Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial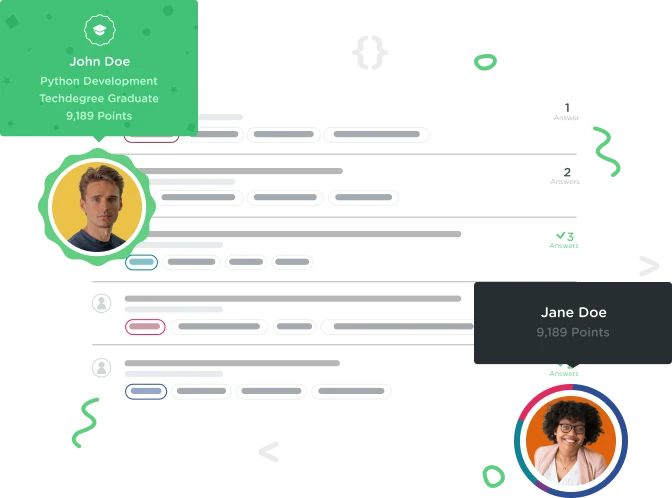

senay yakut
4,124 Pointscan i have in javascript as well? thanks
can you show how i can implement singly and doubly linked list in javascript?
4 Answers

Mike Straw
7,100 PointsOf course, sometimes the community can help. Here's the JavaScript I built:
// Node for a Singly Linked List
class Node {
/**
* Object to store single node of a linked list
* Contains data and link do next list
*/
data;
next_node;
constructor( data ) {
this.data = data;
}
}
// Node for a Doubly Linked List
class LinkedNode {
data;
next_node;
previous_node;
constructor( data ) {
this.data = data;
}
}
/** Linked List
* This works with both types of lists. Create a Node or LinkedNode as appropriate
*/
class LinkedList {
head;
is_empty() {
return ( this.head === undefined );
}
size() {
let current = this.head,
count = 0;
while ( current ) {
count++;
current = current.next_node;
}
return count;
}
}

jl64
Full Stack JavaScript Techdegree Graduate 19,359 PointsGreatly appreciate you sharing this!

hunter bougis
Full Stack JavaScript Techdegree Graduate 18,367 PointsIt was noted at the top of these links to contact you guys for the copy of this lesson in another language we’re looking for. In my situation...Javascript. I did that, but they said that email address was no longer in service. So, they advised to post it here in the community, which seems odd since someone previously asked the same question here and they directed them back to that email address??....Would really appreciate the JS version of the links below......Many thanks
Singly and Doubly:
Or…
This one, which covers singly, doubly with adding node, inserting nodes, search nodes, and delete nodes
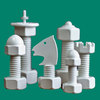
Steven Parker
230,274 PointsIf you find a bad link or email address on any pages you can report them as a bug to the Support staff. They're perhaps the folks who can help with your original issue too.

Matthew McQuain
14,184 PointsAs of 4.29.20, I got the exact same message from the Treehouse staff when I inquired about an earlier video in this same data structures series. I also requested something in JavaScript and was told to post here for help.
I'm genuinely surprised that there is very little content on Treehouse at all for Data Structures and Algorithms (in JavaScript). Not just here though, on YouTube as well. Trying to practice for whiteboard interviews in JavaScript is such a pain in the butt...
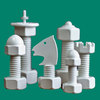
Steven Parker
230,274 PointsYou may have missed the note at the top of the page:
Note: If code is not provided below in the language you are looking for, send an email to content+cs@teamtreehouse.com

Stacy Davis
5,410 Pointshttps://www.geeksforgeeks.org/implementation-linkedlist-javascript/ this was the best JavaScript implementation I've found so far. It's nice to take the code from there and bring it to JSFiddle/etc and play with it.
jl64
Full Stack JavaScript Techdegree Graduate 19,359 Pointsjl64
Full Stack JavaScript Techdegree Graduate 19,359 PointsThis course, and comment section, have been out for years, and yet there is still no JavaScript for this lesson. This is literally at the end of the JS Full Stack Tech Degree, and while the theoretical aspects of data structures are nice to learn in general, it would be ideal for students taking tracks other than Python to see this code implemented in the language they chose to study, and are paying to learn about. Looking at the comments here, it seems like students are being sent back and forth, trying to properly report this issue. This should have been handled a long time ago.