Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial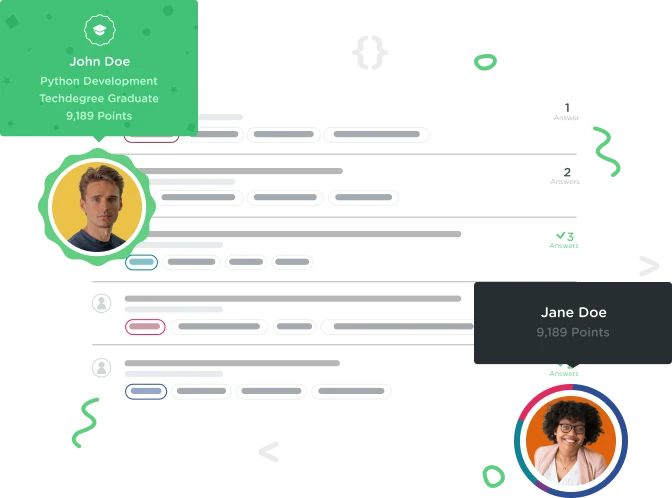

Matt Duarte
2,497 PointsCalling back the function.
What am I doing wrong here? I am overlooking something. function returnValue(hi) { var word = hi; return word; }
var echo = prompt(word + "there"); returnValue("hi, there");
function returnValue(hi) {
var word = hi;
return word;
}
var echo = prompt(word + "there");
returnValue("hi, there");
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers

K Cleveland
21,839 PointsFrom what I can see, the challenge asks you to set the echo variable to the returned string passed in from your returnValue function -- "The function will accept the string you pass to it and return it back (using the return statement). Then your echo variable will be set with what is returned, the results."
In your code, however, you are not setting the echo variable with the returned string from returnValue.
var echo = prompt(word + "there");
returnValue("hi, there");
Try looking at that piece again, and see what you come up with. Good luck!

K Cleveland
21,839 Points if(greaterNumber === numberOne) return numberOne; } else { return numberTwo; }
Check out your syntax on this line.
Also, I just wanted to point out that the way you have written your function, the arguments that you're passing through aren't being used in the function. Take a look at this:
function max(numberOne)
{
var numberOne = 21;
return numberOne
}
max(22)
max(23)
max(24)
All three of those function calls will return 21 instead of the values you passed in. Hope this helps and good luck! :)
Matt Duarte
2,497 PointsMatt Duarte
2,497 PointsThank you, I had figured it out after posting it, but I cannot figure out why my function is not returning the larger number.
function max(numberOne, numberTwo) { var numberOne = 21; var numberTwo = 12; var greaterNumber = numberOne; if(greaterNumber === numberOne) return numberOne; } else { return numberTwo; }
I put it in to the console and it marks else as a syntax error. Why is that? What am I overlooking?