Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial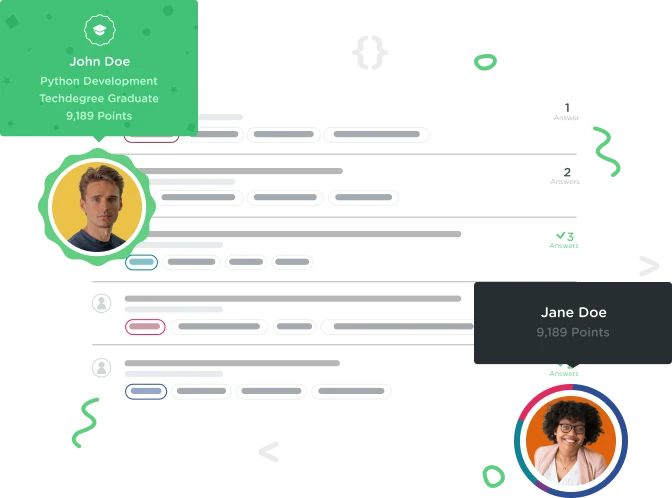

Patrick McLeod
5,913 PointsC# Objects Challenge: Address Book
Hi I should just say it and get it out of the way that I´m an absolute beginner in programming and in spite of my best efforts, I´m mostly stumbling along I´m kinda stumped on an error I´m getting. It´s a CS1061 error in my Program.cs file on the 43rd line where its says: contacts.NewEntry(); I´d really appreciate some help here please. The code is below...
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Threading; using AddressBookChallenge.Helpers;
namespace AddressBookChallenge { class Program { static void Main(string[] args) {
AddressBook[] contacts = new AddressBook[5];
bool keepGoing = true;
string entry;
while (keepGoing == true)
{
Console.WriteLine("Hello, welcome to Address Book");
Console.Write("Please enter your name: ");
string name = Console.ReadLine();
Thread.Sleep(1000);
Console.WriteLine("Let´s begin!");
Thread.Sleep(2000);
Console.Clear();
Menu.Options();
Console.Write("Option: ");
entry = Console.ReadLine();
if (entry.ToLower() == "quit")
{
Console.WriteLine("Goodbye");
Thread.Sleep(2000);
keepGoing = false;
}
if (entry.ToLower() == "add")
{
Console.Clear();
contacts.NewEntry();
}
else if (entry.ToLower() == "clear")
{
Console.Clear();
continue;
}
else if (entry.ToLower() == "search")
{
}
else
{
Console.WriteLine("That is not a valid Option, please try again " + name);
continue;
}
Thread.Sleep(2000);
break;
}
}
}
}
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
namespace AddressBookChallenge.Helpers { class AddressBookEntry { public string FirstName { get; set; } public string LastName { get; set; } public int PhoneNumber { get; set; } public string Address { get; set; } public string Email { get; set; }
public AddressBookEntry()
{
}
public AddressBookEntry(string firstName, string lastName)
{
FirstName = firstName;
LastName = lastName;
}
}
}
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks;
namespace AddressBookChallenge.Helpers { class Menu { public static void Options() { Console.WriteLine("The following is a list of options: \n" + "1. Type \"add\" to add someone in the Address Book. \n" + "2. Type \"clear\" to clear the Console. \n" + "3. Type \"quit\" to close the Address Book. \n" + "4. Type \"search\" to look for soomeone in the Address Book."); } } }
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Threading; using AddressBookChallenge.Helpers;
namespace AddressBookChallenge.Helpers { class AddressBook { public AddressBookEntry[] people; public int Size { get; private set; } private int _totalEntries = 0;
public AddressBook(int size)
{
people = new AddressBookEntry[size];
Size = size;
}
public void NewEntry()
{
Console.Write("New Entry: \n");
AddressBookEntry person = new AddressBookEntry();
Console.Write("Enter as First Name: ");
person.FirstName = Console.ReadLine();
Console.Write("Enter a Last Name: ");
person.LastName = Console.ReadLine();
Console.Write("Enter a Phone Number: ");
person.PhoneNumber = Int32.Parse(Console.ReadLine());
Console.Write("Enter an Address: ");
person.Address = Console.ReadLine();
Console.Write("Enter an Email: ");
person.Email = Console.ReadLine();
Console.WriteLine("Your new contacts details are:");
Console.WriteLine("\n" + person.FirstName + "\n" + person.LastName + "\n" + person.Address + "\n" + person.PhoneNumber + "\n" + person.Email);
Thread.Sleep(5000);
people[_totalEntries++] = person;
}
}
}
1 Answer

Nicko Pekhlivanov
4,451 PointsThe problem with the code is in this line :
AddressBook[] contacts = new AddressBook[5];
It should be changed to :
AddressBook contacts = new AddressBook(5);
The local variable has to be of type AddressBook but it is declared to be an array of AddressBook(s).
The code would be much more readable and maintainable if the numerous if ... else if ... statements are replaced with a switch statement:
switch (entry.ToLower())
{
case "quit":
{
...
}
case "add":
{
...
}
case "clear":
{
...
}
case "search":
{
...
}
default:
{
...
}
}
Steven Parker
231,269 PointsSteven Parker
231,269 PointsA much better way to share your project is to make a snapshot of your workspace and post the link to it here.
That makes it easy to replicate and analyze the issue.