Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial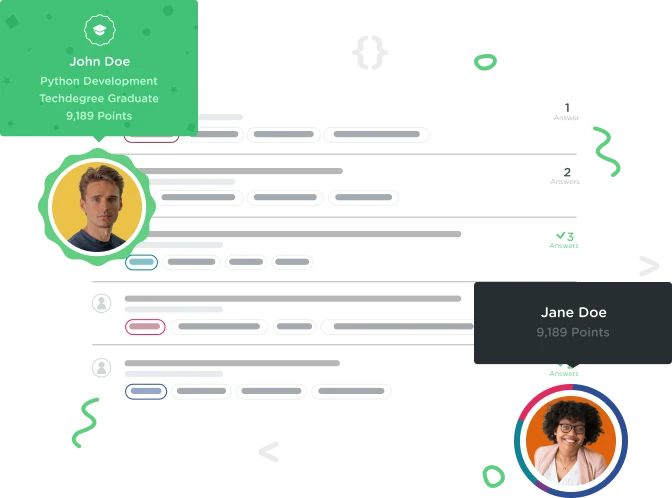
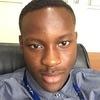
Osaro Igbinovia
Full Stack JavaScript Techdegree Student 15,928 PointsCan I get a solution to this challenge?
Here's my code. Where did I go wrong?
const list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) { if (e.target.tagName == 'BUTTON') { if(e.target.className == 'highlight'){ let li = e.target.parentNode; let prev = li.previousElementSibling; let ul = li.parentNode; if(prev){ ul.insertBefore(li,prev); } prev = li + prev; } } });
const list = document.getElementsByTagName('ul')[0];
list.addEventListener('click', function(e) {
if (e.target.tagName == 'BUTTON') {
if(e.target.className == 'highlight'){
let li = e.target.parentNode;
let prev = li.previousElementSibling;
let ul = li.parentNode;
if(prev){
ul.insertBefore(li,prev);
}
prev = li + prev;
}
}
});
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>Things to Learn</p>
<ul>
<li><p>Element Selection</p><button class = 'highlight'>Highlight</button></li>
<li><p>Events</p><button class = 'highlight'>Highlight</button></li>
<li><p>Event Listening</p><button class = 'highlight'>Highlight</button></li>
<li><p>DOM Traversal</p><button class = 'highlight'>Highlight</button></li>
</ul>
</section>
<script src="app.js"></script>
</body>
</html>
2 Answers
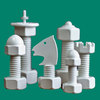
Steven Parker
229,783 PointsYou won't need to change the position of any elements for this challenge, and if you've already identified the sibling element you won't need to access anything further up the DOM tree in ancestry.
All you need here is to change (or add to) the class property of the sibling element.
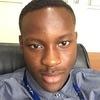
Osaro Igbinovia
Full Stack JavaScript Techdegree Student 15,928 PointsI've already passed the challenge and understand what you've been trying to say. Thank you :)
e.target.previousSibling.className = 'highlight';
Osaro Igbinovia
Full Stack JavaScript Techdegree Student 15,928 PointsOsaro Igbinovia
Full Stack JavaScript Techdegree Student 15,928 PointsI've been trying to solve this challenge for the past 12 hours with your tip but I'm really confused and I've tried everything I can.
Steven Parker
229,783 PointsSteven Parker
229,783 PointsHere's another couple of hints: a typical solution requires only one line and is an assignment statement. No conditionals ("if" statements) are necessary.
If you're still stuck after that, post the code you have now.