field variables the wrong way round.
Not accepted by the code challenge.
public Square(int sideLength) : base(4)
{
sideLength = SideLength;
}
Accepted by the code challenge.
public Square(int sideLength) : base(4)
{
SideLength = sideLength ;
}
Bruno DaSilva
316 PointsBruno DaSilva
316 PointsAlso can you explain why when you answer? Thanks :D