Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial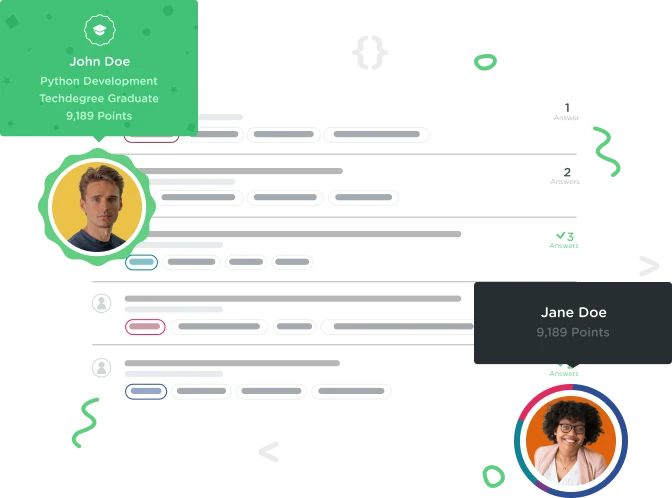
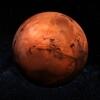
Duane Smith
1,919 PointsCan i assign a method inside of a method?
For some reason I'm not connecting the dots on how to complete this particular challenge, though it should be very easy. I'm trying to figure out how I can assign arguments that hold methods so I can print the statements I need. I'm not sure what I'm missing or if I'm even attacking this correctly.
import random
class Student:
name = "Your Name"
#grade = random.randint(0,101)
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
grade = random.randint(0,101)
if grade > 50:
print(f"{self.praise}")
return print(f"{self.reassurance}")
1 Answer

Benyamin Kohanchi
Courses Plus Student 2,342 PointsHey Duane, a lot of the code you wrote is not necessary to complete this challenge, especially the random module which you imported and the random.randint() function which you used; its a lot more simple.
Regarding the grade, which you tried assigning to random.randint(), don't worry about that. When you run this code, grades will be thrown into your code to see whether your code has the right output. In simpler terms, don't worry about assigning the grade, that is taken care of.
Since grade is not an issue, you can remove the Random module which you imported above (the import random) and the random.randint() function. After this is done, your code will be a lot closer to the answer then it was before; the Random module was holding you back, in other words.
Your code without the random module will look something like this:
class Student:
name = "Your Name"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
if grade > 50:
print(f"{self.praise}")
return print(f"{self.reassurance}")
This is way closer to the answer compared to your original code, but it is still incorrect; you would get this error if you ran it:
Exception: argument of type 'NoneType' is not iterable
Now in the method feedback which you wrote, you have the gist of what needs to happen. Instead of using the print function with an f string with placeholders -- print(f'{self.praise}'), you can simply call on the praise method by using the return function and self.praise(). The same goes with self.reassurance.
In the end, your code should look like this:
lass Student:
name = "Your Name"
def praise(self):
return "You inspire me, {}".format(self.name)
def reassurance(self):
return "Chin up, {}. You'll get it next time!".format(self.name)
def feedback(self, grade):
self.grade = grade
if grade > 50:
return self.praise()
return self.reassurance()
When comparing your code to the correct code, you can see it is not entirely different. You basically did the challenge correctly, but you had a few obstacles to overcome.
All aside, good job! If you have any questions, feel free to comment them below.
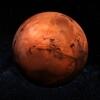
Duane Smith
1,919 PointsAhhh I see! I tried it first without the random import, but I was getting that error message you mentioned above. So I was confused as to what I was doing wrong, so i figured I needed to give 'grade' a range to work within to make the if statement function properly. Setting Self.Grade = Grade didn't even cross my mind, but now I understand why it's there!

Benyamin Kohanchi
Courses Plus Student 2,342 PointsGreat, I'm happy I was able to help. If you have any questions, you can always @Benyamin Kohanchi me. Good luck!
Duane Smith
1,919 PointsDuane Smith
1,919 PointsBy the way, the question for this problem was:
'Alright, I need you to make a new method named feedback. It should take self and an argument named grade. Methods take arguments just like functions do.
Inside the feedback method, if grade is above 50, return the result of the praise method. If it's 50 or below, return the reassurance method's result.'