Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial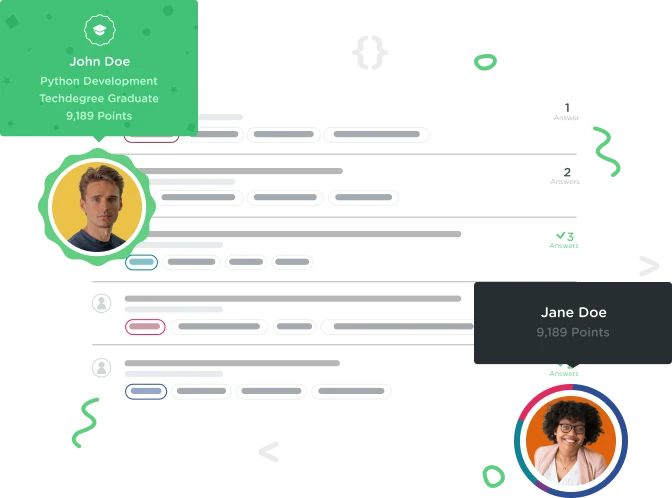
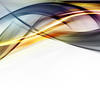
Scott Tucker
3,765 PointsC# Objects for loop challenge task help
This challenge is really bugging me. I don't know if it is how this challenge is worded or if I am really a noob in C# but I need help with this. The error that I am getting says 'not all code paths return a value'. I figured maybe I am suppose to create the array but I did that and it still returns the same error. As always it feels like I am really close to the answer. Any help would be appreciated.
using System;
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
double[] frog = {1.1,2.2,3.3,4.4,5.5};
for(int i = 0; i < frog.Length; i++)
{
Console.WriteLine(frog[i]);
}
}
}
}
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
2 Answers
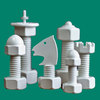
Steven Parker
229,788 PointsThe error message 'not all code paths return a value' generally means that you have defined a function or method that should return a value, but in the code you forgot to actually put a return statement that has a value.
Sure enough, your method GetAverageTongueLength is defined to return a double but it has no return statement.
Also, you seem to have deviated from the challenge instructions quite a bit. Here's a few more hints:
- you won't need to define any static data, the challenge will pass the data to you
- you won't need to print (Console.WriteLine) anything. just calculate and return the result.
- be sure to use the passed-in array argument (frogs) in your calcuations
- remember, you're trying to calculate average length of the tongues of the frogs in the array
I'll bet you can get it now without an explicit spoiler.

Jeremy McLain
Treehouse Guest TeacherIt sounds to me like you've declared the sum
variable as type int
. You can fix this by making it a double so that it matches the type of the things being added up.
Steven Parker's advice will get you the rest of the way. :)
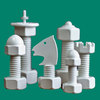
Steven Parker
229,788 PointsI didn't see your comment until Jeremy tagged me. It does sound like you have the right idea. As Jeremy said, be sure you're adding things of the same type. Also be sure to:
- declare the sum variable before the loop (and set it to 0).
- accumulate the sum in the loop
- get the average by dividing after the loop
- be sure to return the average
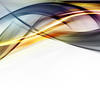
Scott Tucker
3,765 PointsI figured it out. Thank you both for your help. My error was not realizing I can put the TongueLength property onto the frogs array.
Scott Tucker
3,765 PointsScott Tucker
3,765 PointsAm I suppose to return and calculate the average in the for loop or just calculate the average in the for loop? I am pretty sure the average gets calculated like this: 'Sum of data in frogs array' / frogs.Length.
I have tried using '+=' to get the sum of the data in the frogs array by doing this: sum += frogs[i]; but I get an error that says 'Operator += cannot be applied to operands of type int'. I think I use the for loop to get the sum of the array and then divide that sum by frogs.Length. Then return the result of the average calculation. In my head I know what it should do but I can't seem to write it out.