Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial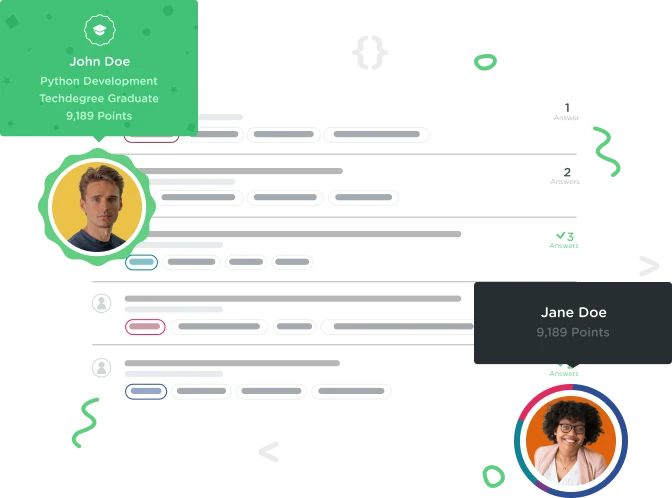
Kai Aldag
Courses Plus Student 13,560 Pointscan Dictionaries hold multiple values.
Hi guys so I'm making a quotes app and I'm using an NSDictionary to store the quote strings, I added an image view and when i call the randomQuote method i made i want it to pass the string to the label and an image to the image view, would a dictionary be the right choice. if so how do you pass in 2 values for one key and then how to 2 different view objects call the 2 different values I'm adding.
thanks, Kai.
1 Answer
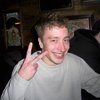
Brett Doffing
4,978 PointsAn NSDictionary can indeed hold multiple values, although you can't have two values for one key. I think the example below does what you want.
NSMutableArray *arrQuotes = [[NSMutableArray alloc] init];
NSDictionary *dictQuote1 = [NSDictionary dictionaryWithObjectsAndKeys:@"Quote 1", @"quote", [UIImage imageNamed:@"imageForQuote1.png"], @"image", nil];
NSDictionary *dictQuote2 = [NSDictionary dictionaryWithObjectsAndKeys:@"Quote 2", @"quote", [UIImage imageNamed:@"imageForQuote2.png"], @"image", nil];
[arrQuotes addObject:dictQuote1];
[arrQuotes addObject:dictQuote2];
This way you could randomly select from the array of quotes (arrQuotes), which will give you a dictionary. You can then access the quote value with the key @"quote", and the image value with the key @"image", from the dictionary.
Kai Aldag
Courses Plus Student 13,560 PointsKai Aldag
Courses Plus Student 13,560 PointsOk but now how would i get the string to the label and image the the imageView if there were a couple strings and images. Becasue if a had 3 string and 3 images and each string coresponds to an image i need to display a string and the coresponding image to the view.
Brett Doffing
Brett Doffing
4,978 PointsBrett Doffing
4,978 PointsThere are many ways you could go about getting such results, as such, I wouldn't know exactly how you would imagine implementing this functionality, but for the sake of completion, I'll try an example. Let's say you have an Image View and three buttons. Assign a corresponding tag value (int) to the tag property of each button. arrQuotes could be a property of the View Controller, and in your viewDidLoad method you could do as I had done previously, or like so:
Then you could have a method (or IBAction) that checks to see what button is tapped, and go from there.
Kai Aldag
Courses Plus Student 13,560 PointsKai Aldag
Courses Plus Student 13,560 Pointsok then i can display them randomly by making a method that has the arc4randomuniform pass in the length of 3 and randomly display one of those strings and image through that. btw what is the .tag for, i understand the sender but the .tag is new to me Brett Doffing
Brett Doffing
4,978 PointsBrett Doffing
4,978 Points.tag is an integer property that UI elements have
Kai Aldag
Courses Plus Student 13,560 PointsKai Aldag
Courses Plus Student 13,560 Pointsok thanks. and one last question can arrays store arrays. Brett Doffing
Brett Doffing
4,978 PointsBrett Doffing
4,978 PointsAbsolutely!