Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial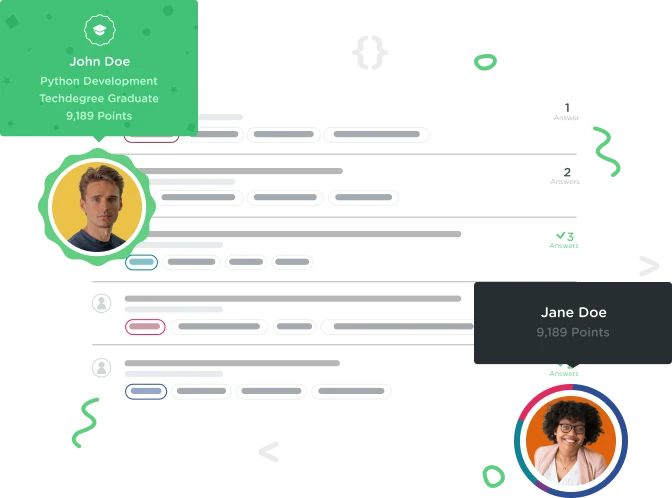

Lisa Conyers
53 PointsCall the printf function on the console object and make it print out "<YOUR NAME> can code in Java!"
Call the printf function on the console object and make it print out "<YOUR NAME> can code in Java!"
// I have setup a java.io.Console object for you named console
2 Answers
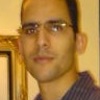
Samuel Mayol
4,786 PointsVery easy, look this
import java.io.Console;
public class PrintYourName {
public static void main(String[] args) {
Console console = System.console();
// Your code goes below here
String firstName = "Sam";
console.printf("Hello, my name is %s\n", firstName);
}
}
You have to import the console input/output using: import java.io.Console; Then create a class and set a name for that class, I used: public class PrintYourName { }, do not forget the { } to create a class block of code. Inside of the { } curly braces goes the body of your class. The body of the class contain the main method: public static void main(String[] args) { }, the main method is run by the JVM, it is where your code start running. In the main method include the body block for this method.
console.printf("Hello, my name is %s\n", firstName);
Create an instance of the Console imported like this: Console console = System.console(); Create a local variable type string: String firstName = "Sam";
This is just a single line comment, it is mandatory: // Your code goes below here
We are using the object created after instantiate our Console class, like this: console.printf("Hello, my name is %s\n", firstName);
console is an instance of Console and it has a method called 'printf' inside of this method we added an argument: "Hello, my name is %s\n", firstName
where "Hello, my name is %s\n" it is going to be display on the screen and the variable value firstName too, however in this case the value of firstName variable will be displayed next to Hello, my name is, because we are using %s which is a format specifier for string values, then \n is a separator that perform a new line, after the " " double quotes goes a comma to separate the variable of the literal. The literal is again "Hello, my name is". The value of the variable is substituted for the %s. The result will be:
Hello, my name is Sam
To see how formatted strings works here I provide a link: [example link]http://www.homeandlearn.co.uk/java/java_formatted_strings.html
I hope to be clear in my example and you can understand my explanation, if you are not so familiar with the term that I used try to google it, because I do not want to do this comment too long.

Kelvin Warui
Courses Plus Student 878 PointsHI i am getting a java.lang.NullPointerException when i run the above code in netbeans IDE,am using netbeans ide
String firstName ="Kelvin";
console.printf("Hello, my name is %s ", firstName);
Why am i getting the error and i have initialized the string variable to "kelvin"
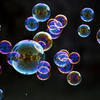
Jialong Zhang
9,821 PointsI used to write my codes in Eclipse. Fortunately, console.printf is similar to System.out.print() method.
String firstName = "Name";
console.printf(firstName + "$s can code in Java!");
Dylan Merritt
15,682 PointsDylan Merritt
15,682 PointsWhat code have you been trying? It should look similar to this:
Good luck.