Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial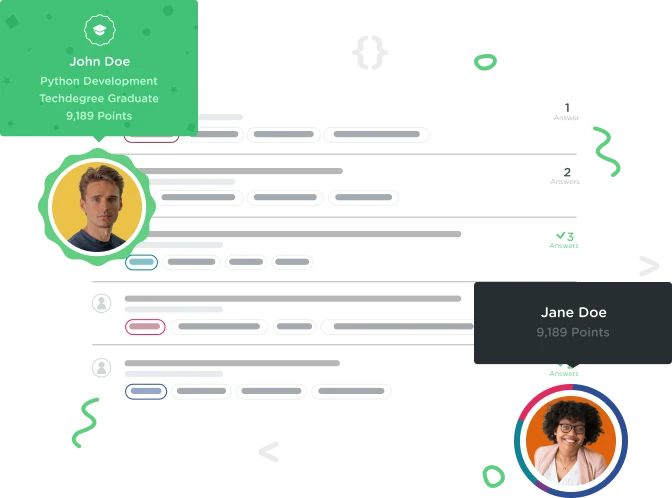
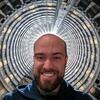
Michael Greig
Front End Web Development Techdegree Student 10,328 PointsCalling a method from within another method - how's it done?
Hi everyone,
I think I may have misunderstood what 'call the normalizeGuess method from the applyGuess method' means.
I've tried placing the entire normaliseGuess method within the applyGuess method but am getting a few error messages, including 'this is not a statement'. Return statement is also unexpected but not sure why - I know the applyGuess method is void but not the normalizeGuess one...
Why is this not working? Do I need to declare it separately first or something...
Any help would be much appreciated!
Michael
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
String normalizeDiscountCode(discountCode){
this.discountCode = discountCode.toUpperCase();
return discountCode;
}
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
2 Answers
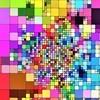
james south
Front End Web Development Techdegree Graduate 33,271 Pointsyou're trying to define a method in another method when you just want to call it. to call you just put the name and (). if it is called with any arguments, they go in the (). myMethod(arg1, arg2...). that's a call that goes in the other method. so in the body of apply, you would have normalizeDiscountCode(discountCode). the return of normalize is then used however you need in apply. the discountCode argument given to apply can be passed into normalize when it is called.
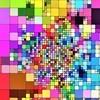
james south
Front End Web Development Techdegree Graduate 33,271 PointsdiscountCode is a field in the Order class. it isn't static, which means it belongs to each instance (each Order) of the class, so you need Order instances to modify it and each instance has its own discountCode. this makes perfect sense, right? each Order in our real lives has however many variations that determine what, if any, discount will apply to that Order. here is what i think is a good run-down of fields and static-ness:
Michael Greig
Front End Web Development Techdegree Student 10,328 PointsMichael Greig
Front End Web Development Techdegree Student 10,328 PointsI think the penny has dropped! Thanks so much for your help.
Yeah I get it now. Calling a method from inside another method only requires the name (I guess that's why it's called 'calling'!) and (), along with any arguments that need to go in there. This means you can define a method separately and then use it as and when you please.
I think I also had the wrong understanding of what this.discountCode actually did but now I can see it relates back to the class field/member variable (is that the right terminology?) at the top.