Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial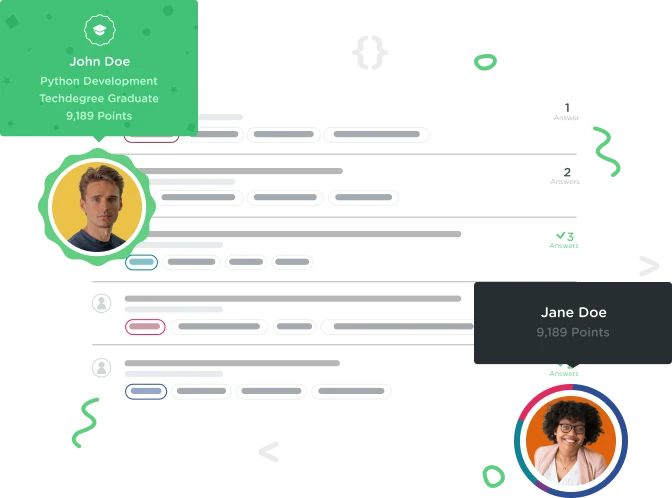

Muzhi Ou
4,921 Pointscall back code challenge
//Why doesn't it work
import Foundation
// Add your code below
func fetchTreehouseBlogPosts(completion: ((NSData!, NSURLResponse!, NSError!) -> Void)){}
// Step 2 fully completed looks like this
let blogURL = NSURL(string: "http://blog.teamtreehouse.com/api/") let requestURL = NSURL(string: "get_recent_summary/?count=20", relativeToURL: blogURL)
let request = NSURLRequest(URL: requestURL!)
let config = NSURLSessionConfiguration.defaultSessionConfiguration() let session = NSURLSession(configuration: config)
// Add your code between the comments let dataTask: NSURLSessionDataTask = session.dataTaskWithRequest(request, completionHandler : { (data : NSData!, reponse : NSURLResponse!, error : NSError!) -> Void in return fetchTreehouseBlogPosts(data,response,error)
}) // Add code above
dataTask.resume()
import Foundation
// Add your code below
func fetchTreehouseBlogPosts(completion: ((NSData!, NSURLResponse!, NSError!) -> Void)){}
// Step 2 fully completed looks like this
let blogURL = NSURL(string: "http://blog.teamtreehouse.com/api/")
let requestURL = NSURL(string: "get_recent_summary/?count=20", relativeToURL: blogURL)
let request = NSURLRequest(URL: requestURL!)
let config = NSURLSessionConfiguration.defaultSessionConfiguration()
let session = NSURLSession(configuration: config)
// Add your code between the comments
let dataTask: NSURLSessionDataTask = session.dataTaskWithRequest(request, completionHandler : {
(data : NSData!, reponse : NSURLResponse!, error : NSError!) -> Void in
return fetchTreehouseBlogPosts(data,response,error)
})
// Add code above
dataTask.resume()
1 Answer
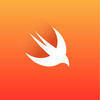
Steven Deutsch
21,046 PointsHey Muzhi Ou,
You're close! This was a difficult one for me. We have to make some changes to your completion handler. First off, when the last argument in a function is a closure, we can implement it as a trailing closure. It's much cleaner to write it this way, so I will use a trailing closure in my example below.
import Foundation
// Add your code below
typealias BlogPostCompletion = ((NSData!, NSURLResponse!, NSError!) -> Void)
func fetchTreehouseBlogPosts(completion: BlogPostCompletion) {
let blogURL = NSURL(string: "http://blog.teamtreehouse.com/api/")
let requestURL = NSURL(string: "get_recent_summary/?count=20", relativeToURL: blogURL)
let request = NSURLRequest(URL: requestURL!)
let config = NSURLSessionConfiguration.defaultSessionConfiguration()
let session = NSURLSession(configuration: config)
// Add your code between the comments
let dataTask: NSURLSessionDataTask = session.dataTaskWithRequest(request) {
(let data, let response, let error) in
completion(data, response, error)
}
dataTask.resume()
}
// Add code above
/* The changes I made were:
1. I used a trailing closure, writing the closure outside the parentheses
2. You don't need to specify the parameter types, just the names
3. You call the completion handler with the completion parameter name,
not the fetchTreehouseBlogPost function
4. Your fetchTreehouseBlogPost function and your completion handler return void,
so no return statement is needed
5. I used a typealias for the completion handler's function signature
*/
Good Luck!
Muzhi Ou
4,921 PointsMuzhi Ou
4,921 PointsThanks Steven! Great help!!