Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial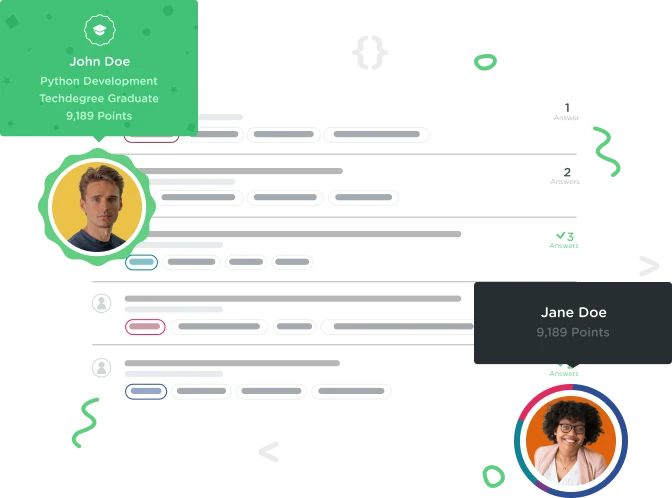
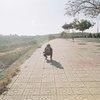
Johnny Nguyen
3,875 PointsCan I do random like this? How Pasan's way different from me?
func random(_ n:Int) -> Int { return Int(arc4random_uniform(UInt32(n))) }
@IBAction func changeLabel() {
funFactLabel.text = factProvider.facts[random(factProvider.facts.count)]
}
}
3 Answers
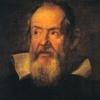
landonferrier
25,097 PointsYes this can be done. Pasan is using the standard methods but using custom in a case like this is 100% fine.
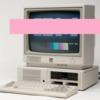
kols
27,007 PointsYou can use the arc4random_uniform
as an alternative way to generate a random number; however, in addition to using a different method for generating a random number, Pasan is actually trying to get you to generate a random fact (String) as the return value, not just the index (he actually passes this value through as a part of the method itself). So, in order to achieve the same thing as the video — but while using your method instead of the GameKit random generator option — you'd want to:
struct FactProvider {
let facts = [ "My first fact goes here",
"This is the second fact",
"A third fact can be placed here on this line",
"Fourth fact of the array goes here",
"I love facts" ]
// Arc4Random_uniform number generator
func random(_ n:Int) -> String {
return facts[Int(arc4random_uniform(UInt32(n)))]
}
}
And in the view controller file, you'd instead add:
funFactLabel.text = factProvider.random(factProvider.facts.count)
The main reason for why you'd want to do this (generate an actual fact rather than simply an index value that you have to pass through) is that you can actually pass the final value around more easily, and this better achieves the DRY (Don't Repeat Yourself) principle by cutting down on the code required.
Hope this explains the difference a little better!!

Andrew Czachowski
3,039 PointsI believe in the current version of Swift you can also do
Int.random(in: range)