Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial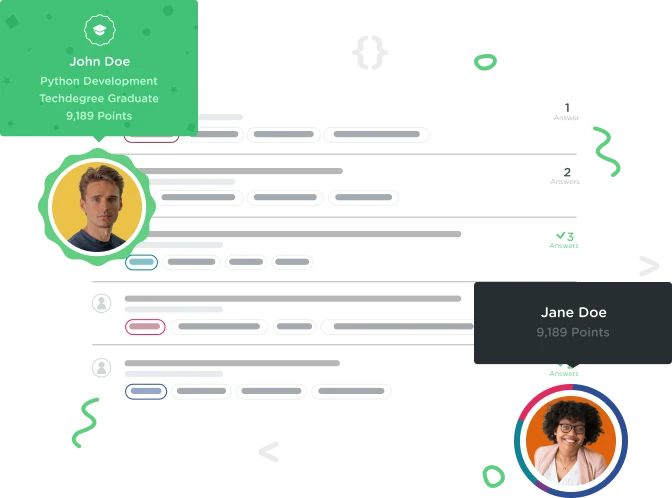

Lana Toogood
10,158 Pointscalling methods on new objects in javascript
Hi, I'm really confused about where and how you can call object methods in javascript. When I load the browser, I get "Uncaught ReferenceError: Stock is not defined". Can anyone tell me what concept I'm not understanding here?
In my stockApp.js file, I have this:
const stock = new Stock();
// calls addItemsToStock() and drawStockItems() on stock object on page load
document.onload = function(){
stock.addItemsToStock();
stock.drawStockItems();
}
And in my Stock.js file, I have this:
class Stock {
constructor(){
this.stockItemsArray = [];
}
addItemsToStock(){
const stockItemsArray = this.stockItemsArray;
const firestoreStock = db.collection('stock');
firestoreStock.orderBy('itemNo').get().then((snapshot) => {
snapshot.docs.forEach(doc => {
if (doc.exists) {
let item = Item.parseDocToItem(doc);
if (item) {
stockItemsArray.push(item);
console.log('new item object successfully added to stock array');
} else {
console.log('item object null: object was not added to stock array');
};
} else {
console.log('error getting document - does not exist');
};
});
});
}
drawStockItems(){
const currentStockDisplay = document.querySelector("#currentStockDisplay");
for (let item of this.stockItemsArray) {
let itemDiv = document.createElement('div');
let itemImage = document.createElement('img');
let itemName = document.createElement('p');
let itemRemove = document.createElement('div');
let itemRemoveButton = document.createElement('button');
itemDiv.setAttribute('class', 'col-6 col-sm-4 col-lg-3 edit');
itemDiv.setAttribute('data-id', item.id);
itemImage.setAttribute('src', item.imageURL);
itemImage.setAttribute('class', 'img-fluid mt-3');
itemName.textContent = item.name;
itemImage.setAttribute('alt', itemName.textContent);
itemRemove.setAttribute('class', 'row');
itemRemoveButton.setAttribute('class', 'removeButton col-8 col-sm-6 col-lg-4 mx-auto mb-3');
itemRemoveButton.textContent = 'REMOVE';
currentStockDisplay.appendChild(itemDiv);
itemDiv.appendChild(itemImage);
itemDiv.appendChild(itemName);
itemDiv.appendChild(itemRemove);
itemRemove.appendChild(itemRemoveButton);
}
}
}
1 Answer

scribbles
21,173 PointsIt seems like to Stock class is not declared or defined when you are instantiating it on the following line:
const stock = new Stock();
The stock class needs to be created before you instantiate it. Otherwise JavaScript doesn't know what you mean by new Stock(). It's likely that you aren't including the 'Stock.js' file.
If Stock.js is a separate file, the easiest way to include it would be to just like how you would any other .js file, something like this (in your html file):
<!DOCTYPE html>
<head>
</head>
<body>
<script src="path/to/Stock.js"> <!-- This one needs to be first -->
<script src="path/to/stockApp.js">
</body>
</html>
Lana Toogood
10,158 PointsLana Toogood
10,158 PointsYou're exactly right - it was the order of the files that was causing the problem. I had stockApp.js inserted above Stock.js. It is working perfectly now! I really appreciate your help :D