Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial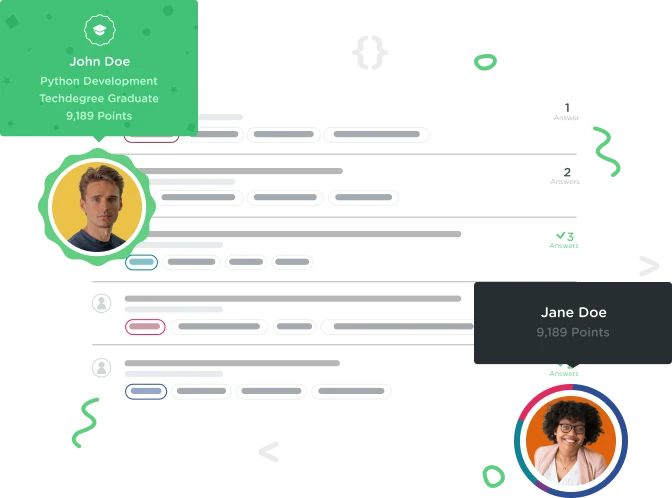

Frank Perez
30 PointsCan anyone help me fix this code ?
THIS IS WHAT I HAVE TO DO:
A DicePlayer is a class that represents the player in a Craps game. A DicePlayer is a data class. The data we need to manage (the fields or class variables) are the players name and how much money the player has to bet.
the player name (String)
cash on hand (Double)
Methods are needed to manage this data.
setName - take a string as the input parameter and assign it to the name variable
getName - return the value of the name
setCash - take a double as input and assign the value the the variable
getCash - return the value of the cash variable
addCash - take a double as the input parameter and add it to the cash variable if the input is nonnegative. If the given value is less than zero, return false, otherwise return true.
subtractCash - take a double as the input parameter and subtract it from the cash variable if the input is nonnegative and not greater than the cash on hand. If the given input is greater than the cash on hand or it is negative, return a false value. Otherwise return true
THIS IS MY CODE:
package diceplayer;
public class DicePlayer {
String playerName;
double cashOnHand;
public void setName(String name){
playerName = name;
}
public String getName(){
return playerName;
}
public void setCash(double cash){
cashOnHand = cash;
}
public double getCash(){
return cashOnHand;
}
public boolean addCash(double add){
boolean cashAdd = true;
if(add >= 0){
cashOnHand = cashOnHand + add;
}
else if(0 < cashOnHand){
cashAdd = false;
}
return cashAdd;
}
public boolean subtractCash(double sub){
boolean cashSub = true;
if(sub >= 0 && sub <= cashOnHand){
cashOnHand = cashOnHand - sub;
}
else if(sub > cashOnHand || sub < 0){
cashSub = false;
}
return cashSub;
}

Frank Perez
30 PointsTHIS IS THE CODE COMLETED:
package diceplayer;
public class DicePlayer {
String playerName;
double cashOnHand;
public void setName(String name){
playerName = name;
}
public String getName(){
return playerName;
}
public void setCash(double cash){
cashOnHand = cash;
}
public double getCash(){
return cashOnHand;
}
public boolean addCash(double add){
boolean cashAdd = true;
if(add >= 0){
cashOnHand = cashOnHand + add;
}
else if(0 < cashOnHand){
cashAdd = false;
}
return cashAdd;
}
public boolean subtractCash(double sub){
boolean cashSub = true;
if(sub >= 0 && sub < cashOnHand){
cashOnHand = cashOnHand - sub;
}
else if(sub > cashOnHand || sub < 0){
cashSub = false;
}
return cashSub;
}
public static void main(String[] args)
{
DicePlayer player = new DicePlayer();
player.setName("michael");
player.setCash(100);
System.out.println("Hello "+ player.getName());
System.out.printf(player.getName() + ", you have $%.2f on hand\n",player.getCash());
if(player.addCash(100)) //should work
{
System.out.println("Added 100 to cash");
}
else
{
System.out.println("failed to add 100 to cash");
}
if(player.addCash(-100)) //should fail
{
System.out.println("Added -100 to cash");
}
else
{
System.out.println("failed to add -100 to cash");
}
System.out.printf(player.getName() + ", you have $%.2f on hand\n",player.getCash());
if (player.subtractCash(12)) //should work
{
System.out.println("Withdrawal of 12 OK");
}
else
{
System.out.println("Withdrawal of 12 fail");
}
System.out.printf(player.getName() + ", you have $%.2f on hand\n",player.getCash());
if (player.subtractCash(300)) //should fail
{
System.out.println("Withdrawal of 300 OK");
}
else
{
System.out.println("Withdrawal of 300 fail");
}
System.out.printf(player.getName() + ", you have $%.2f on hand\n",player.getCash());
if (player.subtractCash(-1)) //should fail
{
System.out.println("Withdrawal of -1 OK");
}
else
{
System.out.println("Withdrawal of -1 fail");
}
System.out.printf(player.getName() + ", you have $%.2f on hand\n",player.getCash());
}
}
THIS IS THE RESULT:
Hello michael
michael, you have $100.00 on hand
Added 100 to cash
failed to add -100 to cash
michael, you have $200.00 on hand
Withdrawal of 12 OK
michael, you have $188.00 on hand
Withdrawal of 300 fail
michael, you have $188.00 on hand
Withdrawal of -1 fail
michael, you have $188.00 on hand
it should be "you have $100.00 on hand" instead of "200" because it failed to add the extra $100 and that is the problem I'm having.

