Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial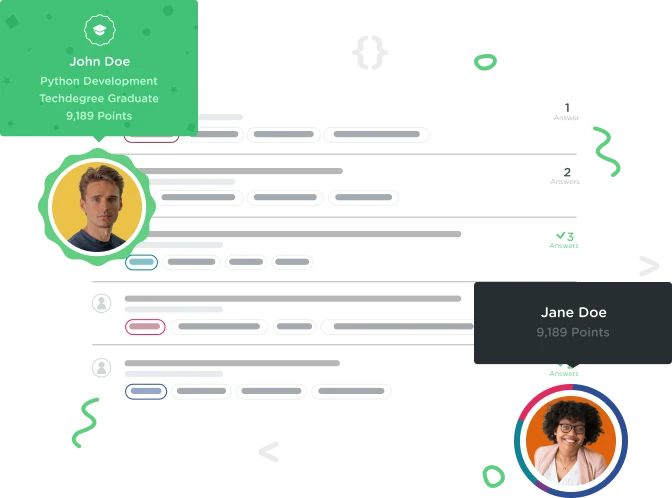

Matthew Byrne
17,558 PointsCan create user in Rails Console, but can't with HTML Form
So I've run through a bunch of courses/tracks here at Treehouse, and have also worked through railstutorial.org once already. Now I'm running through the rails tutorial again with some changes and customization, and have run into an issue I haven't been able to figure out:
I'm currently creating a User Sign Up for the application, and the HTML form won't create users, or even validation the input properly (it gives all errors, even with valid entries). In the rails console, I'm able to create a new user, find that it's valid or invalid, get the correct error messages with invalid data, and save the user with valid data.
Upon clicking the submit button in the, the rails server gives me this message: (0.0ms) BEGIN User Exists (81.0ms) SELECT 1 AS one FROM 'users' WHERE 'users'.'email' IS NULL LIMIT 1 (0.0ms) ROLLBACK
There is no data in the DB when receiving this message, I've checked many times.
It's driving me crazy, so hopefully someone can take a look and give me a pointer or two!
Thanks in advance!
Here's some of the code:
User controller file:
class UsersController < ApplicationController
def index
end
def show
@user = User.find(params[:id])
end
def new
@user = User.new
end
def create
@user = User.new(params[user_params])
if @user.save
flash[:success] = "Welcome to FFBL!"
redirect_to @user
else
render 'new'
end
end
private
def user_params
params.require(:user).permit(:name, :email, :team, :password,
:password_confirmation)
end
end
Model user.rb file:
class User < ActiveRecord::Base
validates :name, presence: true, length: { maximum: 50 }
VALID_EMAIL_REGEX = /\A[\w+\-.]+@[a-z\d\-]+(\.[a-z\d\-]+)*\.[a-z]+\z/i
validates :email, presence: true, length: { maximum: 255 },
format: { with: VALID_EMAIL_REGEX },
uniqueness: { case_sensitive: false }
before_save { self.email = email.downcase }
validates :team, presence: true, length: { maximum: 50 }
has_secure_password
validates :password, presence: true, length: { minimum: 6 }
end
View new.html.erb file (HTML Form):
<% provide(:title, "Sign up") %>
<h1>Sign Up</h1>
<div class="row">
<div class="col-md-6 col-md-offset-3">
<%= form_for(@user) do |f| %>
<%= render 'shared/error_messages' %>
<%= f.label :name %>
<%= f.text_field :name, class: 'form-control' %>
<%= f.label :email %>
<%= f.email_field :email, class: 'form-control' %>
<%= f.label :team %>
<%= f.text_field :team, class: 'form-control' %>
<%= f.label :password %>
<%= f.password_field :password, class: 'form-control' %>
<%= f.label :password_confirmation, "Confirmation" %>
<%= f.password_field :password_confirmation, class: 'form-control' %>
<%= f.submit "Create FFBL Team", class: "btn btn-primary" %>
<% end %>
</div>
</div>
And finally, the Shared _error_messages.html.erb:
<% if @user.errors.any? %>
<div id="error_explanation">
<div class="alert alert-danger">
The form contains <%= pluralize(@user.errors.count, "error") %>.
</div>
<ul>
<% @user.errors.full_messages.each do |msg| %>
<li><%= msg %></li>
<% end %>
</ul>
</div>
<% end %>
1 Answer

Seth Kroger
56,413 PointsLooking over the code from ODOT and railstutorial.org the strong params function is supposed to be used on its own, not by params[user_params].
def create
@user = User.new(user_params)
# ...
Matthew Byrne
17,558 PointsMatthew Byrne
17,558 PointsThanks for taking the time Seth, that's absolutely the culprit. I really appreciate it!
Seth Kroger
56,413 PointsSeth Kroger
56,413 PointsNo problem!