Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial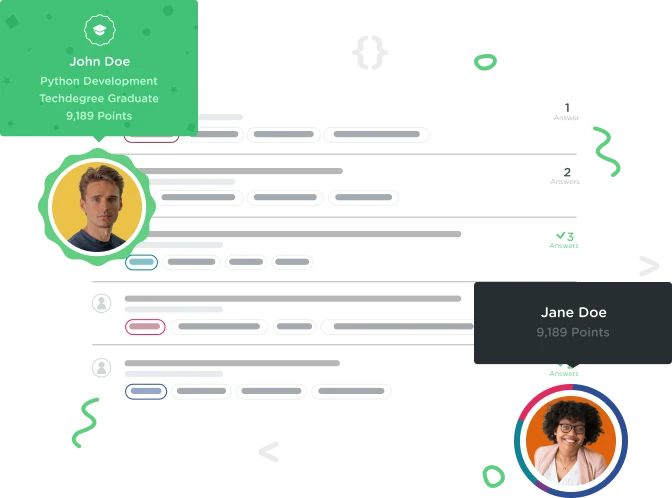

Carolyn Sauck
976 PointsCan I create a method getTileCount with no char input that returns an integer and calls char tile in an enhanced forloop
I don't get any compiling errors when I run the code, but the system does not recognize the getTileCount method I created. Error: Bummer! Did you forget to create getTileCount method with char input?
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(){
int tileCount = 0;
for(char tile: mHand.toCharArray()){
if(mHand.indexOf(tile)>=0){
tileCount++;
}
}
return tileCount;
}
}
2 Answers
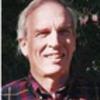
jcorum
71,830 PointsThe challenge wanted a method that takes a tile and counts how many of them are in a hand. So you need a parameter for the method. And then you need to see if each tile in the hand matches the one passed in to the method:
public int getTileCount(char tile){
int tileCount = 0;
for(char t: mHand.toCharArray()){
if(t == tile ){
tileCount++;
}
}
return tileCount;
}
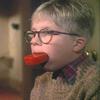
Sean Gallagher
2,054 PointsI can see how jcorum's solution works, is there a reason you can't call hasTile to do the compare?
public int getTileCount() {
int counter = 0;
for (char tile: mHand.toCharArray()) {
if (hasTile(tile)) {
counter++;
}
} return counter;
}
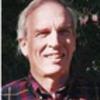
jcorum
71,830 PointsThere are several reasons.
First, the editor won't accept it, because the editor requires a char parameter for the getTileCount() method.
Second, even if we overlook what the editor wants, it still doesn't work. For any hand of 8 tiles this version will return a count of 8. Why? Because it is not comparing some given tile, say 'e', with the tiles in the hand, say 'srecihak' (the tile and hand the editor uses). In the loop it is taking each tile from the hand, and asking if the hand has that tile. And of course, the answer is always "Yes".
Sean Gallagher
2,054 PointsSean Gallagher
2,054 PointsGreat explanation here and below. Thanks!