Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial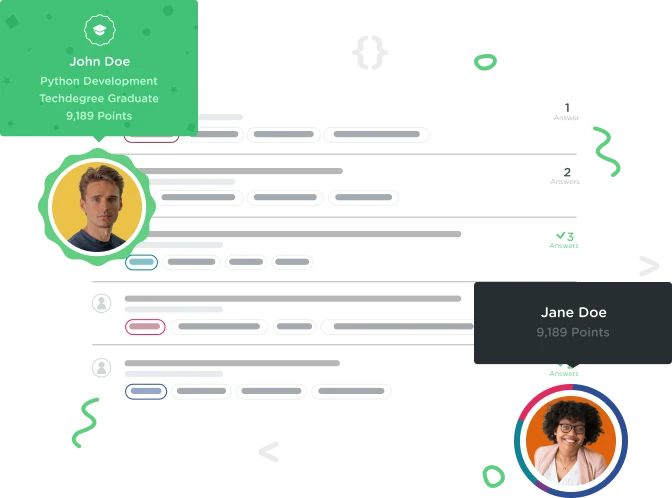
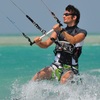
cabrinha98
10,616 PointsCalender Slider
I´m currently programming an Calender and had the idea to design it like a slider.
Something like: http://wisestartupblog.com/wp-content/uploads/2008/02/itunes-cover-flow1.png
The selected day should be centered on the viewport and should show below later on different events.
I´ve created a flex-box and created for each day a rounded div-container and set the parent container to overflow for hiding the non relevant days.
HTML with JavaScript:
<!DOCTYPE html>
<html>
<head>
<title><%= title %></title>
<link rel='stylesheet' href='/stylesheets/style.css' />
<script src="/javascripts/jquery-1.11.3.min.js"></script>
</head>
<body>
<section id="top_container">
<div id="date_rotation">
</div>
</section>
<nav id="menu_bar">
</nav>
<section id="event_container">
jj
</section>
<script>
// Variables
var date = new Date();
var daynames = ["Sonntag","Montag","Dienstag","Mittwoch","Donnerstag","Freitag","Samstag"]
var monthnames = ["Januar","Februar","März","April","Mai","Juni","Juli","August","September","Oktober",
"November","Dezember"];
var calStart = new Date(2015, 4, 28);
var selectedYear = calStart.getFullYear();
var selectedMonth = calStart.getMonth();
var selectedDay = calStart.getDate();
var calLength = 2000;
//Functions
function daysofMonths(myyear,mymonth) {
var monthStart = new Date(myyear, mymonth, 1);
var monthEnd = new Date(myyear, mymonth + 1, 1);
var monthLength = (monthEnd - monthStart) / (1000 * 60 * 60 * 24);
return monthLength;
}
// Creating Calender
for (var i=0; i <= calLength; i++) {
currentDate = new Date(selectedYear, selectedMonth, selectedDay);
if (selectedDay > daysofMonths(selectedYear,selectedMonth)) {
selectedDay = 1;
selectedMonth ++;
if (selectedMonth > 11) {
selectedMonth = 0;
selectedYear ++;
}
$("#date_rotation").append('<div class="date_picker" id="' + selectedDay + selectedMonth + selectedYear + '"><p>' + selectedDay + monthnames[selectedMonth] + '<br>' + daynames[currentDate.getDay()] + ' ' + selectedYear + '</p></div>');
console.log("First");
} else {
$("#date_rotation").append('<div class="date_picker" id="' + selectedDay + selectedMonth + selectedYear + '"><p>' + selectedDay + monthnames[selectedMonth] + '<br>' + daynames[currentDate.getDay()] + ' ' + selectedYear + '</p></div>');
selectedDay ++;
console.log("Secound");
}
}
// Rotate Calender
// Get Position relative to Container
$(".date_picker").click(function( event ) {
var thisPos = $(this).position();
var parentPos = $(this).parent().position();
var x = thisPos.left - parentPos.left;
var y = thisPos.top - parentPos.top;
var width = $("#date_rotation").width();
$("#menu_bar").text(x + "px, " + y + "px," + width + "px");
</script>
</body>
</html>
SCSS
@import "compass/css3";
// Variables
$overlay: rgba(0,0,0,0.75);
$light_overlay: rgba(0,0,0,0.50);
//Layout
#top_container {
position: fixed;
z-index: 100;
top: 0;
left: 0;
right: 0;
width: 100%;
height: 300px;
background-color: $overlay;
border-bottom: 2px solid black;
}
#event_container {
margin: 0 auto 0;
height: 100%;
width: 70%;
background-color: $light_overlay;
}
#menu_bar {
width: 70%;
margin: 302px auto 0;
height: 50px;
background-color: $overlay;
color: white;
}
#date_rotation {
@include display-flex(flex);
@include flex-direction(row);
position: fixed;
top: 75px;
z-index: 101;
.date_picker {
display: inline-block;
position: relative;
overflow: hidden;
margin: 0 25px;
width: 150px;
height: 150px;
background-color: white;
border-radius: 50%;
text-align: center;
font-size: 1em;
p {
line-height:50px
}
}
.date_picker:hover {
cursor: pointer;
transform: scale(1.5);
background-color: $light_overlay;
color: white;
}
}
What I´m trying to archieve is to center the clicked div with the class date_picker and therefore moving the complete parent overflowed container.
I´m totally stuck and dont know how to exactly move the parent-container till the selected child-item is centered perfectly =/ Id love to have an animated solution or hints how I can archieve my goal.
edit: Would be also nice to know if there is a possibility to change the css related to the position. So the selected day in the center of the window should be highlighted with transform: scale(1.5).
Would really appreciate some hints/answers =)
Best regards Cab
1 Answer

Daniel Newman
Courses Plus Student 10,715 PointsIt would be great if you provide us with CSS without mixins and so on. You have a problem inside script tags JS too.
When you add a new one element inside DOM and execute 'handler' on it there is a time when element doesn't exist and jQuery can't attach it. There is some other construction for such situation. Look below and try to find out, how "jquery event on appended element" works.
$(document).on('click', '.someClass', function(){});
I hope that is what you are looking for. BTW, check your code with some tools. There is the problem with brackets on it. {{{ }}}
Edited code: http://codepen.io/newmandani/pen/PqWwMe