Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial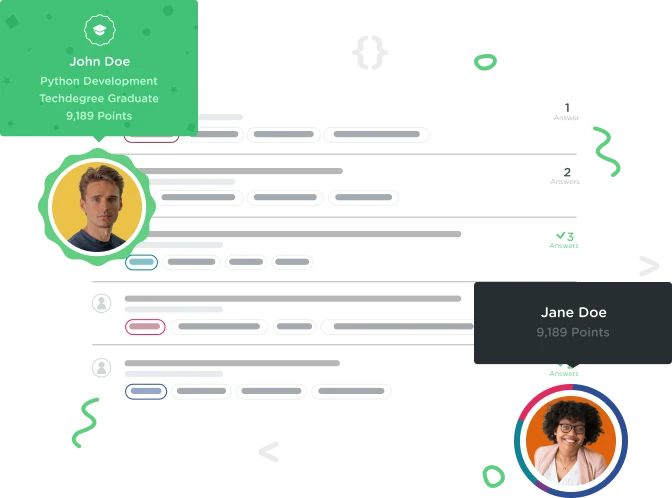

Jordan Miller
3,953 PointsCan anybody help me print the arrays to the list below, please?
I am really struggling with trying to print the arrays to the UL below the comment in my code. I have tried so many different options but I keep receiving error messages. If somebody could talk me through this and provide an answer I would be very grateful.
<?php
//edit this array
$contacts = array(
array (('Alena' => 'Alena Holligan', 'Alena' => 'alena.holligan@teamtreehouse.com')),
array (('Dave' => 'Dave McFarland', 'Dave' => 'dave.mcfarland@teamtreehouse.com')),
array (('Treasure' => 'Treasure Porth', 'Treasure' => 'treasure.porth@teamtreehouse.com')),
array (('Andrew' => 'Andrew Chalkley', 'Andrew' => 'andrew.chalkley@teamtreehouse.com')));
echo var_dump($contacts);
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>$contacts</li>\n";
echo "<li>$contacts</li>\n";
echo "<li>$contacts</li>\n";
echo "<li>$contacts</li>\n";
echo "</ul>\n";

Jordan Miller
3,953 PointsNow I have seen the way you've coded it, it is starting to make sense to me so thank you again for your help! Like my little guarding (coding) angel :)

Caleb Kemp
12,754 PointsGlad to hear it's starting to make sense :)
Caleb Kemp
12,754 PointsCaleb Kemp
12,754 PointsWell, for the first part, your arrays have to many parenthesis "(" in them, so
should be
Your second problem is putting your contacts array into the proper format, currently you have entries like
'Alena' => 'Alena Holligan'
. You don't want that. Your entries for names should be in the form'name' = 'Alena Holligan'
and'name' = 'Dave McFarland'
and so on. The same thing is also true for the emails.'Alena' => 'alena.holligan@teamtreehouse.com'
should be written as'email' => 'alena.holligan@teamtreehouse.com'
and so on.Your third problem seems to be how to reference the data. If you want information from the first person (Alena) in your
contacts
, you would write$contacts[0]
, for Andrew (4th person), you would write$contacts[3]
. for completeness here is how you would get Dave's name$contacts[1]['name']
and Treasure's email$contacts[2]['email']
Whew! I realize that may seem like a lot, however, there is still one more issue we must address, how do we add the variable to our echo statement? Unfortunately, the teacher did not cover this (that I could find), but it isn't too difficult. If you have the echo statement
echo "<li></li>\n";
If you wanted to have Dave's name in there, here is how you would do it.
echo "<li>" . $contacts[1]['name'] ."</li>\n";
Pay careful attention to where the 2 extra quotation marks
"
were added and that you need a dot.
on each side of the variable. Hope that helps!