Jason Anello
Courses Plus Student 94,610 Pointschanged category to java and fixed code formatting
7 Answers

Diar Selimi
1,341 PointsMaybe you should do this instead
public boolean addCash(double add){
boolean cashAdd = true;
if(add >= 0){
this.cashOnHand = this.cashOnHand + add;
}
else if(0 < this.cashOnHand){
cashAdd = false;
}
return cashAdd;
}
The problem is that your compiler can't find the cashOnHand variable inside the function so you should tell the compiler that you have to access the variable that is declared in the class by addin this.
before the variable, you should do this also in the subtract function.

Frank Perez
30 PointsI just tried what you said and still get the same results.

Jason Anello
Courses Plus Student 94,610 PointsHi Frank,
Aren't you getting the expected results?
You started out with $100 and then you successfully added 100 so I think at that point you should have $200.
However, I do think you have a bug with your addCash
method that isn't showing up with your testing code.
Try adding a negative cash value when you have 0 cash.
player.setCash(0);
if(player.addCash(-100)) //should fail
{
System.out.println("Added -100 to cash");
}
else
{
System.out.println("failed to add -100 to cash");
}
I didn't test this out but I think you'll find that "Added -100 to cash" gets printed.
public boolean addCash(double add){
boolean cashAdd = true;
if(add >= 0){
cashOnHand = cashOnHand + add;
}
else if(0 < cashOnHand){
cashAdd = false;
}
return cashAdd;
}
cashAdd never gets set to false because cashOnHand isn't greater than 0.
You want to set cashAdd to false if the add amount is negative.
However, it's better to switch the else if
to an else
and not have a condition there. There's less chance of making a logic error that way.
else {
cashAdd = false;
}
I recommend that you do the same for your subtractCash method.

Frank Perez
30 PointsJason Anello I tried what you told me but when I submit it this error comes up "call all methods with good and bad input"

Jason Anello
Courses Plus Student 94,610 PointsCan you provide more context on what you're doing here?
Is this an assignment where you have to submit it and pass a tester?
Are you getting any info about what test cases are failing?
Are you submitting your tests or do you only have to submit the DicePlayer class?
I did find a bug in the subtractCash method that I missed before. So maybe that's your problem when submitting.
if(sub >= 0 && sub < cashOnHand){
you're checking if sub is less than cashOnHand but you should be checking if it's less than or equal to cashOnHand. The instructions state that the amount can't be greater than the cash on hand which means it has to be less than or equal.
If you have $200, then you should be able to subtract $200 and have $0 cash on hand. Your method wasn't correctly handling that situation.
public boolean subtractCash(double sub){
boolean cashSub = true;
if(sub >= 0 && sub <= cashOnHand){
cashOnHand = cashOnHand - sub;
}
else {
cashSub = false;
}
return cashSub;
}

Frank Perez
30 Pointsyea, I have to submit it on zybooks and just the DicePlayer part NOT the main part. I submitted it like that and I still get this error when submitting it "call all methods with good and bad input"

Jason Anello
Courses Plus Student 94,610 PointsI'm not familiar with that. Is that all that it says?
I assumed that cashOnHand can't be negative.
The instructions don't specifically state this but maybe the setCash method needs to make sure that the argument is not negative although it doesn't tell you what you're supposed to do if it is negative.

Frank Perez
30 PointsWHAT TO DO:
A DicePlayer is a class that represents the player in a Craps game. A DicePlayer is a data class. The data we need to manage (the fields or class variables) are the players name and how much money the player has to bet.
the player name (String) cash on hand (Double) Methods are needed to manage this data.
setName - take a string as the input parameter and assign it to the name variable getName - return the value of the name setCash - take a double as input and assign the value the the variable getCash - return the value of the cash variable addCash - take a double as the input parameter and add it to the cash variable if the input is nonnegative. If the given value is less than zero, return false, otherwise return true. subtractCash - take a double as the input parameter and subtract it from the cash variable if the input is nonnegative and not greater than the cash on hand. If the given input is greater than the cash on hand or it is negative, return a false value. Otherwise return true.

Jason Anello
Courses Plus Student 94,610 PointsThat was what you posted at the top.
I meant, is that all the feedback you get from the tester?
"call all methods with good and bad input"

Frank Perez
30 Pointsyea, when I submit it thats all I get. i just asked my professor what does that mean and this is what he said.
"Your class has a bunch of methods. All me require input.
Your main method should test all of the object methods by calling all of them.
For those that require input you should different input. Add 10 to the player cash. Should work. Add -10 to player cash should not. Try both."

Jason Anello
Courses Plus Student 94,610 PointsSorry for all the back and forth but I'm not sure what you need to submit here.
You mentioned that you're only submitting the class but your professor is saying your main method should call all the object methods.
I would try to get an example of how you should be submitting your code.

Frank Perez
30 Pointsthank you man and don't worry, shoot me an email at ogamerboy@outlook.com if you don't mind. so I can send you there the whole thing and also reply faster. thank you once again.
Bryan Peters
11,996 PointsBryan Peters
11,996 PointsPerhaps this belongs in Java instead of JavaScript.
Also at first glance, its hard to tell if your code is missing the closing } for the class because of where your code markdown starts/ends. You might also describe the issue you are having.