Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial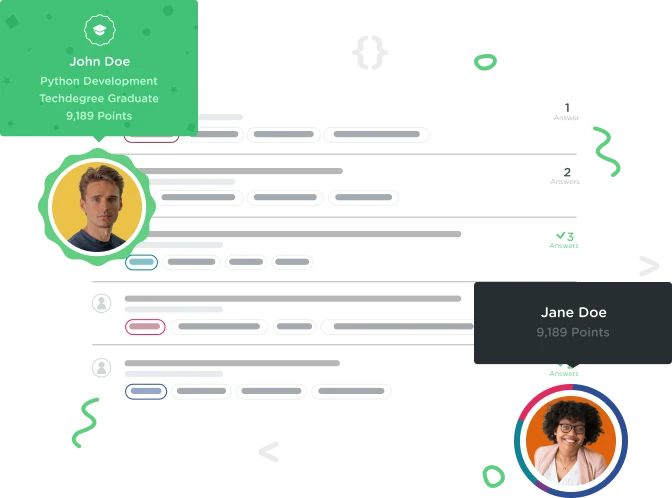

Charles Magee
Python Development Techdegree Student 657 PointsCan I get some guidance here
def suggest(product_idea):
return product_idea + "inator"
understand = input("What's your Product idea")
if proudct_idea <= 3:
raise ValueError ("Sorry your product idea is too short"
3 Answers

Mei Lee
3,339 PointsHere's a few hints:
You don't need the input(). When the suggest function is called, we pass along the product idea, which is used inside the function.
You could use the len() function to find the length of the String that is stored in the product_idea.
You could put the return statement last
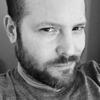
rayorke
27,709 PointsHi Charles!
I'll try to provide a bit of direction for this without giving it away. When Python processes a function, it handles things in sequence:
def function_name(argument):
if condition is true then:
this
else:
do this
I've broken down the process line by line.
- We are passing the argument of product_idea to the function - therefore, we don't need to worry about getting input from the user.
- We test the argument. In this case, we will want to use the len function, as Mei Lee suggested to find the length of the string product_idea, and see if it is less than 3 (not less than or equal to).
- If the argument is true (product_idea is less than 3), then we raise a ValueError - we can pass a string back to the user to let them know what the error is. This is returned to the user and the function stops.
- Here's where we use else - if the argument is false, then product_idea is equal to, or greater than 3, so we can return the product_idea + "inator"
I hope that helps you understand the challenge a bit more clearly. You'll be writing a lot of these in Python, so this will become easy in no time!

Tinevimbo Gororo
Courses Plus Student 42 Pointsdef suggest(product_idea):
if len(product_idea) < 3:
raise ValueError("Suggestion is too short")
return product_idea + "inator"