Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial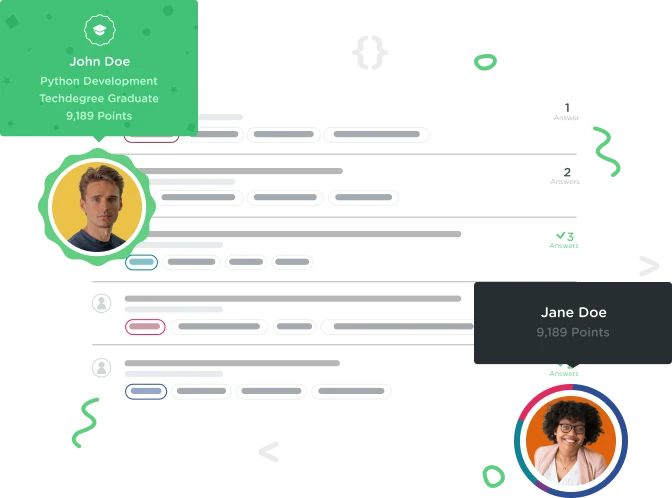
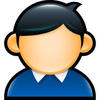
Daniel Hildreth
16,170 PointsC# Working With Enums
I don't know what I'm doing wrong here. I seem to have it set up as what the videos showed us. Can someone help me out please? This is the error I'm getting:
Program.cs(20,46): error CS0103: The name `condition' does not exist in the current context
Program.cs(20,22): error CS0453: The type `' must be a non-nullable value type in order to use it as type parameter `TEnum' in the generic type or method `System.Enum.TryParse(string, out TEnum)'
Program.cs(22,53): error CS0103: The name `condition' does not exist in the current context
Compilation failed: 3 error(s), 0 warnings
using System;
using System.IO;
namespace Treehouse.CodeChallenges
{
public class Program
{
public static void Main(string[] arg)
{
}
public static WeatherForecast ParseWeatherForecast(string[] values)
{
var weatherForecast = new WeatherForecast();
DateTime timeOfDay;
if (DateTime.TryParse(values[1], out timeOfDay))
{
weatherForecast.TimeOfDay = timeOfDay;
}
if (Enum.TryParse(values[2], out condition))
{
weatherForecast.Condition = condition;
}
return weatherForecast;
}
}
}
using System;
/* Sample CSV Data
weather_station_id,time_of_day,condition,temperature,precipitation_chance,precipitation_amount
HGKL8Q,06/11/2016 0:00,Rain,53,0.3,0.03
HGKL8Q,06/11/2016 6:00,Cloudy,56,0.08,0.01
HGKL8Q,06/11/2016 12:00,PartlyCloudy,70,0,0
HGKL8Q,06/11/2016 18:00,Sunny,76,0,0
HGKL8Q,06/11/2016 19:00,Clear,74,0,0
*/
namespace Treehouse.CodeChallenges
{
public class WeatherForecast
{
public string WeatherStationId { get; set; }
public DateTime TimeOfDay { get; set; }
public Condition Condition { get; set; }
}
public enum Condition
{
Rain,
Cloudy,
PartlyCloudy,
PartlySunny,
Sunny,
Clear
}
}
2 Answers

Chris Atchley
18,468 PointsYou need to create your Condition variable before you can use it.
Condition condition;
if (Enum.TryParse(values[2], out condition))
{
weatherForecast.Condition = condition;
}
```

Sara Rena Anderson
15,045 Pointsusing System;
using System.IO;
namespace Treehouse.CodeChallenges
{
public class Program
{
public static void Main(string[] arg)
{
}
public static WeatherForecast ParseWeatherForecast(string[] values)
{
var weatherForecast = new WeatherForecast();
DateTime timeOfDay;
if (DateTime.TryParse(values[1], out timeOfDay))
{
weatherForecast.TimeOfDay = timeOfDay;
}
Condition condition;
if (Enum.TryParse(values[2], out condition))
{
weatherForecast.Condition = condition;
}
return weatherForecast;
}
}
}
Daniel Hildreth
16,170 PointsDaniel Hildreth
16,170 PointsThanks Chris Atchley. I think I may have had that part of the code, otherwise I would not have passed the second part of this code challenge. However, when I went on to the third challenge it did not save that bit of information in the code.