Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial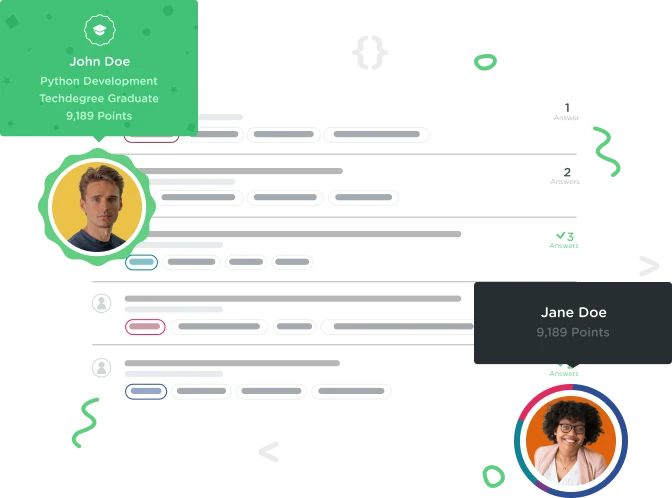
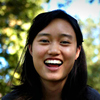
Christine Chang
1,917 PointsCan a do-try-catch block also throw an error?
Would it ever make sense to have a function that both catches and throws?
func myFunction(string: String) throws {
do {
try usingDoTryCatch(string)
} catch StringCheckErrors.EmptyStringError {
print(StringCheckErrors.EmptyStringError)
}
}
And would it ever make sense to have a try-only block that does not throw?
func myFunction2(string: String) {
try usingDoTryCatch(string) // NO catch - we can't tell user what's wrong. If there's no catch, does that mean the error isn't handled?
}
1 Answer

Simon Coates
28,694 PointsI don't know about ios, but in java the answer is yes, i think. you can catch the error, and fix the current object, then rethrow the error, so that something further up has to also handle the exception. (hoping i understood your question)
Christine Chang
1,917 PointsChristine Chang
1,917 PointsAh, I see. Thanks!
So can you try a string without catching or throwing? (Like in the second code sample)
Simon Coates
28,694 PointsSimon Coates
28,694 PointsI'm not sure about swift but sometimes it makes sense to let an exception occur, but this is usually when some other bit of code (that calls the current code) has a catch block. THere is usually a choice about where it makes sense to attempt recovery from an exception. In java for example, you can call a method that has has been marked as possibly causing an exception (in java you mark a method using the throws keyword), and should should use a try-catch block (swift seems to use the keyword 'do' in place to try), or indicate that the current method may cause an exception. This passes the responsibility of exception handling to the an earlier method.
(anyhow, i hope this makes sense. The syntax is probably just giving you options about where or if to handle the exceptions)