Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial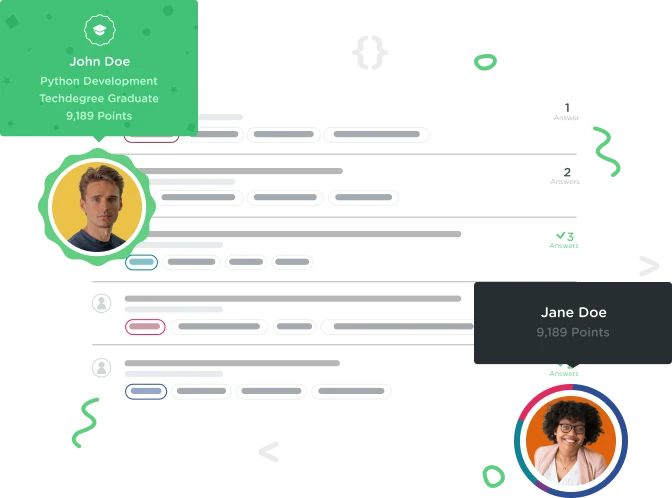
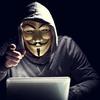
Pratham Mishra
Courses Plus Student 935 PointsCan I get help for this problem. I am not able to solve it. What is wrong in the code which I wrote in the program.
For this first task, create a new private method named normalizeDiscountCode. It should take the discount code that is passed into the method and return the uppercase version. Call it from the current applyDiscountCode method and set this.discountCode to the result.
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
private String normalizeDiscountCode(String discountCode) {
for(char l : discountCode.toCharArray()){
if(!Character.isLetter(l) && l != '$'){
throw new IllegalArgumentException("Invalid discount code");
}
}
// RETURN THE UPPER CASE
return discountCode.toUpperCase();
// CLOSE THE METHOD
}
public void applyDiscountCode(String discountCode) {
this.discountCode = discountCode;
}
}
2 Answers
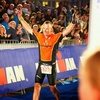
Steve Hunter
57,712 PointsHi there,
The method, normalizeDiscountCode
just needs to return the uppercase version of the String passed in. So that could look like:
private String normalizeDiscountCode(String code){
return code.toUpperCase();
}
Next, in the existing method called applyDiscountCode()
use the normaliseDiscountCode()
method before assigning the returned value into this.discountCode
. That looks like:
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
That should get you through the first task in the challenge.
Good luck!
Steve.
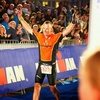
Steve Hunter
57,712 PointsSorry, are you on the second task here? Your question posed the text from Task 1, which is what I answered. The second task is to validate that the discount code contains only letters or the dollar symbol. That's what your code is trying to do.
You've done that correctly but have omitted the first task requirement to return the uppercase version of the code. Add the last line in my method below to yours and make sure you close the method's curly brace. That's missing. I've added some comments in your code.
private String normalizeDiscountCode(String code){
for(char c : code.toCharArray()){
if(!Character.isLetter(c) && c != '$'){
throw new IllegalArgumentException("Invalid discount code.");
}
}
return code.toUpperCase();
}
Steve.
rudyrbastari
5,212 Pointsrudyrbastari
5,212 Pointsthanks for the answer.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNo problem - I just added another as I'm confused about which task you are on!
