Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial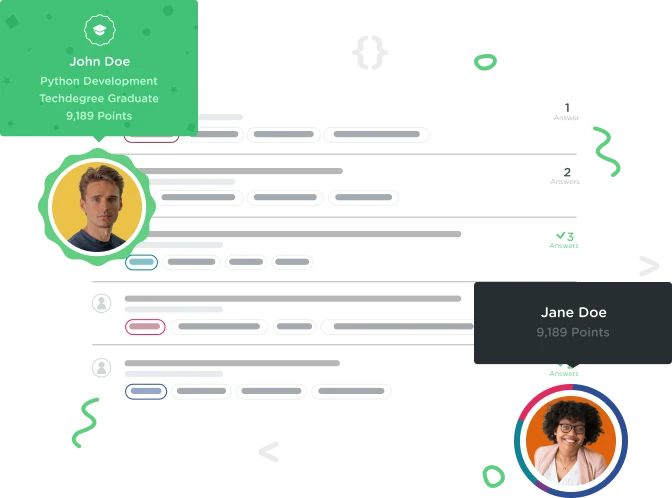
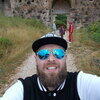
Alexandru Pescaru
6,410 Pointscan anyone help me with the corect code ai can figure iti out...
i can figure it out ....
const redButton = document.getElementById('redButton');
const blueButton = document.getElementById('blueButton');
redButton.addEventListener('click', (e) => {
const redButton = document.getElementById('redButton');
redButton.style.backgroundColor = 'red';
});
blueButton.addEventListener('click', (e) => {
const blueButton = document.getElementById('blueButton');
blueButton.style.backgroundColor = 'blue';
})
div {
height: 50px;
float: left;
padding-top: 40px;
padding-left: 20px;
}
#colorDiv {
padding: 0;
width: 100px;
height: 100px;
background-color: gray;
}
button {
height: 30px;
border-radius: 10px;
}
#redButton {
background-color: #ff5959;
border-color: red;
}
#blueButton {
background-color: lightblue;
border-color: #7C9EFC;
}
2 Answers

Helari Sosi
6,168 PointsHi Alexandru,
You have already declared const redButton = document.getElementById('redButton');
at the top of your file. Using const
you can't declare it twice plus there is no need to declare it in the function. You can read more about how to properly declare variables here - Variables.
redButton.addEventListener('click', (e) => {
const redButton = document.getElementById('redButton'); //try your code without this line(delete it)
redButton.style.backgroundColor = 'red';
});
Let me know if you have any more questions.

Helari Sosi
6,168 PointsSorry, you didn't add the original code snippet in your post. I actually didn't look the challenge itself. So what you need to think is that const
is block-scoped, meaning that it is not accessible outside the function it is in. Only the function it is in can use this and since there is a second funtion to change the color blue, it's not accessible there. You need to move this
line of code on the top, with rest of the variables so every function can get access do it.
const colorSquare = document.getElementById('colorDiv');
All you need to do is -
const redButton = document.getElementById('redButton');
const blueButton = document.getElementById('blueButton');
const colorSquare = document.getElementById('colorDiv');
redButton.addEventListener('click', (e) => {
colorSquare.style.backgroundColor = 'red';
});
blueButton.addEventListener('click', (e) => {
colorSquare.style.backgroundColor = 'blue';
})
And I would still recommend looking thorugh what differences there are between variables const, let & var
. It's the basics and the core of the language :)
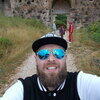
Alexandru Pescaru
6,410 Pointsso i havd to declare colorSquare constant and get the elements by ID .... i didn't think about that thx very much! You been a great help!
Alexandru Pescaru
6,410 PointsAlexandru Pescaru
6,410 Pointsso i tried to put the code that you gave me in the challange and it says: The
colorSquare
variable cannot be seen by the two buttons. Has it still been declared?