Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial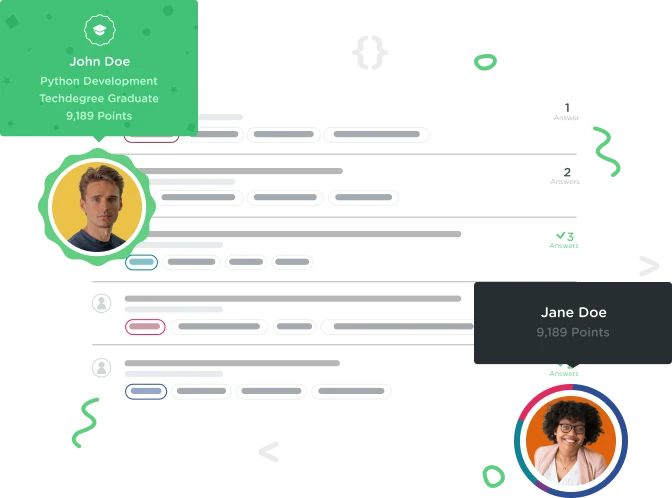
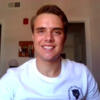
Evan Welch
1,815 PointsC# Public Variables Not Working as Expected
I am coding a battleship game where you can play the computer to better understand what I learned in the C# Objects course.
My program has no errors, yet is not continuing past this point:
Computer computer = new Computer();
computer.ComputerCoordinates();
Ship computerShip = new Ship(computer.ComputerXAxis, computer.ComputerYAxis);
Console.WriteLine("The computer's ship has been created at a secret location.");
Here is the Computer class referenced:
using System;
using System.Collections.Generic;
using System.Text;
namespace BattleShip
{
class Computer
{
public int ComputerXAxis;
public int ComputerYAxis;
public void ComputerCoordinates()
{
Random random = new Random();
ComputerXAxis = random.Next(6); //x coordinate value between 0 and 5
ComputerYAxis = random.Next(6);
}
}
}
I expected for the computer method ComputerCoordinates()
to pick two random integers for the x and y coordinates. Then I will instantiate a ship for the computer using those coordinates. I can instantiate a ship for the player, so instantiating the ship is not the issue.
I think my issue has to do with public variables. I expect that I can assign them from another class or method just as long as they are connected with a namespace and accessible. But this does not seem to be the case. I would really appreciate help in solving this elegantly, more than that if someone could help me think differently about variables and how I can and can't (and shouldn't) assign them.
If I didn't given enough information to help, please say, I'll send more of my code if you think that could help. Thanks!
3 Answers

Simon Coates
8,223 PointsI ran a demo in workspaces with:
using System;
using BattleShip;
class Program
{
static void Main(string[] args)
{
Computer computer = new Computer();
computer.ComputerCoordinates();
Ship computerShip = new Ship(computer.ComputerXAxis, computer.ComputerYAxis);
Console.WriteLine(computer.ComputerXAxis);
Console.WriteLine(computer.ComputerYAxis);
Console.WriteLine("The computer's ship has been created at a secret location!");
}
}
using System;
using System.Collections.Generic;
using System.Text;
namespace BattleShip
{
class Computer
{
public int ComputerXAxis;
public int ComputerYAxis;
public void ComputerCoordinates()
{
Random random = new Random();
ComputerXAxis = random.Next(6); //x coordinate value between 0 and 5
ComputerYAxis = random.Next(6);
}
}
}
namespace BattleShip
{
class Ship{
public Ship(int x, int y)
{
}
}
}
to get:
treehouse:~/workspace$ dotnet run
4
3
The computer's ship has been created at a secret location!
Unless I've modified something without paying attention, it seems like your code works. The fields of computer are populated and accessible.
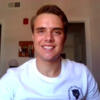
Evan Welch
1,815 PointsThank you for doing that Simon Coates. I was so convinced it must be this code which was not working because I wouldn't see "the computer's battleship was created in a secret location" and my code would just stop even though there was much more for the Main method to do.
I feel bad that there wasn't any errors. There is also a second if and else statement right where my code will go back to the top of the method and say welcome to BattleShip
for the second time and repeat the method that has already run. Is there some reason I can't have two if statements that are very similar like:
if (twoPlayerOrOne.IsTwoPlayer())
{
player1.Player1XAxis = coordinateInput.GetCoordinate(map.dimensions, "player 1", "X-axis", "player 2");
player1.Player1YAxis = coordinateInput.GetCoordinate(map.dimensions, "player 1", "Y-axis", "player 2");
player2.Player2XAxis = coordinateInput.GetCoordinate(map.dimensions, "player 2", "X-axis", "player 1");
player2.Player2YAxis = coordinateInput.GetCoordinate(map.dimensions, "player 2", "Y-axis", "player 1");
}
else
{
player1.Player1XAxis = coordinateInput.GetCoordinateVsComputer(map.dimensions, "player 1", "X-axis");
player1.Player1YAxis = coordinateInput.GetCoordinateVsComputer(map.dimensions, "player 1", "Y-axis");
}
Ship player1Ship = new Ship(player1.Player1XAxis, player1.Player1YAxis);
Console.WriteLine("Player1's ship has been created a secret location.");
if (twoPlayerOrOne.IsTwoPlayer())
{
Ship player2Ship = new Ship(player2.Player2XAxis, player2.Player2YAxis);
Console.WriteLine("Player2's ship has been created at a secret location.");
}
else
{
Computer computer = new Computer();
computer.ComputerCoordinates();
Ship computerShip = new Ship(computer.ComputerXAxis, computer.ComputerYAxis);
Console.WriteLine("The computer's ship has been created at a secret location.");
}
Or is this a circumstance where I need to learn troubleshooting. Thank you for your continued help Simon Coates. I'm dealing with a big time learning curve. This is more or less my first time programming.

Simon Coates
8,223 PointsIn honesty, I'm not sure what you mean about the program stopping. If there's something syntactically wrong with it, you'd typically get a compile error. But if the thing runs and then stops and doesn't produce some kind of error message or abnormal behavior, that sounds weird to me. if it's running at all, a primitive form of debugging would be to whack a bunch of writelines that allow you to verify the program flow. A more advanced way to debug a program that runs would be to use an IDE and set breakpoints. Then you can step though and examine what it's doing (flow, variable values) and verifying your assumptions.
Running similar conditions shouldn't be a problem for the computer, I don't think. Depending on what you're doing, it might be a problem if you were repeating code that could be broken out into a method or if the level of repetition created a very long method that might be difficult to think about (some people are very stringent about method length).
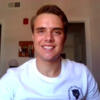
Evan Welch
1,815 PointsOk! I was actually able to find the problem using a breakpoint! Thank you!