Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial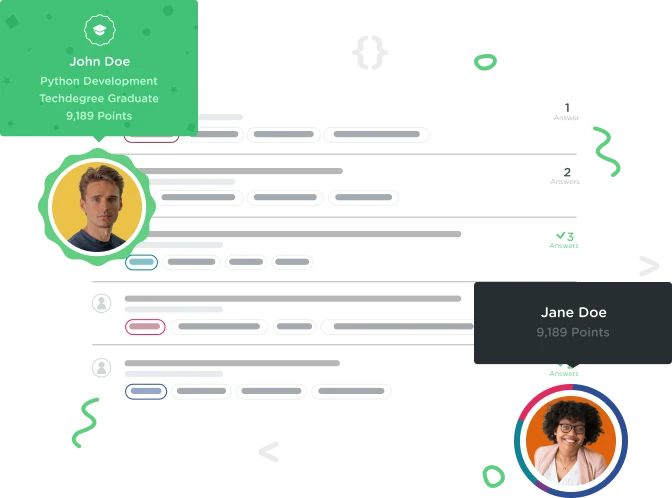
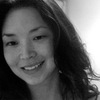
michikoote
20,646 PointsCalling method from another class
I created class named Question with string array and want to get single string one by one. There is MainActivty and I want to call the method from the class. What Am I doing wrong? I'm using Android Studio. Please someone help.
public class Question {
public void main() {
String[] mQuestion = {
"Monterey Jack",
"Abbaye de Belloc",
"Abondance",
"Airedale",
"Alverca",
};
for (int i = 0; i < mQuestion.length; i++) {
String singleQuestion = mQuestion[i];
}
}
}
//Part of MainActivity
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
//Creating constructor
Question question = new Question();
mQuestionView = (TextView) rootView.findViewById(R.id.tv_question);
//here- I want to load individual String using method I created from question.class
mQuestionView.setText(question.main());
}
2 Answers

Dan Johnson
40,533 PointsWhen dealing with properties, you need to define them outside any methods in order for them to be associated with the class. Also, when you want to return data, your method will need to have the appropriate return type in the signature.
It looks like you're trying to use the method main to initialize a Question object. You'll want to do that in the constructor instead. I'd also avoid using the name main for a method because if it has the signature:
public static void main(String[] args)
It will be treated as the entry point of the application.
Assuming you're trying to build something like a quiz app, here's a simple data model for a question that you could start with:
public class Question {
private String mPrompt;
private String mAnswer;
public Question(String prompt, String answer) {
mPrompt = prompt;
mAnswer = answer;
}
public String getPrompt() {
return mPrompt;
}
public String getAnswer() {
return mAnswer;
}
}
Then you could use a class similar in function to the FactBook class used in the Fun Facts app to setup and retrieve the questions:
public class TriviaBook {
private List<Question> mQuestions;
public TriviaBook() {
mQuestions = new ArrayList<Question>();
mQuestions.add(new Question("First question.", "answer to first question"));
mQuestions.add(new Question("Second question.", "answer to second question"));
}
public int getQuestionCount() {
return mQuestions.size();
}
public Question getQuestionAt(int index) {
return mQuestions.get(index);
}
public String getAnswerToQuestionAt(int index) {
return mQuestions.get(index).getAnswer();
}
public String getPromptToQuestionAt(int index) {
return mQuestions.get(index).getPrompt();
}
}
In MainActivity you could then select individual prompts/answers (or the entire Question object) by index:
TriviaBook triviaBook = new TriviaBook();
String firstAnswer = triviaBook.getAnswerToQuestionAt(0);
Assuming you had at least one question stored.
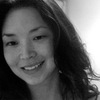
michikoote
20,646 PointsThank you. I tried your code and I'm getting NullPointerException at the line where the method "getAnswerToQuestionAt" is set. I have a feeling that int index has no value. I'm going to find out why. Thank you again.

Dan Johnson
40,533 PointsHere's an example app that just sets two TextFields to the value of the first question:
Java: https://gist.github.com/DanJ-01/978621cbbe90f0640aa2
MainActivity Layout: https://gist.github.com/DanJ-01/d85caeb5220667660f1a
Remember to change the package names so it works in your project if you try it.