Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial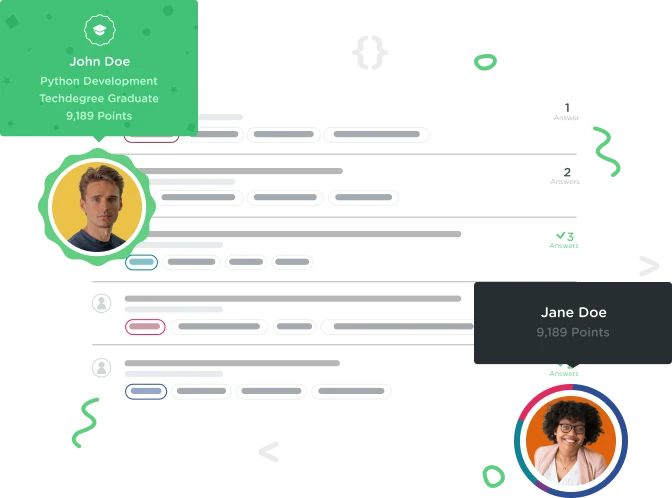

melissa brown
4,670 Pointscalling objects
in the button function why cant we just put dice() instead of dice.roll() since var dice has all the functions inside of it shouldnt it just run it the same way? button.onclick = function () { var result = dice.roll(); printNumber(result); });
2 Answers
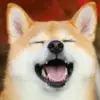
jobbol
Full Stack JavaScript Techdegree Student 17,885 Pointsvar dice = {
sides: 6,
roll: function(){
return Math.floor(Math.random() * dice.sides) + 1;
}
}
As far as syntax is concerned for this code, you can't use dice()
because it would refer to a function and dice is an object.
Even if syntax weren't an issue, the underlying problem is objects can contain multiple properties. The compiler has no way of knowing what you want to get from inside dice. It looks obvious to you because there's one function, but what if we had ten? There has to be a clear way of telling the compiler which property we need. This is why we use dot notation.
//Get the roll method from dice.
dice.roll();
//Get the number of sides from dice.
dice.sides;
If you really wanted to use dice()
you could make dice a function constructor and have it return the roll function. The downside to this is you cannot interact with it in any other way. This is much more complex than using just an object, and I don't recommend it unless you have a purpose to set it this way. You'll learn about constructors later on in this course, but it won't get to this degree.
/*** Dice Constructor ****/
var Dice = function(){
var that = this;
this.sides = 6;
this.roll = function(){
return Math.floor(Math.random() * that.sides) + 1;
}
return this.roll;
}
//create a new dice object.
var dice = new Dice();
//access the roll from dice.
console.log(dice());
//Throws an error because dice refers to the roll function and not itself.
console.log(dice.sides);

melissa brown
4,670 Pointsthank you that makes so much sense now
Gunhoo Yoon
5,027 PointsGunhoo Yoon
5,027 PointsIf object had dozens of methods and properties how does the program figure out which one to use? I wish programming language had an artificial intelligence that just read my mind but it doesn't