Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial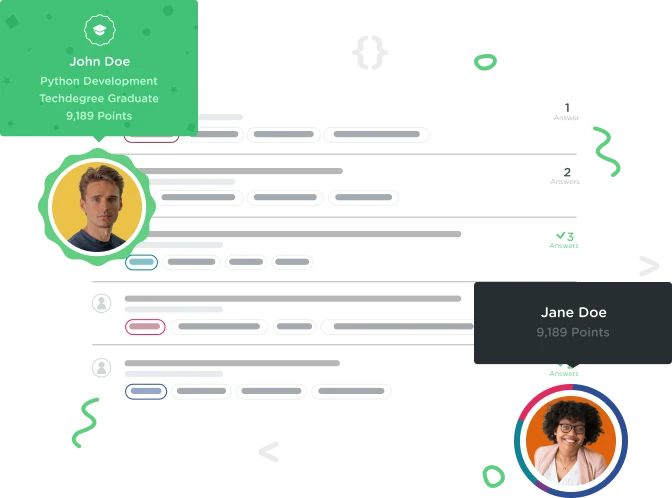

Rob Inman
4,516 PointsCalling External APIs from Express
I recently finished Andrew Chalkley's Express course. For the sake of practice, I'm building on the learnings and am creating some simple, local apps.
One of the apps I'm creating makes a call out to Twitter's APIs. I'm using the twitter library (found https://www.npmjs.com/package/twitter). It's pretty simple to use for what I'm doing.
I have a routes/index.js file that will contain all of my routes. When a simple form is submitted, the variable passed from the form hits Twitter API and streams data on my site.
Is the best practice to have the interaction with Twitter from the route itself, or is it better to have this in a separate file and pass information to it from the route, returning the data that way?
Here the current code for my route.
router.post('/twitter', (req, res) => {
let submission = req.body.trackChoice;
res.cookie('Track', submission);
let stream = client.stream('statuses/filter', {track: submission});
stream.on('data', (event) => {
try {
res.write(event && event.text);
} catch (err) {
new Error('There was an error');
}
})
})
This works for my practice purposes, but I don't know if having API libraries in my routes file is a good practice or not.
Thanks!
1 Answer

Alexander La Bianca
15,959 PointsThe way you are doing it is fine. Assuming you have your twitter set up outside of the routes/index.js . Like:
//in social.js
const Twitter = require('twitter');
module.epxports = function() {
return {
twitter: new Twitter({
consumer_key: 'secreitstuff',
consumer_secret: 'secretagain',
access_token_key: '121552124545f1adfas2df1sd5f',
access_token_secret: 'afafewfsadafasd4f5a45ffa'
})
}
}
//in routes/index.js
const social = require('./social');
const twitter = social().twitter;
//here now do all your routes
That way you keep all your api stuff in a separate file. And you can easily add more providers to the return in social.js like facebook or whatever else there is
I would also perhaps provide some other error handling to let the user know when errors occur
router.post('/twitter', (req, res) => {
let submission = req.body.trackChoice;
res.cookie('Track', submission);
let stream = twitter.stream('statuses/filter', {track: submission});
stream.on('data', (event) => {
try {
res.write(event && event.text);
} catch (err) {
res.status(500).json({msg: 'Server Error'})
}
})
stream.on('error',(err) =>{
res.status(500).json({msg: 'Twitter responded with an error. It is not my fault I promise'})
});
})