Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial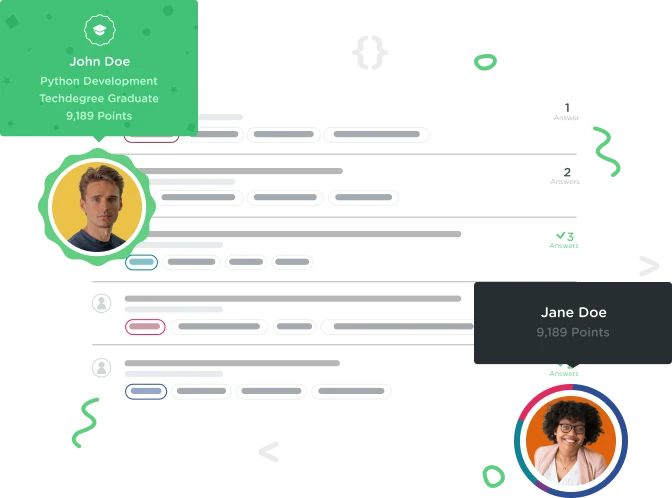

Charlie Harcourt
8,046 PointsCalculator - bonus work
Comilation failed: 5 error(s), 0 warnings
Why is it not working?
URL to code: https://w.trhou.se/hh7wu1g8x4
3 Answers
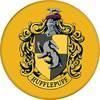
Izabela Matos
6,302 Points1) You forgot to parse a few integers through your code
2) on line 16 you wrote
if(num1.ToLower() == "quit")
but int variables don't accept .ToLower() methods. the same mistake was made on line 36
3) on line 22 you wrote
num1 = double.Parse(userAnswer)
but userAnswer doesn't seem to exist anywhere else on your code
4) in this part of your code, you should take out the "int" in front of your runningTotal variables, cause you have already declared it on the 1st line of the program
if (op == '+')
{
int runningTotal = num1 + num2;
}
else if (op == "-")
{
int runningTotal = num1 - num2;
}
else if (op == "/")
{
int runningTotal = num1 / num2;
}
else if (op == "*")
{
int runningTotal = num1 * num2;
these are the ones I was able to catch ^~^

Charlie Harcourt
8,046 PointsSteven Parker woud you be able to add anything on? Please :)
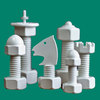
Steven Parker
230,274 Points Hi, I got alerted by your tag.
It looks like you might have prior experience with a language like JavaScript or Python. But in C#, variables have a specific type that cannot be changed. So you'll need to keep your strings/ints/doubles/etc. separate.
For example:
string ans1 = Console.ReadLine(); // need a string here
double num1; // reserve a double for later
if (ans1 == "quit") // compare the string
{
break;
}
else
{
num1 = double.Parse(ans1); // convert to a double
}
You'll also need to do something similar for the second number.

Charlie Harcourt
8,046 PointsOk, so when I run this I get far less mistakes, however i'm getting errors like "Operator == cannot be applied to operands of type method group and string" I don't quite understand what this means?
using System; namespace Calculator { class Arithmatic { static void Main() { var runningTotal = 0.0; while(true) {
//Prompt User for a number
Console.WriteLine("Please type a number");
var ans1 = Console.ReadLine();
double num1;
if(ans1.ToLower == "quit")
{
break;
}
else
{
num1 = double.Parse(num1);
}
//Prompt the User for an operation
Console.WriteLine("Type in a operation + - / *");
var ans2 = Console.ReadLine();
if(ans2.ToLower == "quit")
{
break;
}
//Prompt the user for another number
Console.WriteLine("Please type a number");
var ans3 = Console.ReadLine();
double num2;
if(num2 == "quit")
{
break;
}
else
{
num2 = double.Parse(num2);
}
//Prefprm the operation
if(ans2 == "+")
{
runningTotal = num1 + num2;
}
else if(ans2 == "-")
{
runningTotal = num1 - num2;
}
else if(ans2 == "/")
{
runningTotal = num1 / num2;
}
else if(ans2 == "*")
{
runningTotal = num1 * num2;
}
Console.WriteLine("Your number is " + runningTotal + ".");
}
Console.WriteLine("Bye");
}
}
}
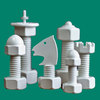
Steven Parker
230,274 PointsThat's another case where different types are being compared. You're seeing "method group" as the type when you name the method (using no parentheses) instead of call it (using parentheses) like on line 18:
// if (ans1.ToLower == "quit") <-- name alone is a "method group"
if (ans1.ToLower() == "quit") // parentheses after name CALL the method
And there are still several cases where you need to separate strings from doubles or ints, like on line 25:
// num1 = double.Parse(num1); <-- can't convert from a double (or itself)
num1 = double.Parse(ans1); // but convert from a string
There are a few more issues like that needing fixing on other lines of the file.
Charlie Harcourt
8,046 PointsCharlie Harcourt
8,046 PointsYes helped thanks :D still getting plenty of errors though.. hmm D: http://w.trhou.se/sn871ual7g