Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial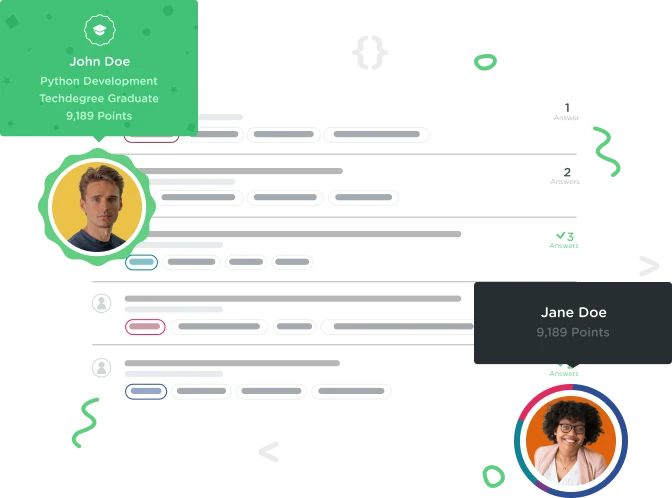

Andrew Fleet
4,305 PointsC# Objects For Loop Challenge
I am struggling to complete this task. Can any body help. My code is attached.
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
double sum = 0;
double average=0;
int count = 0;
for(int i = 0; i < frogs.Length; i++)
{
sum += frogs[i].TongueLength;
if (i == frogs.Length -1)
{
count = i ;
break;
}
}
average =sum/count;
return average;
}
}
}
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
2 Answers

andren
28,558 PointsWhile I can kind of see what your thought process was with your code, it's more complicated than it needs to be, and slightly wrong math wise.
You don't have to keep a count of how many times the loop ran, and you don't need an if statement to break out of the loop. The condition for your if statement is essentially the same condition as your for loop, so the loop would end at the same time even if you completely remove the if statement.
Also to calculate the average of a collection of items you have to divide the sum of the items with the total number of items, in your code you end up dividing the sum by a number 1 less than the total number of items.
The reason why you don't need to keep a count manually is that the number of items to divide by is simply the number of frog objects, which you already have access to through frogs.Length, and as mentioned earlier you don't need to break out of the loop manually.
Here is the solution code with some comments added to clarify what I changed in your code and why:
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
double sum = 0;
double average=0;
// Removed count variable since it is unnecessary
for(int i = 0; i < frogs.Length; i++)
{
sum += frogs[i].TongueLength;
// Removed if statement since the condition i < frogs.Length
// and i == frogs.Length -1 is essentially the same condition.
}
average =sum/frogs.Length;
// Divide the sum by frogs.Length as that contains the total number of frog objects
return average;
}
}
}

Andrew Fleet
4,305 PointsThanks andren
I had slept on it and worked it out