Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial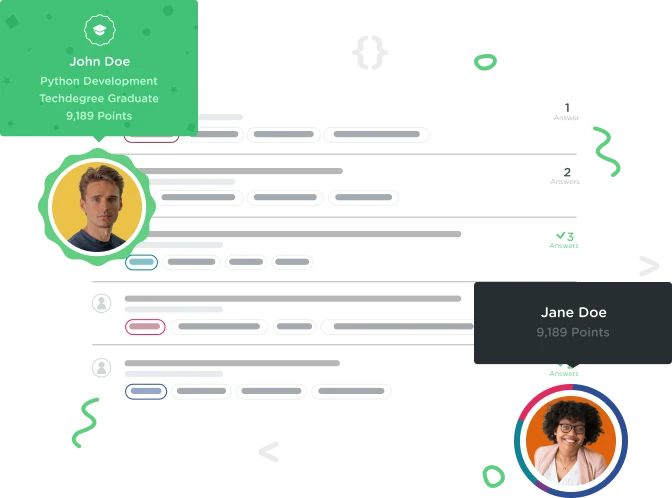

Rick S
11,273 PointsCan I add code to this that will allow me will output the status as <resolved> the way it does <pending>?
Can I add code to this that will allow me will output the status as <resolved> the way it does <pending>?
I see references to Promise object keys PromiseStatus and PromiseValue.
as in the link in the teachers notes : https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise/then
I'm trying to understand how the fulfillment value relates to these keys.
6 Answers

Rick S
11,273 PointsThe later track I mentioned showed my how to play around and use the console to answer some of my questions.
In my Chrome browser the output to console is:
▼Promise {<resolve>: undefined} //unfolds to show three properties:
►__proto__: Promise
[[PromiseStatus]]: "fulfilled"
[[PromiseValue]]: undefined
Note that in Safari's javascript console, this same output is shown as:
▼Promise //shows same three properties but with different names
? result: undefined // ? icon in front of may represent 'undefined'
S status: "resolved" // S icon in front of it may indicate a string type
►Promise Prototype
This indicates to me that these "resolved" and "fulfilled" are strings chosen as defaults by the different javascript engines used by Chrome and Safari. The resolve() function apparently is allowing a way to override these default strings, while resolving the promise.
It also cleared up some doubt I had about the use of resolve and reject as parameters.
const promise1 = new Promise((resolve, reject) => {
resolve('Success!');
});
I could name these anything as long as they match what is used in the body of the function:
const promise1 = new Promise((rainyDay, reject) => {
rainyDay('Success!');
});
This makes sense, but I was in doubt since resolve() is mentioned in the documentation for Promise as a built-in named function. I was wondering if I was supplying the resolve() as I would a parameter in a function call. But the javascript supplies the resolve() function to the formal argument no matter what I name it. Even though I might have expectations on how it should work, I can't help but have doubts since this is all new to me, not just Promises. Being able to play around in the console really helped.
Thanks for the feedback. Of course having moved on to fetch(), looks like I won't be using resolve() anyway. But I feels better knowing what's going on rather than just knowing how to apply something that I don't understand.

Reggie Williams
Treehouse TeacherOf course, again great work going further. For reject/resolve that's sort of what I meant, usually parameter names will be arbitrary an just need to match what you use inside the function body. For example once with for in loops you can name thee object being looped through and the individual items anything you want as the method expects values to be given for both an iterable object and an index of that object. Your curiosity and drive to figure things out will really serve you well on this journey and I wouldn't have known you were newer to js if you hadn't mentioned it. Keep up the great coding and questions!

Reggie Williams
Treehouse TeacherRick Sweeney the documentation here mentions that you can't access the state properties. I'm not sure why the difference but it may be because resolved is the ideal state so the user is only informed of pending/ rejected states

Rick S
11,273 PointsIn the Exploring Async/Await track's " Combine Async/Await with Promises" video it actually uses the console to demonstrate changes in PromiseStatus and PromiseValue, so this will be helpful.

Reggie Williams
Treehouse TeacherAh I see where you're coming from. This is something you'll see other times but my best explanation is that the promise is intended to handle the response so it expects and is programmed to recognize the first argument of the function as the value. Despite the how being cloudy you've already shown a lot of understanding of the what which is most important to use it

Rick S
11,273 PointsWhen I proceed to the next treehouse video and use reject instead of resolve, ie.
reject('Oh no! There was a problem with your order.');
then from the code
console.log(breakfastPromise);
I do get the output:
Promise { <rejected> 'Oh no! There was a problem with your order.' }
Console.logs show the status in the case of <pending> and <rejected> status, but not in the case of <resolved>, in which case it only shows
Promise {'Your order is ready. Come and get it!' }
So I'm wondering why the difference and if the status it shows are values held by PromiseStatus.

Rick S
11,273 PointsI'm guessing that means you can't access them directly by appending .state , but I'm wondering if as a built-in function that is what then() is accessing for you-- that is, then() can access .state but you can't.. Or is it just a string written into the method or the particular browser that it uses when a response is received. This is coming from someone who is not just new to promises, but also javascript, so I'm in a sense feeling my way around trying to better understand.

Reggie Williams
Treehouse TeacherEffectively that is what it's doing, accessing the state for you. The documentation for promises isn't too deep so don't worry. Great work digging for more understanding! Although it may not have the property you can still base any conditionals you would write on your promise response containing the terms pending/ rejected or neither.

Rick S
11,273 PointsWell, in the link I mentioned, it says that .then()'s first parameter is a callback function that itself has the "fulfillment value" as its argument, which the link example names "value". But it could have been named "val", it doesn't matter. It's just a formal argument named by the programmer.
const promise1 = new Promise((resolve, reject) => {
resolve('Success!');
});
promise1.then((value) => {
console.log(value);
// expected output: "Success!"
});
But then() knows what this "value" parameter will access, which in the example is the parameter that was provided to resolve(). So I want to know how then() knows what was provided as the parameter in resolve, but the documentation doesn't make this clear.
The only clue given as far as I can tell is in the middle of the page where it shows some example output:
// logs, in order:
// Promise {[[PromiseStatus]]: "pending", [[PromiseValue]]: undefined}
// "this gets called after the end of the main stack. the value received and returned is: 33"
// Promise {[[PromiseStatus]]: "resolved", [[PromiseValue]]: 33}
So I might guess that resolve() is changing the value of PromiseValue and the "value" parameter from the then() is accessing the value of PromiseValue. But as a newbie, I can also easily see that for whatever reason I may be wrong. Seems like this should have been explained in the documentation, and in the treehouse video if it were known, but instead is one of those things that gets glossed over, but as far as I can tell is a key part of understanding what is taking place. If you compound this example of leaving out this detail with other similar examples of missing details, then, yes, promises will be difficult to understand-- unnecessarily so it seems to me. Unnecessary trips down rabbit holes.
Anyway, thanks for the response. I hope I've explained where my confusion comes from.
Reggie Williams
Treehouse TeacherReggie Williams
Treehouse TeacherHey Rick Sweeney, once the promise is resolved the status becomes the value passed into resolve. You could pass resolved in as a value to accomplish this