Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial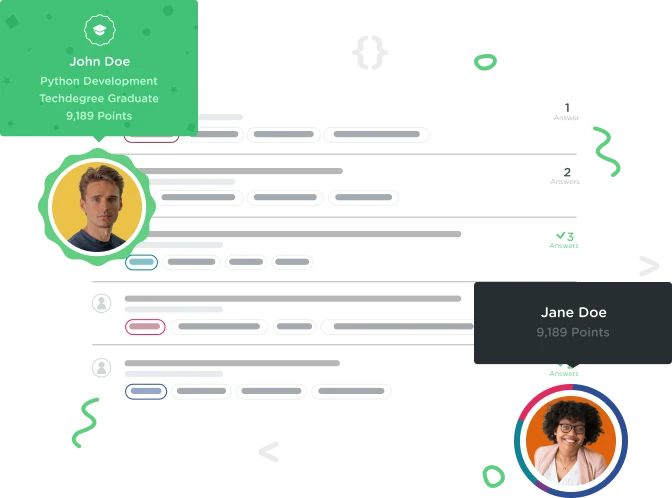

Akshay Rathee
1,205 Pointscall.enqueue error
So guys i was trying to ad call.enqueue method but it says that can't resolve symbol 'enqueue'. what should i do to resolve this? i am using Android Studio 1.2.1.1

Akshay Rathee
1,205 PointsHere it is...
apply plugin: 'com.android.application'
android {
compileSdkVersion 21
buildToolsVersion '20.0.0'
defaultConfig {
applicationId "bollywoodfacts.myweather"
minSdkVersion 16
targetSdkVersion 22
versionCode 1
versionName "1.0"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
}
}
}
dependencies {
compile fileTree(include: ['*.jar'], dir: 'libs')
compile 'com.android.support:appcompat-v7:22.2.0'
compile 'com.squareup.okhttp:mockwebserver:2.4.0'
}

Akshay Rathee
1,205 PointsThanks for responding Harry. But the problem remains. I still see the same error.
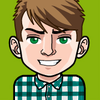
Harry James
14,780 PointsFirst of all, make sure that you have the import statement required for OkHttp:
import com.squareup.okhttp.Call;
// Only the Call import is required right now however, you will also be using the below import statements so you can add them now if you like:
import com.squareup.okhttp.Callback;
import com.squareup.okhttp.OkHttpClient;
import com.squareup.okhttp.Request;
import com.squareup.okhttp.Response;
If you are still having issues, try Cleaning your project. To do this, click on the Build tab and then Clean Project and wait for the Clean to finish:
If even after that you have the error, try Invalidating Android Studio's caches. To do this, click on the File tab then Invalidate Caches / Restart and press Invalidate and Restart:
If after both of these you still get the error, post your new build.gradle file here and the line of code that you're getting the error on.

Akshay Rathee
1,205 PointsHere is my build.gradle
apply plugin: 'com.android.application'
android {
compileSdkVersion 21
buildToolsVersion '20.0.0'
defaultConfig {
applicationId "bollywoodfacts.myweather"
minSdkVersion 16
targetSdkVersion 22
versionCode 1
versionName "1.0"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
}
}
}
dependencies {
compile fileTree(include: ['*.jar'], dir: 'libs')
compile 'com.android.support:appcompat-v7:22.2.0'
compile 'com.squareup.okhttp:okhttp:2.4.0'
}
Here is my mainactivity.java
package bollywoodfacts.myweather;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.util.Log;
import com.squareup.okhttp.OkHttpClient;
import com.squareup.okhttp.Request;
import com.squareup.okhttp.Call;
import java.io.IOException;
public class MainActivity extends ActionBarActivity {
public static final String TAG =MainActivity.class.getSimpleName();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
String apiKey="HIDDEN BY MODERATOR";
double latitude=37.8267;
double longitude= -122.423;
String forecastUrl= "https://api.forecast.io/forecast/"+apiKey+"/"+latitude+","+longitude;
OkHttpClient client= new OkHttpClient();
Request request= new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
call.enqueue------ i am getting error here, it says can't resolve symbol 'enqueue'
{
try {
if (response.isSuccessful()){
Log.v(TAG, response.body().string());
}
} catch (IOException e) {
Log.e(TAG, "Exception Caught;", e);
}
}
}

Akshay Rathee
1,205 Pointsit still isnt working
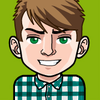
Harry James
14,780 PointsHey Akshay!
It looks as though you've written this code outside of your onCreate() method. Here, it will never get called and so the method won't function.
I've updated the code by moving the end }
for the onCreate() method after the code you've written which should fix the problem for you:
package bollywoodfacts.myweather;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.util.Log;
import com.squareup.okhttp.OkHttpClient;
import com.squareup.okhttp.Request;
import com.squareup.okhttp.Call;
import java.io.IOException;
public class MainActivity extends ActionBarActivity {
public static final String TAG =MainActivity.class.getSimpleName();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
String apiKey="HIDDEN BY MODERATOR";
double latitude=37.8267;
double longitude= -122.423;
String forecastUrl= "https://api.forecast.io/forecast/"+apiKey+"/"+latitude+","+longitude;
OkHttpClient client= new OkHttpClient();
Request request= new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
call.enqueue();
/* Commenting this code out as it currently has no use and would
give you an error
{
try {
if (response.isSuccessful()){
Log.v(TAG, response.body().string());
}
} catch (IOException e) {
Log.e(TAG, "Exception Caught;", e);
}
*/
}
}
}
Hope it helps and if you have any more questions, give me a shout :)

Akshay Rathee
1,205 PointsHarry thank you so much for helping me. I really appreciate it.
1 Answer
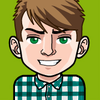
Harry James
14,780 PointsHey again Akshay!
The statement you're using in your build.gradle file is for OkHttp's MockWebServer. Instead, swap the compile line for this:
compile 'com.squareup.okhttp:okhttp:2.4.0'
Then, sync the Gradle files and your error should go away.
Hope it helps and if you have any more problems, give me a shout :)
Harry James
14,780 PointsHarry James
14,780 PointsHey Akshay! Can you please show me your build.gradle file for the app module so that I can take a look at this for you?
Thanks in advance :)