Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial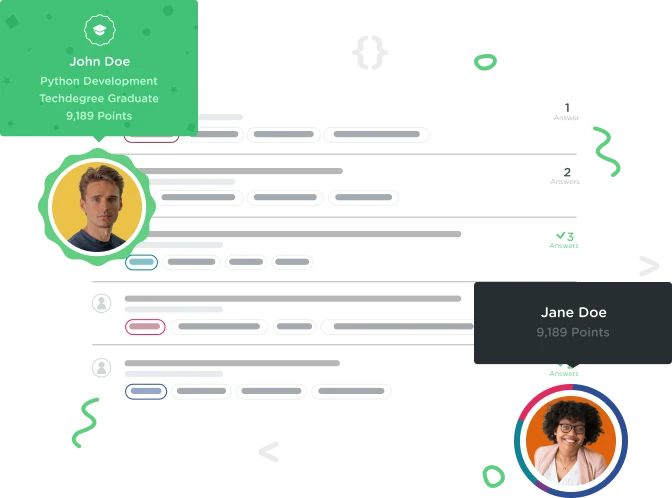

Omotayo Olawepo
2,803 PointsCan i add sound to the button so when clicked on it makes a sound
Can i add sound to the button so when clicked on it makes a sound
2 Answers
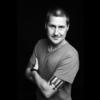
Jens Hagfeldt
16,548 PointsHi Omotayo
Yes, it is possible to add sound to the button so when it is clicked it makes the sound. Off course you must add the sound file to the project and connect the button as usually with an @IBAction. If you import AVFoundation into the View Controller that the button belongs to, you can then use that to play the sound file when the button is pressed. You can find my solution here below:
import UIKit
import AVFoundation
class ViewController: UIViewController {
var soundEffectPlayer: AVAudioPlayer?
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
}
func playSoundEffect(filename: String) {
let url = NSBundle.mainBundle().URLForResource(filename, withExtension: nil)
if (url == nil) {
print("Could not find file: \(filename)")
return
}
var error: NSError? = nil
do {
soundEffectPlayer = try AVAudioPlayer(contentsOfURL: url!)
} catch let error1 as NSError {
error = error1
soundEffectPlayer = nil
}
if let player = soundEffectPlayer {
player.numberOfLoops = 0
player.prepareToPlay()
player.play()
} else {
print("Could not create audio player: \(error!)")
}
}
@IBAction func playSoundButtonPressed(sender: AnyObject) {
playSoundEffect("pop.wav")
}
}
I hope this helped you... Keep coding :) / Jens

Omotayo Olawepo
2,803 PointsHello sorry im abit lost how would i apply it in this particular code
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var funFactLabel: UILabel!
@IBOutlet weak var funFactButton: UIButton!
let factBook = FactBook()
let colorWheel = ColorWheel()
override func viewDidLoad() {
super.viewDidLoad()
funFactLabel.text = factBook.randomFact()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func showFunFact() {
var randomColor = colorWheel.randomColor()
view.backgroundColor = randomColor
funFactButton.tintColor = randomColor
funFactLabel.text = factBook.randomFact()
}
}