Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial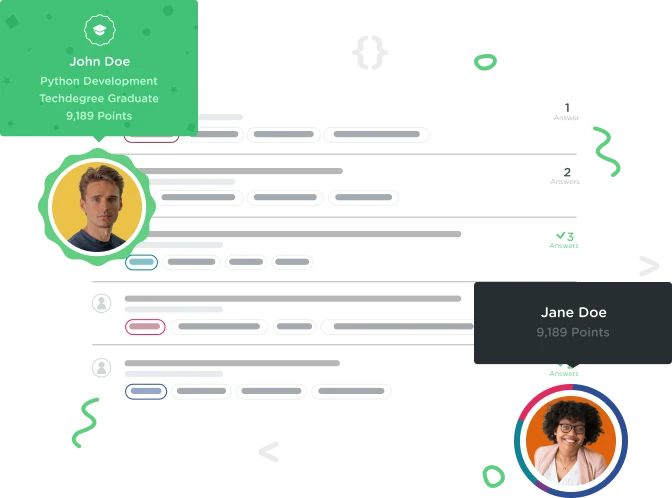

Megan Devenney
885 PointsCan anyone help me figure out what's wrong with my code please?
def valid_command?(command)
if (command == y) || (command == yes) || (command == Y) || (command == YES)
return "true"
end
end
3 Answers

Tim Knight
28,888 PointsHi Megan, I want to just add on to what Luke mentioned for you. Yes, what needed to happen was that you need to put quotation marks around those phrases because they are strings. In Ruby all strings have quote marks around them. One thing that doesn’t need a quote mark however are boolean statements, like true
or false
.
I didn’t see this exercise in the course so I imagine that you wrote this because you’re experimenting to learn a little more. Unless of course I just missed it. Because of that I thought I’d give you a different code example to expand on some of the concepts you’re playing with.
You could also write your code this way:
def valid_command?(response)
response.downcase!
response == “y” || response == “yes” ? true : false
end
Anytime I have to compare if something is valid with various cases I’ll typically just lowercase the input if the case of the input isn’t important. This lets me reduce the comparisons I need to make from 4 to 2. I do this with downcase
. Then I use a ternary operator to ask if both conditions are meet. Ternary operators have this format:
[conditional] ? [if true] : [if false]
Since Ruby will explicitly return the last value of a method you don’t necessarily need to use the return
keyword so I just write true
or false
.
puts valid_command?(“yes”) #=> true
puts valid_command?(“y”) #=> true
puts valid_command?(“no”) #=> false
Again, I know you’re just getting started, but since you were experimenting I thought I’d share some of my experience with you.
You could expand on these ideas and also try something like this:
def valid_command?(response)
response = response[0,1].downcase
response == “y” ? true : false
end
What [0,1] does is actually pull the first letter off of the left side of the string. This is a little less explicit, so something like “yesterday” would return true, but it just serves as part of my example. So now you could ask:
puts valid_command?(“yes”) #=> true
puts valid_command?(“y”) #=> true
puts valid_command?(“yup”) #=> true
puts valid_command?(“yeah”) #=> true
puts valid_command?(“no”) #=> false
puts valid_command?(“nope”) #=> false
Anyway, I just thought that would be interesting to investigate a little further. Good luck and have fun with your journey into Ruby.
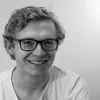
Luke Glazebrook
13,564 PointsHi Megan!
You need to encase your values you are testing for in speech marks. For example:
command == "YES"
I hope that this helped!

Megan Devenney
885 PointsThank you!! So simple and I couldn't even see it! :)