Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial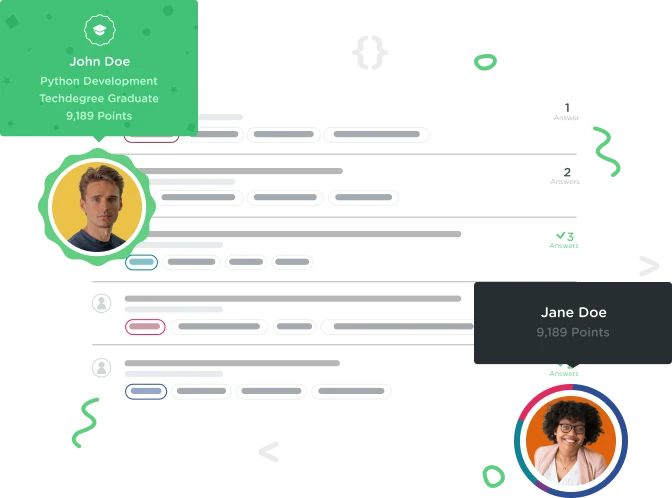

William Lindholm
1,149 PointsC# Objects Method task 3of3 Help
Did you create a method named EatFly that accepts these parameter types: int, int?
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public readonly int ReactionTime;
public readonly int flyReactionTime;
public Frog(int tongueLength, int reactionTime)
{
TongueLength = tongueLength;
ReactionTime = reactionTime;
}
public bool EatFly(int distanceToFly)
{
return (TongueLength >= distanceToFly && ReactionTime <= flyReactionTime);
}
}
}
2 Answers
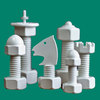
Steven Parker
231,275 PointsThe "flyReactionTime" is not an instance variable. It's a parameter of the new "EatFly" method. Your method body looks OK, but you still need to take the additional parameter.
Also, this should be a new method. The original "EatFly" method (which takes just one argument) should remain as-is.

Ben Reynolds
35,170 Points- You'll need to add a second EatFly method instead of modifying the first one.
- The EatFly overload will take two int parameters (you don't need to declare the new variable as a class field at the top)