Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial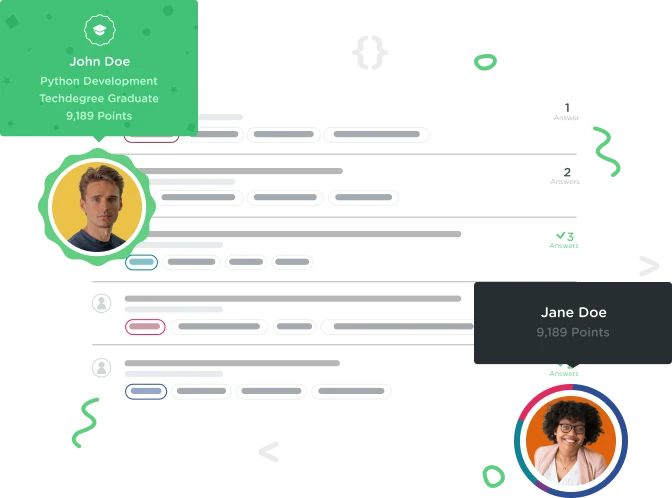

mryoung
4,156 PointsCan I do this? (function inside of a function)
I have a feeling I'm missing something here...
I have 2 arrays, one with id's of images and the other with sayings that appear in a list when the image is clicked. I want the user to be able to click on as many images as they want, and it populates the list according to the matched up saying.
So, I made a for loop, it runs through the array of images, and adds 1 to the counter. There's an onclick event that populates the list with a saying matching the appropriate index number.
Is it that I'm missing another for loop, my syntax is messed up, or is this not possible?
var images = ['#image1', '#image2', '#image3', '#image4'];
var thisList = ['saying1', 'saying2', 'saying3', 'saying4'];
for(let i = 0; i < images.length; i++) {
images[i].addEventListener('click' => {
myList.innerHTML = '<li> .thisList[i] </li>';
});
};
2 Answers
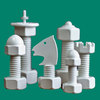
Steven Parker
231,269 PointsThere's nothing wrong with the concept, but you have a few implementation issues.
First, the "images" array contains only strings, and you can't attach event listeners to strings. You probably want to pass those strings to a selector function like document.querySelector. A few other issues:
- you need a comma after the first argument to addEventListener ('click')
- you need a parameter name or a pair of parentheses in front of the arrow operator
- "myList" isn't defined in this code, is it in a part not included here?
- if you're trying to do string interpolation the syntax would be as shown below:
myList.innerHTML = `<li>${thisList[i]}</li>`;

mryoung
4,156 PointsThank you, I had this:
document.querySelector(images[i]).addEventListener("click", () => {
myList.innerHTML = `<li>${thisList[i]}</li>`;
like this
images[i]).addEventListener("click", () => {
myList.innerHTML = `<li>${thisList[i]}</li>`;
I didn't realize I would have needed to add the querySelector into the function, is that typical? I thought I could just add the event listener to images variable, since I already grabbed them with the querySelector.
Also, why is the innerHTML written that way, is it part of ES6?
Sorry for the 20 questions, I'm trying to learn.
mryoung
4,156 Pointsmryoung
4,156 PointsThank you, I've made the changes you mentioned and still don't get results. How can i test the click function? It'd be easier to diagnose my problems if I could see errors in the console, otherwise I'm blind to what's not working.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsYou didn't show your code after the changes, so I'm not able to say what went wrong. But after applying the previous hints, I would expect to see something like this:
Also, I'm only guessing about what's in the HTML. To facilitate a more complete analysis, always show all the code. If you're using the workspaces, the "snapshot" function can be very handy for sharing.