Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial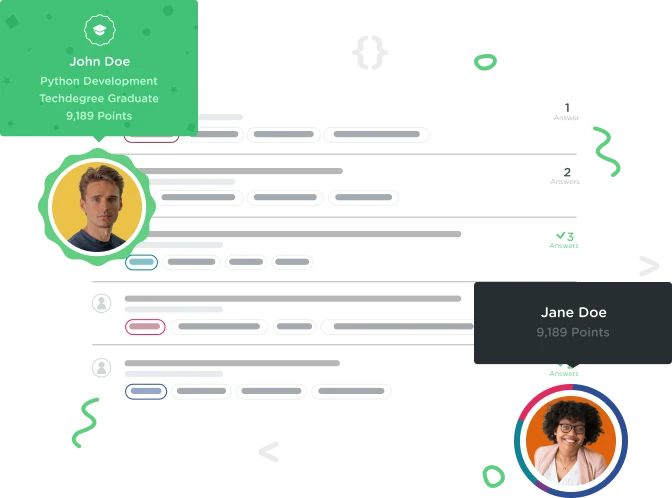

Jan M
1,053 PointsCan anyone explain what happend after 3:00 didnt get anything :(
Title says it all
1 Answer
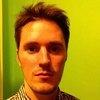
Brendan Whiting
Front End Web Development Techdegree Graduate 84,736 Pointsmy_alphabet_list = list('abcdefghijklmnopqrstuvwxyz')
count = 0
for letter in my_alphabet_list:
print('{}: {}'.format(count, letter))
count += 1
# prints out 0: a, 1: b.....
for index, letter in enumerate(my_alphabet_list):
print('{}: {}'.format(index, letter))
# also prints out 0: a, 1: b.....
This is where I understand you start having trouble. So in the enumerate function, what it gives us back is actually a series of two-tuples. But in the example above, we tell it to break apart the tuple into two variables - index and letter- before we ever see the tuple, and then we format our string with those variables. Now we're gonna actually use the tuple:
for step in enumerate(my_alphabet_list):
print('{}: {}'.format(step[0], step[1]))
So in the example above, we're actually capturing the tuple itself and calling it step. Then when we format our string, we're accessing the first part of the tuple at step[0] (which we previously called index) and the second part of the tuple at step[1] (which we previously called letter). Now we're gonna do something fancy with an asterisk:
for step in enumerate(my_alphabet_list):
print('{}: {}'.format(*step)) # step has to have the same number of items
Now in the example above, the reason this works is that the number of placeholder spots {} in the format string is the same as the number of items in the tuple, so we're just instructing it to put them into place in order. (If there had been three of these {} {} {} we would have had an error because there aren't enough items.)
Now on to dictionaries:
my_dict = {'name': 'Kenneth', 'job': 'Teacher', 'employer': 'Treehouse'}
for key, value in my_dict.items():
print('{}: {}'.format(key.title(), value))
The items() method on a dictionary also returns a series of two-tuple of key value pairs. Then similar to above, we break that tuple up into a variable called key and a variable called value before we ever see the tuple. The in the format string we're reference those variables and also capitalizing the key to make it prettier. The dictionary stuff is going to get fancier in the next video along the same lines as the stuff that was happening on the alphabet string.
Kevin Faust
15,353 PointsKevin Faust
15,353 PointsVery helpful. Im not sure why Kenneth did not explain half the stuff. He just put code on the screen, let it run, and then we moved on. What you wrote should be a replacement to the video