Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial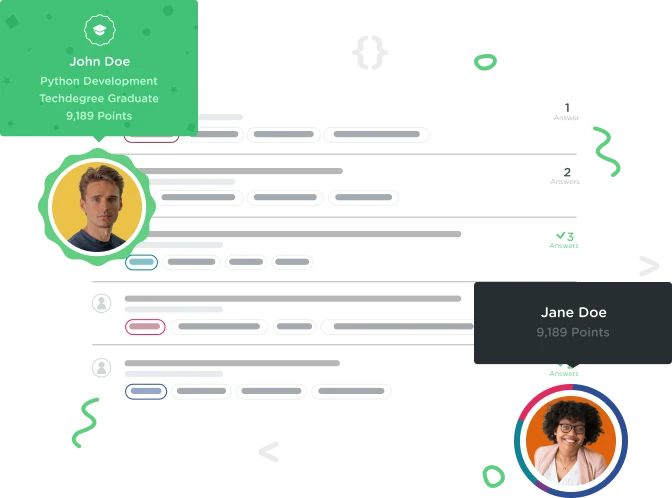

Larry Singleton
18,946 PointsC# Radio Buttons
Link: https://teamtreehouse.com/library/aspnet-mvc-forms/improving-our-form/radio-buttons
Question
In Report.cshtml add two more calls to the Html.RadioButtonFor HTML helper method to render the radio buttons for the "Major" and "Critical" SeverityLevel enumeration values.
Given Code
@model IssueReporter.Models.Issue
@using IssueReporter.Models
@{
ViewBag.Title = "Report an Issue";
}
<h2>@ViewBag.Title</h2>
@using (Html.BeginForm())
{
<div>
@Html.LabelFor(m => m.Name)
@Html.TextBoxFor(m => m.Name)
</div>
<div>
@Html.LabelFor(m => m.Email)
@Html.TextBoxFor(m => m.Email)
</div>
<div>
@Html.LabelFor(m => m.DepartmentId)
@Html.DropDownListFor(m => m.DepartmentId, (SelectList)ViewBag.DepartmentsSelectListItems)
</div>
<div>
@Html.LabelFor(m => m.Severity)
@Html.RadioButtonFor(m => m.Severity,
Issue.SeverityLevel.Minor) @Issue.SeverityLevel.Minor
</div>
<div>
@Html.LabelFor(m => m.Reproducible)
@Html.TextBoxFor(m => m.Reproducible)
</div>
<div>
@Html.LabelFor(m => m.DescriptionOfProblem)
@Html.TextAreaFor(m => m.DescriptionOfProblem)
</div>
<button type="submit">Save</button>
}
My Answer
@model IssueReporter.Models.Issue
@using IssueReporter.Models
@using IssueReporter.Entry.SeverityLevel
@{
ViewBag.Title = "Report an Issue";
}
<h2>@ViewBag.Title</h2>
@using (Html.BeginForm())
{
<div>
@Html.LabelFor(m => m.Name)
@Html.TextBoxFor(m => m.Name)
</div>
<div>
@Html.LabelFor(m => m.Email)
@Html.TextBoxFor(m => m.Email)
</div>
<div>
@Html.LabelFor(m => m.DepartmentId)
@Html.DropDownListFor(m => m.DepartmentId, (SelectList)ViewBag.DepartmentsSelectListItems)
</div>
<div>
@Html.LabelFor(m => m.Severity)
<div>
@Html.RadioButtonFor(m => m.Severity,
Entry.SeverityLevel.Major) @Entry.SeverityLevel.Major
@Html.RadioButtonFor(m => m.Severity,
Entry.SeverityLevel.Critical) @Entry.SeverityLevel.Critical
</div>
</div>
<div>
@Html.LabelFor(m => m.Reproducible)
@Html.TextBoxFor(m => m.Reproducible)
</div>
<div>
@Html.LabelFor(m => m.DescriptionOfProblem)
@Html.TextAreaFor(m => m.DescriptionOfProblem)
</div>
<button type="submit">Save</button>
}
`` Error: Bummer! Did you remove any of the provided code?
Not sure what I'm doing wrong...
```Report.cshtml
@model IssueReporter.Models.Issue
@using IssueReporter.Models
@{
ViewBag.Title = "Report an Issue";
}
<h2>@ViewBag.Title</h2>
@using (Html.BeginForm())
{
<div>
@Html.LabelFor(m => m.Name)
@Html.TextBoxFor(m => m.Name)
</div>
<div>
@Html.LabelFor(m => m.Email)
@Html.TextBoxFor(m => m.Email)
</div>
<div>
@Html.LabelFor(m => m.DepartmentId)
@Html.DropDownListFor(m => m.DepartmentId, (SelectList)ViewBag.DepartmentsSelectListItems)
</div>
<div>
@Html.LabelFor(m => m.Severity)
@Html.RadioButtonFor(m => m.Severity,
Issue.SeverityLevel.Minor) @Issue.SeverityLevel.Minor
</div>
<div>
@Html.LabelFor(m => m.Reproducible)
@Html.TextBoxFor(m => m.Reproducible)
</div>
<div>
@Html.LabelFor(m => m.DescriptionOfProblem)
@Html.TextAreaFor(m => m.DescriptionOfProblem)
</div>
<button type="submit">Save</button>
}
2 Answers

Jeanette Brown
20,485 PointsYou might of just been overthinking it.
<div>
@Html.LabelFor(m => m.Severity)
@Html.RadioButtonFor(m => m.Severity,
Issue.SeverityLevel.Minor) @Issue.SeverityLevel.Minor
@Html.RadioButtonFor(m => m.Severity,
Issue.SeverityLevel.Major) @Issue.SeverityLevel.Major
@Html.RadioButtonFor(m => m.Severity,
Issue.SeverityLevel.Critical) @Issue.SeverityLevel.Critical
</div>
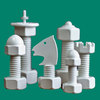
Steven Parker
231,275 PointsDo only what the instructions ask.
The challenge says, "add two more calls to the Html.RadioButtonFor
HTML helper method...". So do only that to pass the challenge. In particular:
- do not add additional
using
statement(s) - do not add additional HTML elements (such as
<div>
) - do not remove the original radiobutton for the "Minor" level