Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial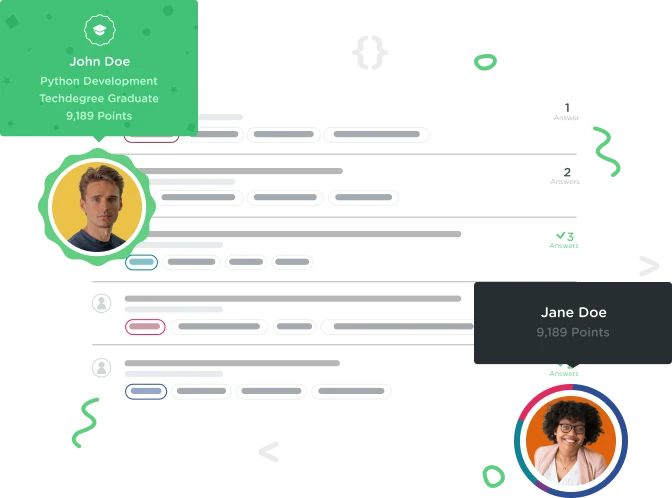
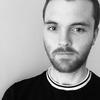
Nick Field
17,091 PointsCalling the super function...
In this video example, the super function is used to call methods on the parent class
class Student extends Person {
constructor({name, age, interestLevel = 5} = {}) {
super({name, age});
this.name = name;
this.age = age;
this.interestLevel = interestLevel;
}
}
let stevenJ = new Student ({name: 'Steven', age: 22, interestLevel: 1});
However, defining this.name = name and this.age = age in the sub-class is not needed, since those methods are already defined in the parent function already, so the following is valid-
class Student extends Person {
constructor({name, age, interestLevel = 5} = {}) {
super({name, age});
this.interestLevel = interestLevel;
}
}
Which case is better practice here? The second case is more DRY, are there any negative effects of using this approach?
4 Answers
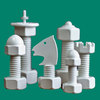
Steven Parker
231,269 PointsYour second example is a best-practice subclass.
Good job!
I notice in the video the subclass is made by copying the parent class and then editing it, I assume these two lines were just overlooked.
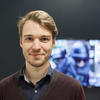
Lean Rasmussen
11,060 PointsI feel like the super({}) Is hardly explained am I the only one ?
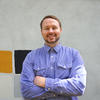
Tom Geraghty
24,174 PointsThe super keyword is used to call functions on an object's parent.
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/super

Jens Filipsson
12,870 PointsThanks Nick, your very question was what made me understand the super concept. With this.name = name
and this.age = age
defined in the sub class, the super function didn't make sense to me.
So once again, a big thank you!
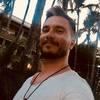
Peter Retvari
Full Stack JavaScript Techdegree Student 8,392 PointsMillion thanks for this question. I didn't understand either that WHY WE HAVE TO DEFINE THE NAME AND AGE if we inherit from the person class. And it could be 'super' frustrating when you just started to learn this concept.
M Ashraful Alam
12,328 PointsM Ashraful Alam
12,328 PointsExactly what I was going to ask, then I saw your question.