Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial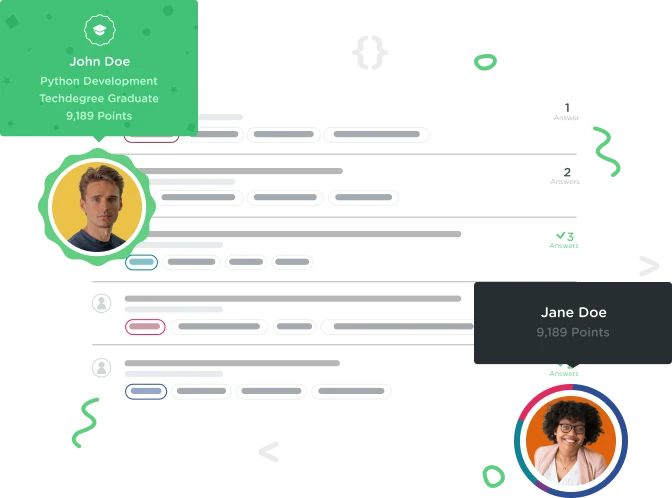
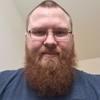
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsC# Way of the concept of OOP?
Hi,
I finished the C# Objects course few days ago and now I'm working on the Address Book assignment the teacher gives in the last video to practice the OOP concept.
Now I have the feeling that I'm not doing it right and would love if someone would tell me if I'm doing it right or if I'm missing something.
So far the program ask for informations and then save it in a .txt file, but I don't feel like creating a Search the file function right now if I'm doing it "wrong" :-P
Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace AddressBook
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Welcome to your personal 'Address Book'.");
Console.WriteLine("\nHere is a quick instruction how to use the 'Address Book':");
Information.Instructions();
while (true)
{
Console.Write("\nPlease enter your instruction: ");
var input = Console.ReadLine();
if(input.ToLower() == "quit")
{
break;
}
else if(input.ToLower() == "help")
{
Information.Instructions();
}
else if(input.ToLower() == "new")
{
Console.Clear();
Information.AddNewPerson();
while (true)
{
Console.Write("Do you want to save this? (yes/no) ");
var saveIt = Console.ReadLine();
if (saveIt.ToLower() == "no")
{
Console.Clear();
Information.Instructions();
break;
}
else if (saveIt.ToLower() == "yes")
{
// Save the information in a text file
SaveInfo.PrintMessage();
Console.Clear();
Console.WriteLine("The information is saved.\n");
Information.Instructions();
break;
}
else
{
Console.WriteLine("Please answer 'yes' or 'no'.");
continue;
}
}
}
else
{
Console.WriteLine("That was not a valid instruction (type 'help' to get the list of instructions)");
}
}
}
}
}
Information.cs
using System;
using System.IO;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace AddressBook
{
class Information
{
public static string[] info = new string[5];
public static readonly string _path = @"AddressBook.txt";
public static void Instructions()
{
Console.WriteLine("Type 'new' to add a new person to your 'Address Book'.");
Console.WriteLine("Type 'quit' to exit the program.");
Console.WriteLine("Type 'help' to get the instructions again.");
}
public static void AddNewPerson()
{
Console.Write("First Name: ");
info[0] = "\nFirst name: " + Console.ReadLine();
Console.Write("Last Name: ");
info[1] = "Last name: " + Console.ReadLine();
Console.Write("Address: ");
info[2] = "Address: " + Console.ReadLine();
while (true)
{
Console.Write("Phone Number: ");
try
{
var number = int.Parse(Console.ReadLine());
info[3] = "Phone number: " + number;
break;
}
catch(FormatException)
{
Console.WriteLine("A phone number can only contain numbers");
continue;
}
catch(OverflowException)
{
Console.WriteLine("The number is too long.");
continue;
}
}
Console.Write("Email: ");
info[4] = "Email: " + Console.ReadLine();
for (int i = 0; i < info.Length; i++)
{
Console.WriteLine(info[i]);
}
}
}
}
SaveInfo.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace AddressBook
{
class SaveInfo
{
private static void LoopInfo(StreamWriter SW)
{
for (int i = 0; i < Information.info.Length; i++)
{
SW.WriteLine(Information.info[i]);
}
SW.WriteLine(); // Added to make a blank line before the new person is added to the file
SW.Close();
}
public static void PrintMessage()
{
if (!File.Exists(Information._path))
{
using (StreamWriter SW = new StreamWriter(Information._path))
{
LoopInfo(SW);
}
}
else
{
using (StreamWriter SW = File.AppendText(Information._path))
{
LoopInfo(SW);
}
}
}
}
}
3 Answers

James Churchill
Treehouse TeacherHenrik,
This is looking great so far! Nice job digging in and making it work :)
For more of an OOP approach, what if you were to replace the array of strings (that you're using to store the address information in) with an "Address" class? Give that a try and see where that leads you next.
Thanks ~James

James Churchill
Treehouse TeacherHenrik,
How about an example of using class properties instead of an array to store values?
// This is a shortened version of your current Person class.
class Person
{
// 0 = First Name
// 1 = Last Name
// 2 = Address
// 3 = Phone Number
// 4 = Email
public string[] Info;
public Person()
{
Info = new string[5];
}
}
// This is a modified version of your Person class that replaces the array of strings with properties.
class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
...
}
I added the first two properties to get you started. Once you finish adding the other properties, your other code will break. You'll need to go through your application and update it to use your new properties instead of the array of strings.
Thanks ~James
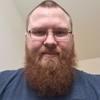
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsHi James,
That might be a better approach. I will give it a try and see if I can make it work! :-)
Thank you very much! :-D
Henrik Christensen
Python Web Development Techdegree Student 38,322 PointsHenrik Christensen
Python Web Development Techdegree Student 38,322 PointsHi James,
Thank you very much for your input :-)
I tried to re-write the program few hours after I made this post and then I got stucked. When I got stucked I tried to make another program (which I might have to re-write too at some time) since I noticed, when I finished the first version, that I keep doing the same things as I always do (just using methods, methods and more methods).
I think I might have to re-watch the C# Objects course again and try understand some of the things that I didn't get the first time :-)
Anyway, the "updated" version of the AddressBook project I posted above can be found at https://github.com/henrikac/AddressBook :-)